Preventing Memory Leaks with Java ThreadLocal Best Practices
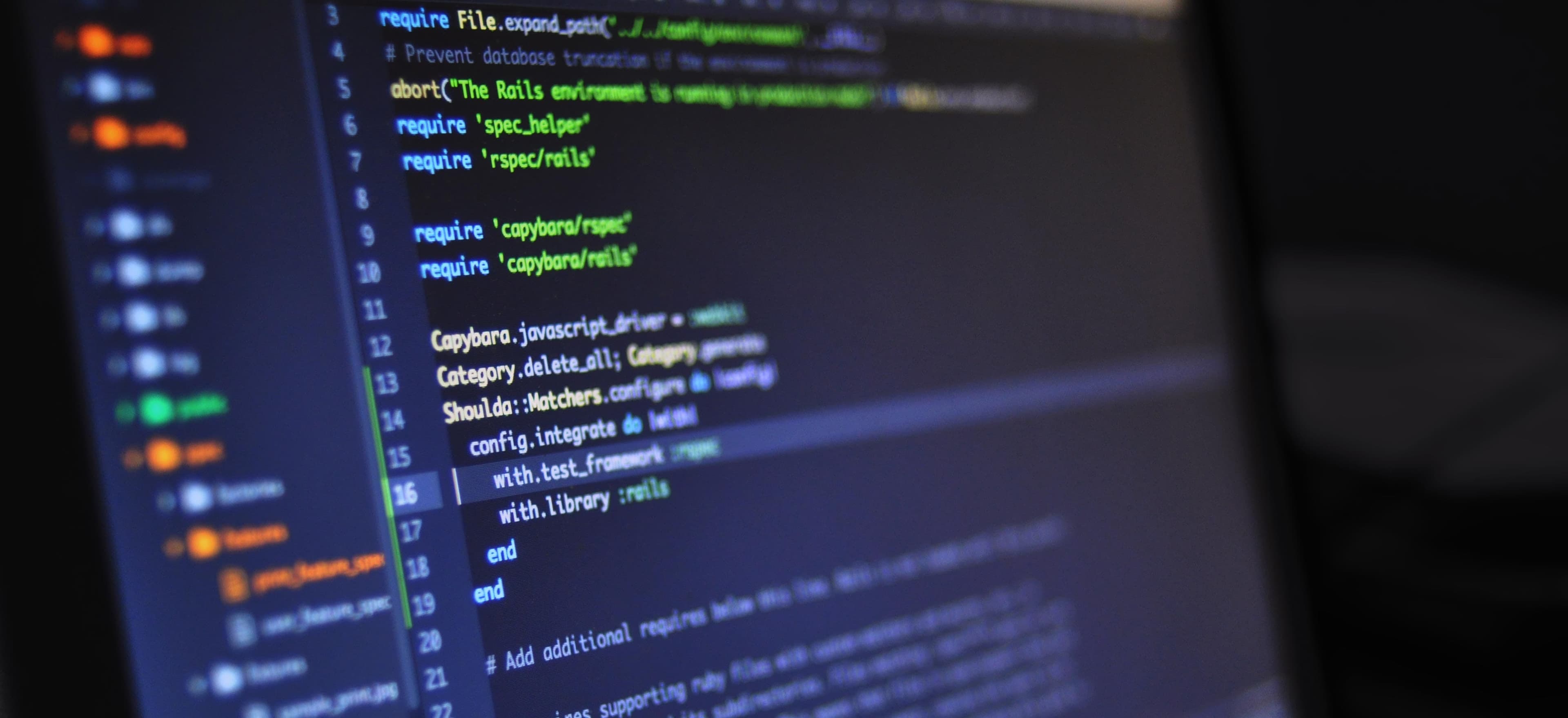
- Published on
Preventing Memory Leaks with Java ThreadLocal: Best Practices
Java’s memory management system is robust, yet developers often face challenges related to memory leaks. One commonly overlooked source of memory leaks in Java applications is the use of ThreadLocal
. In this blog post, we will explore the nuances of ThreadLocal
, its advantages, potential pitfalls, and best practices to prevent memory leaks.
What is ThreadLocal?
ThreadLocal
is a powerful utility provided by Java, enabling developers to maintain variables that are local to a specific thread. Each thread accessing a ThreadLocal
variable has its own independent copy, effectively isolating threads from each other. This can be particularly beneficial for situations like managing user sessions, database connections, or even performance optimization in concurrent applications.
Here’s a simple example of how ThreadLocal
works:
public class ThreadLocalExample {
private static ThreadLocal<Integer> threadLocalValue = ThreadLocal.withInitial(() -> 0);
public static void main(String[] args) {
// Simulating multiple threads
Runnable task = () -> {
Integer currentValue = threadLocalValue.get();
threadLocalValue.set(currentValue + 1);
System.out.println("Thread Value: " + threadLocalValue.get());
};
Thread thread1 = new Thread(task);
Thread thread2 = new Thread(task);
thread1.start();
thread2.start();
}
}
Code Explained
ThreadLocal<Integer> threadLocalValue = ThreadLocal.withInitial(() -> 0);
– Here we define aThreadLocal
variable that initializes to zero for each thread.threadLocalValue.get();
andthreadLocalValue.set(currentValue + 1);
– We access and modify the thread-local state, which is independent for each thread.
This setup demonstrates the convenience ThreadLocal
provides in maintaining state unique to each thread. However, improper use can lead to memory leaks.
Memory Leak Risks with ThreadLocal
While ThreadLocal
can be incredibly useful, it's essential to understand that it may inadvertently maintain strong references to objects held in its context. If a ThreadLocal
variable is not cleared, it can lead to memory leaks due to retaining references to otherwise unreferenced objects after a thread concludes its work.
How Does This Happen?
When a thread finishes its execution, its ThreadLocal
variables can persist in memory due to the innate behavior of the ThreadLocal
implementation. The internal structure may reference the ThreadLocal
and any object stored, therefore preventing these from being garbage-collected.
For example, consider the following case:
class MemoryLeakExample {
private static ThreadLocal<Object> threadLocalObject = new ThreadLocal<>();
public void process() {
Object localObject = new Object();
threadLocalObject.set(localObject);
// Perform operations with localObject
}
}
If process()
is called repeatedly within a thread and never clears the ThreadLocal
, then the localObject
continues to occupy memory, resulting in a memory leak.
Best Practices to Prevent Memory Leaks
-
Always Remove ThreadLocal Variables: After you are done using a
ThreadLocal
variable, callremove()
to clear any references held by theThreadLocal
. This is crucial in environments like servlet containers, where threads are pooled and reused:threadLocalObject.remove();
-
Initialize Wisely: Use
ThreadLocal.withInitial()
to avoid unintentional null references. Creating an initial value guarantees that a thread always has a valid state. -
Minimize Scope: Limit the lifespan and scope of the
ThreadLocal
variable. Create a new instance ofThreadLocal
for truly isolated use to avoid global state leaks. -
Utilize Weak References: Consider using
WeakReference
if applicable. Although Java'sThreadLocal
does not inherently utilize weak references, implementing a customThreadLocal
can help mitigate potential leaks. -
Document
ThreadLocal
Usage: Ensure code comments and documentation clearly indicate where and howThreadLocal
variables should be managed. Coordination among team members enhances code maintainability. -
Monitor Memory Usage: Regularly profile your application’s memory usage. Use tools like VisualVM or Java Mission Control to detect leaks and analyze memory allocation.
-
Use Libraries with Caution: Be aware of third-party libraries that utilize
ThreadLocal
. Validate their usage and ensure they are cleaning up properly.
Closing Remarks
Leveraging Java’s ThreadLocal
can significantly enhance the efficiency and maintainability of multi-threaded code. Conversely, if not managed correctly, it can lead to severe memory management issues.
By following best practices, such as clearing variables and managing their scope judiciously, developers can prevent memory leaks and ensure robust application performance.
For further reading, check out the Java Documentation on ThreadLocal or dive deeper into Java Memory Management.
By understanding the ins and outs of ThreadLocal
, you can write cleaner, more efficient Java code while effectively managing your application’s memory. Happy coding!
Checkout our other articles