Mastering Reentrant Locks to Eliminate Deadlock Issues
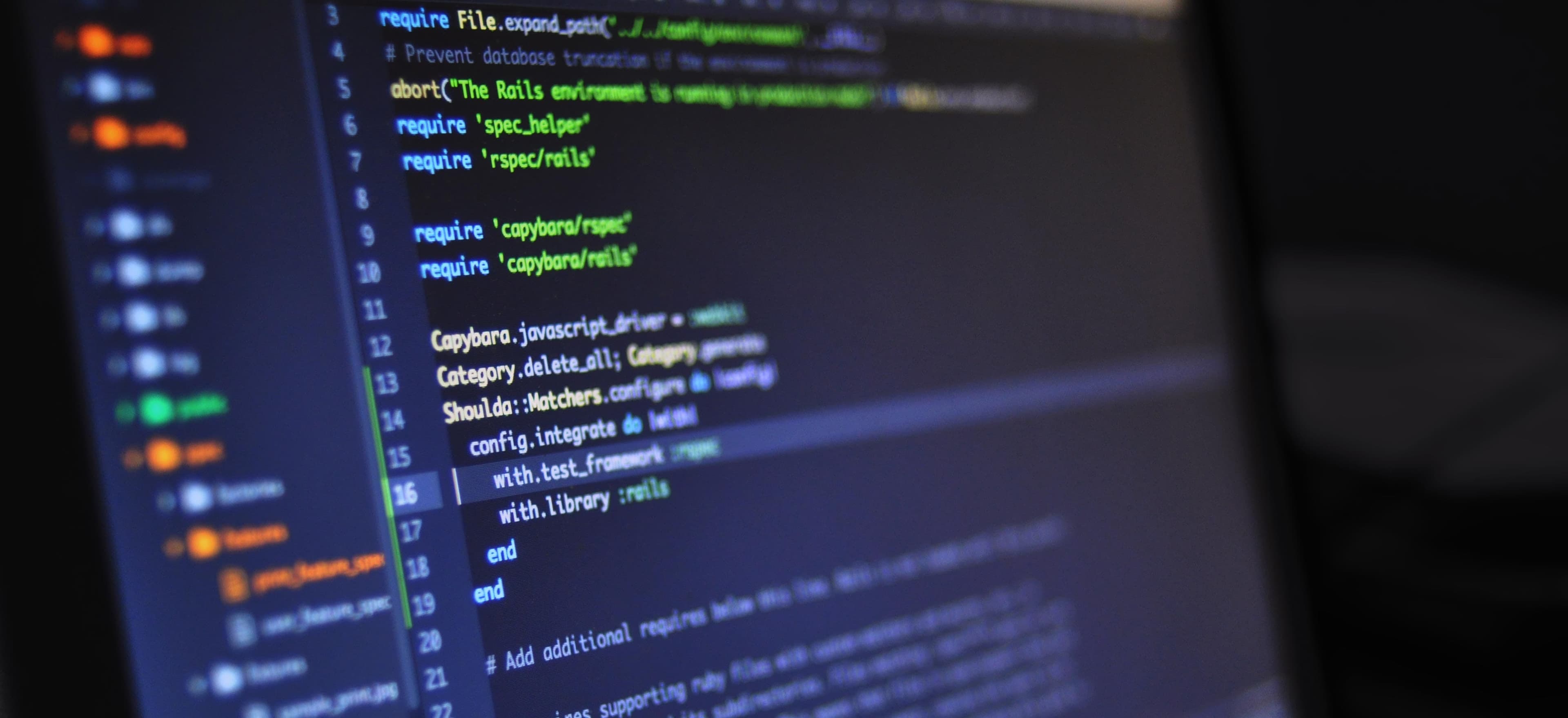
- Published on
Mastering Reentrant Locks to Eliminate Deadlock Issues
In the world of concurrent programming, one of the most critical concerns is ensuring that threads can safely interact with shared resources. A fundamental concept in managing threads is locking mechanisms. Among various locking techniques, Reentrant Locks have emerged as a robust solution to prevent deadlock scenarios. This blog post dives deep into understanding reentrant locks, their advantages, and how to use them effectively in Java.
What are Reentrant Locks?
A Reentrant Lock is an advanced locking mechanism found in the Java java.util.concurrent.locks
package. Unlike traditional synchronized blocks, which are tied to the intrinsic locking of an object, reentrant locks allow a thread that already owns the lock to re-enter the same lock without causing a blockage. This is especially useful when there are multiple synchronized methods trying to gain access to the same resource.
Key Features of Reentrant Locks:
- Fairness: Reentrant locks can be configured to provide a fair access policy.
- Check Lock State: You can query whether the lock is held.
- Interruptible Locking: The wait for acquiring a lock can be interrupted.
- Support for Multiple Condition Variables: You can create multiple waiting states with different conditions.
Why Use Reentrant Locks?
To understand the utility of reentrant locks, consider the following scenarios:
-
Avoiding Deadlocks: When multiple threads need to access shared resources, using reentrant locks can help avoid deadlock situations by allowing a thread to re-enter a lock it already holds.
-
Improved Concurrency: They allow for more granular control over locking and unlocking resources, which can optimize performance in multithreading applications.
-
Better Code Readability: Reentrant locks can make your code cleaner and easier to understand than nested synchronized blocks.
A Simple Example
Let's illustrate the use of reentrant locks with a practical code example. Consider a banking system where two accounts can transfer money between each other.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class BankAccount {
private int balance;
private final Lock lock = new ReentrantLock(); // Using Reentrant Lock
public BankAccount(int initialBalance) {
this.balance = initialBalance;
}
public void deposit(int amount) {
lock.lock(); // Acquire the lock
try {
balance += amount;
System.out.println("Deposited " + amount + ", new balance: " + balance);
} finally {
lock.unlock(); // Ensure the lock is released
}
}
public void transfer(BankAccount target, int amount) {
if (this == target) {
// Prevents deadlock by avoiding self-locking
deposit(amount);
return;
}
// Locking both to prevent deadlock
boolean myLock = false;
boolean targetLock = false;
try {
myLock = lock.tryLock();
targetLock = target.lock.tryLock();
if (myLock && targetLock) {
this.balance -= amount;
target.deposit(amount);
System.out.println("Transferred " + amount + " to account, new balance: " + balance);
} else {
if (myLock) lock.unlock();
if (targetLock) target.lock.unlock();
System.out.println("Transfer failed due to lock not acquired!");
}
} finally {
if (myLock) lock.unlock();
if (targetLock) target.lock.unlock();
}
}
}
Commentary on the Code
- Lock Initialization: We create a
ReentrantLock
instance for eachBankAccount
. - Lock Management: Using
lock.lock()
andlock.unlock()
ensures that only one thread can execute critical sections. - Avoiding Deadlocks: In the
transfer
method, we check if the current account is the target account. If so, we simply deposit the amount, avoiding the deadlock condition that could arise if we held the same lock twice.
Handling Fairness
Reentrant locks can be configured to provide fair access, which ensures that threads acquire the lock in the order they requested it. If you prioritize fairness in concurrent applications, you can create a fair reentrant lock as shown below:
Lock fairLock = new ReentrantLock(true); // 'true' for fairness
When you set the fairness flag to true
, threads will acquire the lock in FIFO order. While fairness may reduce throughput in high-concurrency situations, it can be beneficial if task completion order is critical.
The Last Word
Reentrant locks are powerful tools for managing concurrency in Java. Their ability to allow a thread to re-acquire a lock and their flexibility with fairness make them suitable for many scenarios where traditional synchronized blocks might lead to deadlocks or inefficient execution.
Further Reading
To enhance your understanding of Java concurrency, explore these resources:
By mastering reentrant locks and understanding their nuances, you can create more reliable and efficient Java applications capable of handling multiple threads safely. Happy coding!
Checkout our other articles