Mastering Java Garbage Collection: Common Tuning Mistakes
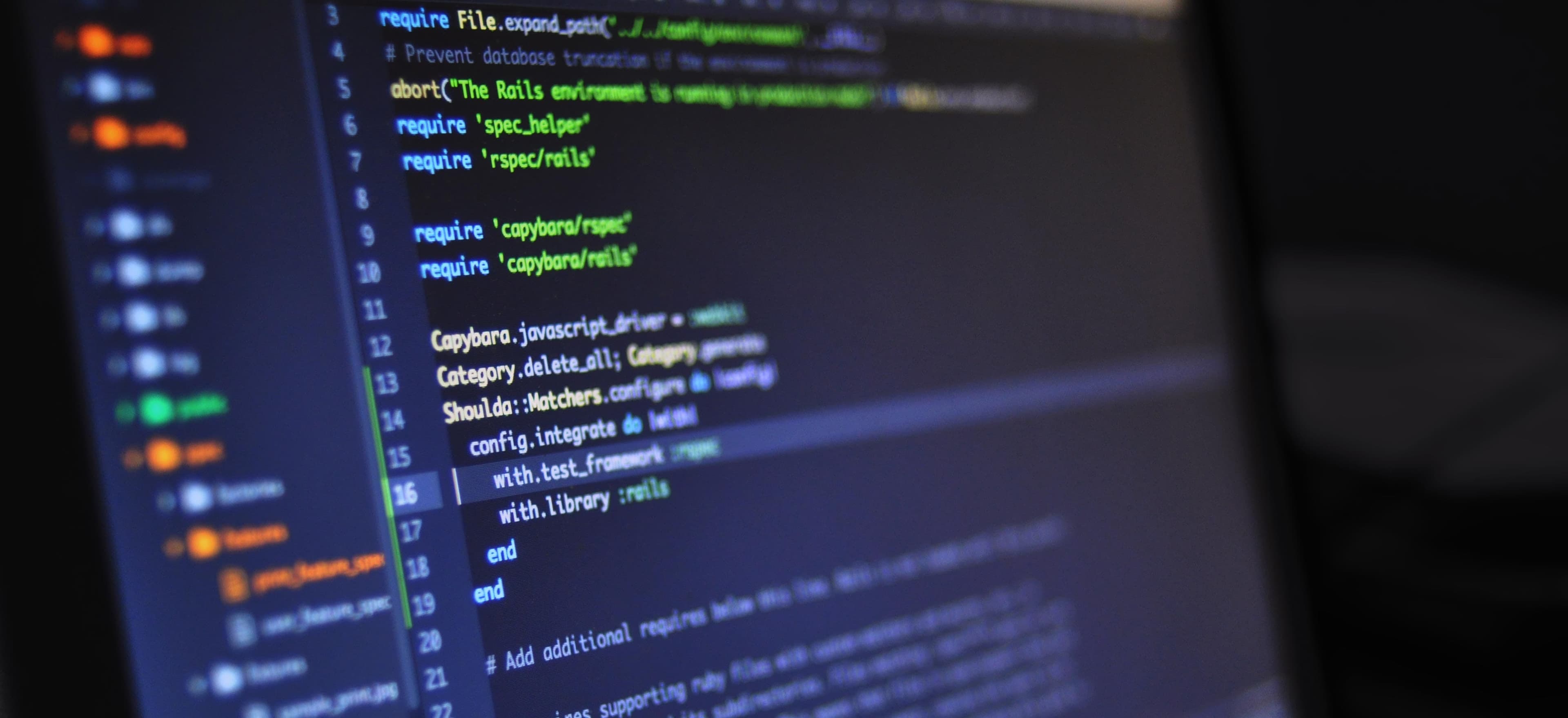
- Published on
Mastering Java Garbage Collection: Common Tuning Mistakes
Java has long been a go-to language for developers around the world. One of its key features is automatic memory management through Garbage Collection (GC). Although this automates a critical aspect of memory management, tuning the garbage collector improperly can lead to performance degradation. In this blog post, we will explore common tuning mistakes in Java garbage collection and how to avoid them.
Understanding Garbage Collection
Garbage collection in Java is the process by which the Java Virtual Machine (JVM) automatically identifies and reclaims memory that is no longer in use. Despite the complexity behind the scenes, for developers, the operation largely remains invisible. However, understanding some basics is crucial for effective tuning.
Types of Garbage Collectors
The JVM provides several garbage collectors, each designed to cater to different application requirements:
- Serial Garbage Collector: Best for small applications. It uses a single thread for garbage collection.
- Parallel Garbage Collector: Utilizes multiple threads for garbage collection, enhancing performance on multi-core machines.
- Concurrent Mark-Sweep (CMS): Aimed at minimizing pause times.
- G1 Garbage Collector: Designed for applications that require predictable response times.
For a deeper dive into different types of garbage collectors, check out Oracle's official documentation.
Common Tuning Mistakes in Java Garbage Collection
1. Over-Tuning the JVM Parameters
Mistake: Changing too many JVM flags without recognizable patterns.
Why it Matters: Java's garbage collectors are complex systems that interact with the application. Introducing multiple flag changes can lead to unpredictable behavior.
Solution: Start by understanding the impact of each parameter individually before attempting combinations. For example, you might want to adjust the heap size:
java -Xms512m -Xmx2048m -XX:+UseG1GC -jar YourApplication.jar
Here, -Xms
sets the initial heap size, while -Xmx
sets the maximum heap size. Adjusting these correctly can optimize garbage collection performance significantly.
2. Ignoring Monitoring Tools
Mistake: Neglecting tools that provide insights into memory performance.
Why it Matters: Without proper monitoring, developers often end up making blind guesses about what to tune. This could lead to ineffective changes.
Solution: Utilize monitoring tools such as Java Mission Control or VisualVM. These allow you to visualize memory consumption and garbage collection metrics, providing valuable insights.
jcmd <pid> GC.heap_dump
The above command can capture a heap dump for analysis. This dump can be visualized with tools like Eclipse MAT (Memory Analyzer Tool).
3. Setting Small Heap Sizes
Mistake: Using a small heap size to minimize memory usage.
Why it Matters: While this may help in controlling memory consumption, it can also lead to frequent garbage collections, thus impacting performance.
Solution: Perform load testing to gauge the ideal heap size for your application. Start with a reasonable range and increase based on performance metrics gathered during peak loads and times.
4. Ignoring Object Lifetimes
Mistake: Failing to understand the lifetimes of objects in your application.
Why it Matters: Tuning garbage collection settings without considering the lifecycle of objects can result in excessive object churn or retention.
Solution: Analyze your application to determine which objects are short-lived and which are long-lived.
List<HeavyObject> heavyList = new ArrayList<>();
for (int i = 0; i < 100; i++) {
heavyList.add(new HeavyObject());
}
// heavyList is a short-lived object
In the example above, the heavyList
objects can be reclaimed quickly after use. Hence, players should avoid tuning parameters that maximize the retention of short-term objects.
5. Changing GC Algorithms Without Context
Mistake: Switching garbage collection algorithms without any performance measurements or context.
Why it Matters: Each application has its distinct workload characteristics. Just because G1 is suited for one application doesn’t mean it will work for yours.
Solution: Analyze your performance metrics before and after changing GC algorithms. Run benchmarks to understand the implications.
java -Xmx2g -XX:+UseG1GC -jar YourApplication.jar
Monitor the performance and garbage collection metrics before you settle on a new configuration.
6. Not Considering the Impact on CPU
Mistake: Overlooking the effect GC can have on CPU usage.
Why it Matters: Some GC algorithms consume more CPU resources than others. This may lead to performance bottlenecks.
Solution: Profile your application and monitor CPU utilization during garbage collection phases. Tools like YourKit Profiler and JProfiler can help you analyze GC behavior and its CPU usage.
Best Practices for Effective GC Tuning
1. Establish Baselines
Before making any tuning decisions, establish a performance baseline. This will help you measure the impact of your changes accurately.
2. Incremental Changes
Make changes one at a time and monitor their impact. This approach allows you to identify which changes yield the most benefit.
3. Regular Review
Garbage collection and object allocation patterns may change over time as your application evolves. Review your GC settings regularly, especially after significant code changes.
4. Utilize the Right Collector
Choose the garbage collector that best fits your application type. High-throughput applications will benefit from a different collector than those requiring low-latency responses.
To Wrap Things Up
Mastering Java garbage collection is not merely about changing configurations but understanding your application's memory requirements. By avoiding common tuning mistakes and applying best practices, you can significantly enhance your application's performance and responsiveness.
For further reading and to deepen your understanding of Java memory management, explore the comprehensive guide on Java Memory Management by Baeldung. Your journey to mastering garbage collection starts now. Happy tuning!