Understanding and Fixing ClassCastException in Generics
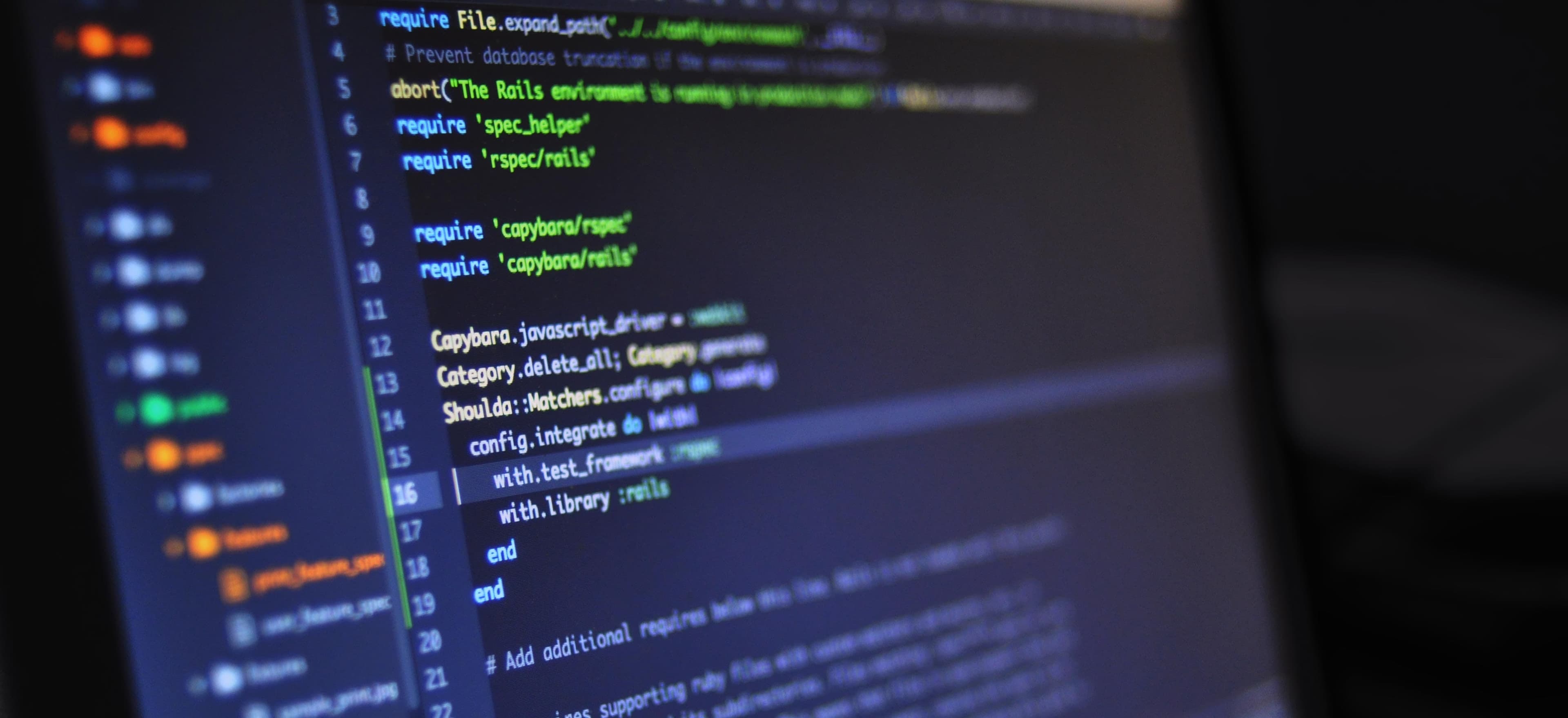
- Published on
Understanding and Fixing ClassCastException in Generics
Java is a statically typed language, allowing developers to specify the type of data objects. This can help in catching errors at compile time rather than runtime. However, there's a common pitfall related to generics that many Java developers face: ClassCastException
. This exception can occur when you attempt to cast an object to a class of which it is not an instance. In this blog post, we will dive deep into understanding the ClassCastException
, particularly in the context of generics, and how to fix it.
What is ClassCastException?
ClassCastException
is a runtime exception that is thrown when an object is attempted to be cast to a subclass or interface that it does not implement. For example, if you try to cast an instance of Apple
to Orange
, it would throw a ClassCastException
.
Fruit fruit = new Apple();
Orange orange = (Orange) fruit; // This line will throw ClassCastException
The Impact of Generics
Before Java 5, developers relied heavily on type casting, which contributed to many ClassCastException
instances at runtime. Generics were introduced to the Java language to bring stronger type-checking at compile time. However, improper use of generics may still lead to ClassCastException
.
Example Scenario
Suppose we have a generic class:
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
Now let’s see how it could lead to ClassCastException
if misused.
A Problematic Sample Code
public class Main {
public static void main(String[] args) {
Box<Fruit> fruitBox = new Box<>();
fruitBox.setItem(new Apple());
Box<Apple> appleBox = (Box<Apple>) (Box<?>) fruitBox; // Unsafe cast
Apple apple = appleBox.getItem(); // Works fine
}
}
In the code above, we had to cast the fruitBox
to Box<Apple>
, which is unsafe. If we changed our fruitBox
to contain a different type of Fruit:
fruitBox.setItem(new Banana()); // Now it contains a Banana instead of an Apple
When you call appleBox.getItem()
, you'll get a ClassCastException
because we are trying to treat the Banana
as an Apple
.
Best Practices to Avoid ClassCastException
1. Leverage Type Safety with Generics
Ensure that when you define your generics, you stick to the type you specified. Instead of casting manually, let the compiler help you with type-safety.
2. Avoid Raw Types
Using raw types defeats the purpose of generics. Always specify the type parameter to maintain type checks.
Example of Raw Type Usage:
Box rawBox = new Box(); // Raw type
rawBox.setItem("Hello"); // No compile-time error
Fruit fruit = (Fruit) rawBox.getItem(); // May cause ClassCastException at runtime
3. Use Wildcards When Necessary
If you need to handle objects of multiple types, consider using wildcards (?
).
Example of Wildcard Usage
public void printBoxItems(Box<? extends Fruit> box) {
Fruit fruit = box.getItem();
System.out.println(fruit);
}
This allows for a more flexible design without the risk of ClassCastException
while still ensuring type safety.
4. Employ Type Checking
If you need to check object types at runtime, use the instanceof
operator to provide safe type checks.
if (fruit instanceof Apple) {
Apple apple = (Apple) fruit;
// Safely operate with the apple object
}
5. Utilize Generics Enforcers
Consider using generics enforcers or utility libraries like Guava’s TypeToken
which aids in preserving the generic type at runtime.
Final Considerations
ClassCastException
can be a frustrating experience for Java developers, especially when working heavily with generics. Adhering to the best practices discussed will help mitigate the occurrence of this exception and enhance your code's robustness.
By leveraging type safety through generics, avoiding raw types, using wildcards properly, employing type-checking, and considering libraries that assist with generics, you can ensure a smoother programming experience. Remember, the aim is clear and maintainable code, which complies with types and their intended usages.
For further reading, you may find Oracle's Guide on Generics helpful, and consider exploring the Java Documentation for additional insights.
Recommended Next Steps
- Practice making generic classes and interfaces.
- Experiment with wildcards in collections and methods.
- Try integrating
TypeToken
from Guava in your projects for advanced generics handling.
By following these steps, not only will your understanding deepen, but you’ll also avoid common pitfalls like ClassCastException
. Happy coding!