Common Pitfalls in Java Float and Double Comparisons
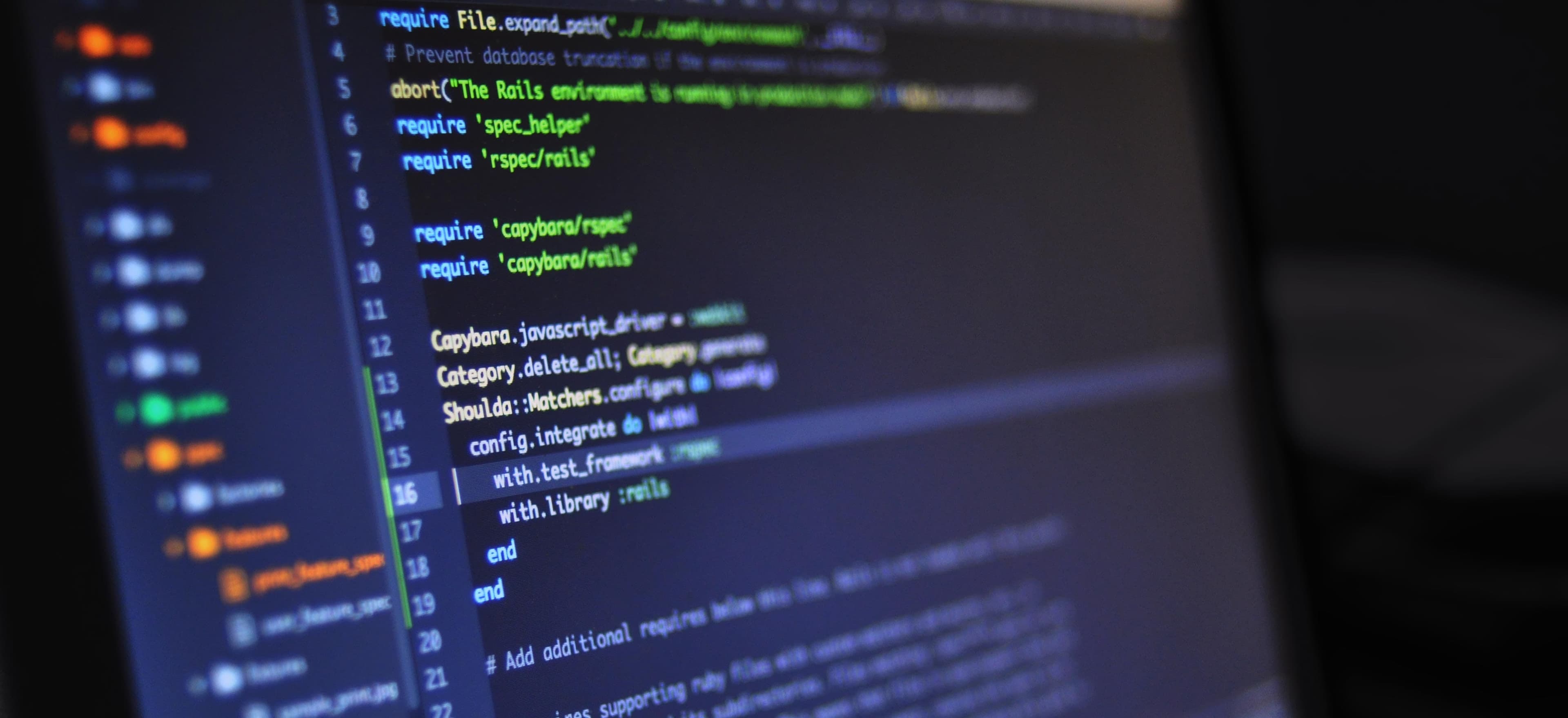
- Published on
Common Pitfalls in Java Float and Double Comparisons
Java is a powerful programming language widely utilized in various applications, including Android development, web services, and enterprise-level solutions. One of the more nuanced areas of Java that can trip up even seasoned developers is the comparison of floating-point numbers, specifically float
and double
. In this blog post, we will delve into common pitfalls encountered when comparing these types of numbers, discuss the reasons behind these challenges, and provide practical solutions.
Understanding Floating-Point Representation
Before we embark on the common pitfalls, it's crucial to understand how floating-point numbers are represented in Java. Both float
and double
represent numbers in binary format, which can lead to precision loss. Here's a brief overview:
- Float: A single-precision 32-bit IEEE 754 floating-point. Suitable for saving memory in large arrays of floating-point numbers.
- Double: A double-precision 64-bit IEEE 754 floating-point. Offers greater precision and is the default type for decimal values in Java.
Example of Floating-Point Representation
Here's a simple code snippet that illustrates how floating-point numbers are stored in Java:
public class FloatingPointRepresentation {
public static void main(String[] args) {
float floatVal = 0.1f; // Float representation
double doubleVal = 0.1; // Double representation
System.out.println("Float value: " + floatVal);
System.out.println("Double value: " + doubleVal);
}
}
In this example, the decimal 0.1 cannot be represented exactly in binary form for both types, leading to minor precision differences.
Common Pitfalls in Comparisons
1. Direct Comparison of Float and Double
One of the most notorious pitfalls arises when developers compare two floating-point numbers directly, such as using ==
or !=
. Due to precision issues, this can often yield unexpected results.
Example:
public class FloatDoubleComparison {
public static void main(String[] args) {
float floatVal = 0.1f;
double doubleVal = 0.1;
if (floatVal == doubleVal) {
System.out.println("Equal!");
} else {
System.out.println("Not equal!");
}
}
}
What Happens?
The output will likely be "Not equal!" because floatVal
is a float
, and doubleVal
is a double
. The precision loss stemming from the single-precision representation means floatVal
loses the exact representation of the decimal, leading to this unexpected comparison outcome.
2. Adding Small Numbers
Another common issue arises when small numbers are added to a floating-point variable. You might expect the result to exactly match a known value, but floating-point arithmetic can yield surprising results.
Example:
public class SmallNumberAddition {
public static void main(String[] args) {
float floatResult = 0.1f + 0.2f;
System.out.println("Float result: " + floatResult);
if (floatResult == 0.3f) {
System.out.println("Float result is equal to 0.3f");
} else {
System.out.println("Float result is NOT equal to 0.3f");
}
}
}
What Happens?
This code will likely produce "Float result is NOT equal to 0.3f" because the operation does not yield an exact amount. Although 0.1 and 0.2 seem simple enough, the binary representation of these numbers means some precision is lost.
3. Rounding Errors
Precision loss can propagate through calculations and lead to significant errors, especially in scientific computations or financial applications where precision is paramount.
Example:
public class RoundingError {
public static void main(String[] args) {
double result = 0.1 + 0.2 - 0.3;
if (result == 0.0) {
System.out.println("Result is zero!");
} else {
System.out.println("Result is NOT zero! Value: " + result);
}
}
}
What Happens?
You'll likely see "Result is NOT zero!" as output due to minor rounding errors throughout the calculations. This is a clear indication of accumulating errors when using floating-point numbers.
How to Safely Compare Floats and Doubles
1. Use an Epsilon Value
One standard approach to safely compare float
and double
values is to define a small tolerance level, often referred to as epsilon. This allows for some acceptable deviation when comparing two floating-point numbers.
Example:
public class SafeComparison {
public static void main(String[] args) {
float floatVal = 0.1f + 0.2f;
double expectedValue = 0.3;
double epsilon = 0.00001;
if (Math.abs(floatVal - expectedValue) < epsilon) {
System.out.println("Float value is approximately equal to expected value.");
} else {
System.out.println("Float value is NOT approximately equal to expected value.");
}
}
}
2. BigDecimal for Precision
For applications requiring high precision, such as financial applications, it's often recommended to use BigDecimal
. Here's a quick example:
import java.math.BigDecimal;
public class BigDecimalComparison {
public static void main(String[] args) {
BigDecimal bd1 = new BigDecimal("0.1");
BigDecimal bd2 = new BigDecimal("0.2");
BigDecimal bdTotal = bd1.add(bd2);
if (bdTotal.compareTo(new BigDecimal("0.3")) == 0) {
System.out.println("BigDecimals are equal!");
} else {
System.out.println("BigDecimals are NOT equal!");
}
}
}
Using BigDecimal
, you can avoid the precision issues inherent to float
and double
types altogether.
3. Awareness and Thorough Testing
Lastly, always be aware of the limitations associated with float
and double
. It's essential to test different scenarios where comparisons may fail. Developing a thorough test suite can help catch these pitfalls before they surface in production.
Final Thoughts
While float
and double
types in Java provide powerful capabilities for working with decimal numbers, they also come with inherent risks. By understanding the common pitfalls, adopting safe comparison strategies, and leveraging tools like BigDecimal
, you can conduct your operations smoothly and accurately.
For additional context on floating-point precision, consider exploring the IEEE 754 standard for floating-point arithmetic. This resource will provide further insights into how floating-point numbers behave in various programming languages, including Java.
If you're interested in deeper insights into Java's numerical types, Java's official documentation is an invaluable resource that can guide you through the intricacies of data handling in Java.
Thank you for reading! Explore your floating-point comparisons carefully, and stay tuned for more insights into effective Java programming.
Checkout our other articles