Preventing StackOverflowError in Java: Tips and Tricks
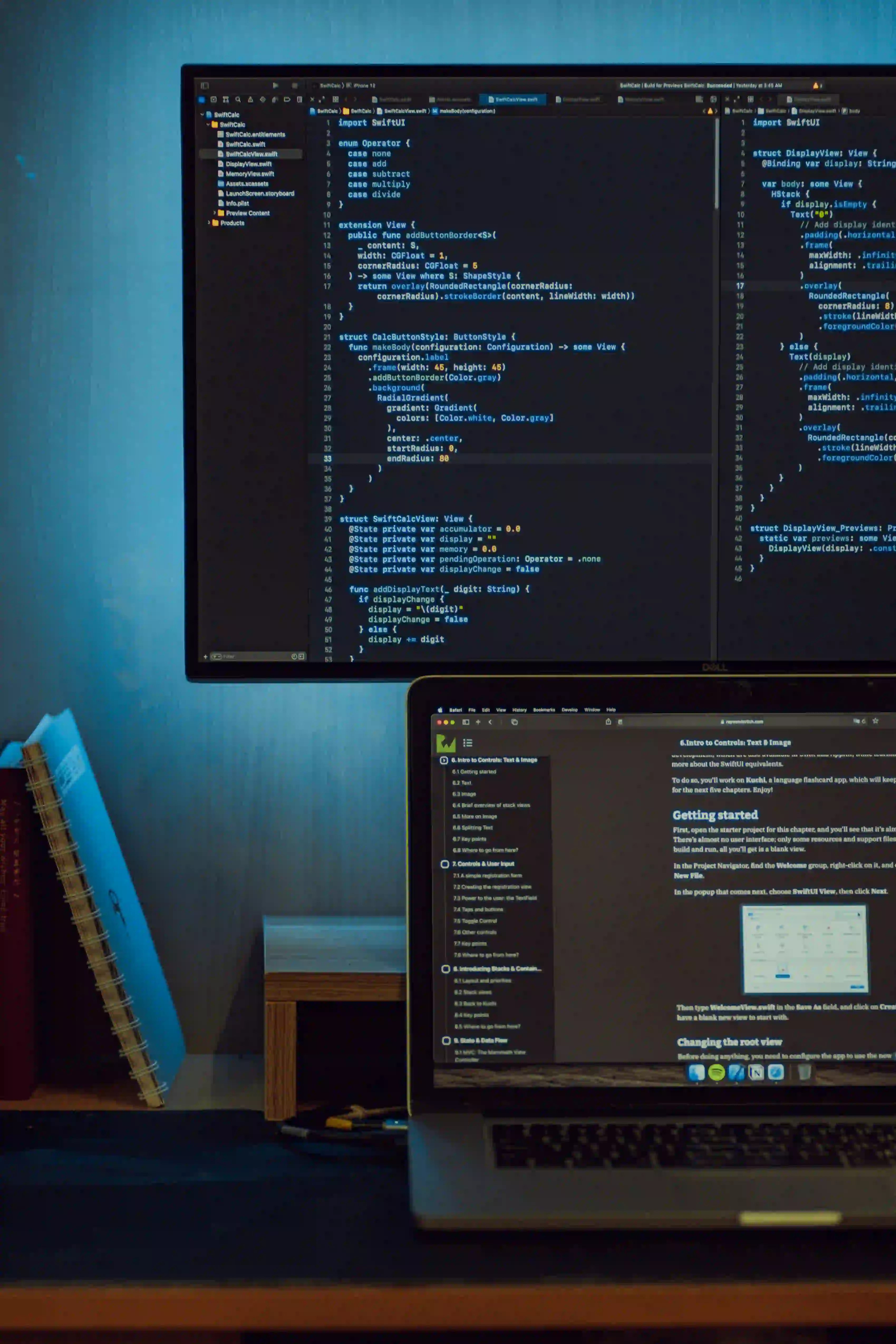
Preventing StackOverflowError in Java: Tips and Tricks
The StackOverflowError
in Java is a runtime error that occurs when a program recurses too deeply and the Java Virtual Machine (JVM) runs out of stack space. This can happen in scenarios involving poorly defined recursive functions or excessive nested method calls. Understanding and preventing this error is crucial for developers who want to create robust applications. In this blog post, we will explore tips and tricks to prevent StackOverflowError
, including code examples and best practices.
Understanding StackOverflowError
Before we dive into prevention strategies, it's essential to understand what happens when a StackOverflowError
occurs. In Java, each thread has its own stack, which stores local variables, method calls, and other data. Every time a method is invoked, a new frame is pushed onto the stack. If the recursion is too deep or too many method calls occur, the allotted stack space is exhausted, leading to a StackOverflowError
.
Here’s a simple code example that demonstrates a StackOverflowError
:
public class RecursiveExample {
public static void recursiveMethod(int value) {
// Recursive call without termination condition
recursiveMethod(value + 1);
}
public static void main(String[] args) {
recursiveMethod(1);
}
}
In this code snippet, recursiveMethod
calls itself indefinitely without a termination condition, ultimately resulting in a StackOverflowError
.
Tips for Preventing StackOverflowError
1. Define Base Cases
One of the most effective ways to prevent a StackOverflowError
is to ensure that recursive methods have well-defined base cases. A base case provides a condition under which the recursion will stop.
Example: Factorial Calculation
public class FactorialExample {
public static int factorial(int n) {
// Base case
if (n == 0 || n == 1) {
return 1;
}
return n * factorial(n - 1);
}
public static void main(String[] args) {
System.out.println("Factorial of 5: " + factorial(5)); // Outputs 120
}
}
In the example above, the base case is defined when n
is 0 or 1, preventing infinite recursion and potential StackOverflowError
.
2. Use Iteration Instead of Recursion
In certain cases, a recursive approach can be converted to an iterative one. Iteration uses looping constructs, thus bypassing the need for stack frames.
Example: Fibonacci Calculation Iteratively
public class FibonacciExample {
public static int fibonacci(int n) {
if (n <= 1) return n;
int a = 0, b = 1, sum = 0;
for (int i = 2; i <= n; i++) {
sum = a + b;
a = b;
b = sum;
}
return sum;
}
public static void main(String[] args) {
System.out.println("Fibonacci of 5: " + fibonacci(5)); // Outputs 5
}
}
In this snippet, we calculate Fibonacci numbers iteratively, which eliminates the risk of StackOverflowError
.
3. Tail Recursion Optimization
Java does not perform tail call optimization, which is a technique where recursive calls that are in tail position can be converted to iterations. However, it can be useful to manually implement this concept when designing recursive methods.
Example: Tail Recursion with an Accumulator
public class TailRecursionExample {
public static int factorialTail(int n, int accumulator) {
// Base case
if (n == 0) return accumulator;
// Tail recursive call
return factorialTail(n - 1, n * accumulator);
}
public static void main(String[] args) {
System.out.println("Factorial of 5 using tail recursion: " + factorialTail(5, 1)); // Outputs 120
}
}
In this example, an accumulator is used to carry the result through recursive calls. This approach can help clarify how the state is maintained and can be altered to prevent stack overflow in languages that support tail call optimization.
4. Increase Stack Size
If you encounter a StackOverflowError
while running a Java application, sometimes the solution is to increase the stack size allocated to the JVM. This can be done using the -Xss
parameter.
For example, this command increases the stack size to 2 MB:
java -Xss2m YourClassName
However, be cautious when using this method. Rather than a permanent fix, it is better to optimize your code by ensuring proper termination in recursive calls.
5. Monitor Recursion Depth
In some complex algorithms, you might need to track the recursion depth during execution. This allows you to implement preventive measures before the recursion goes beyond a safe limit.
Example: Monitoring Recursion Depth
public class RecursionDepthExample {
private static final int MAX_DEPTH = 1000;
public static void recursiveMethod(int depth) {
if (depth > MAX_DEPTH) {
throw new StackOverflowError("Maximum recursion depth exceeded");
}
// Recursive call
recursiveMethod(depth + 1);
}
public static void main(String[] args) {
try {
recursiveMethod(1);
} catch (StackOverflowError e) {
System.err.println(e.getMessage());
}
}
}
In this example, we define a maximum recursion depth and throw an exception when it is exceeded, preventing a StackOverflowError
.
Final Thoughts
A StackOverflowError
can be a developer's worst nightmare, but understanding its underlying causes can significantly reduce the risk of encountering this issue in your code. By following the tips outlined in this article, such as defining base cases, opting for iteration when possible, leveraging tail recursion concepts, increasing stack size, and monitoring recursion depth, you can write more resilient Java applications.
For further reading on recursion and memory management in Java, check out the Oracle Java Documentation and the book Effective Java by Joshua Bloch.
By implementing these strategies, you'll not only prevent StackOverflowError
but also improve the overall clarity and efficiency of your code. Happy coding!