Navigating Common JNI Edge Cases in Android Development
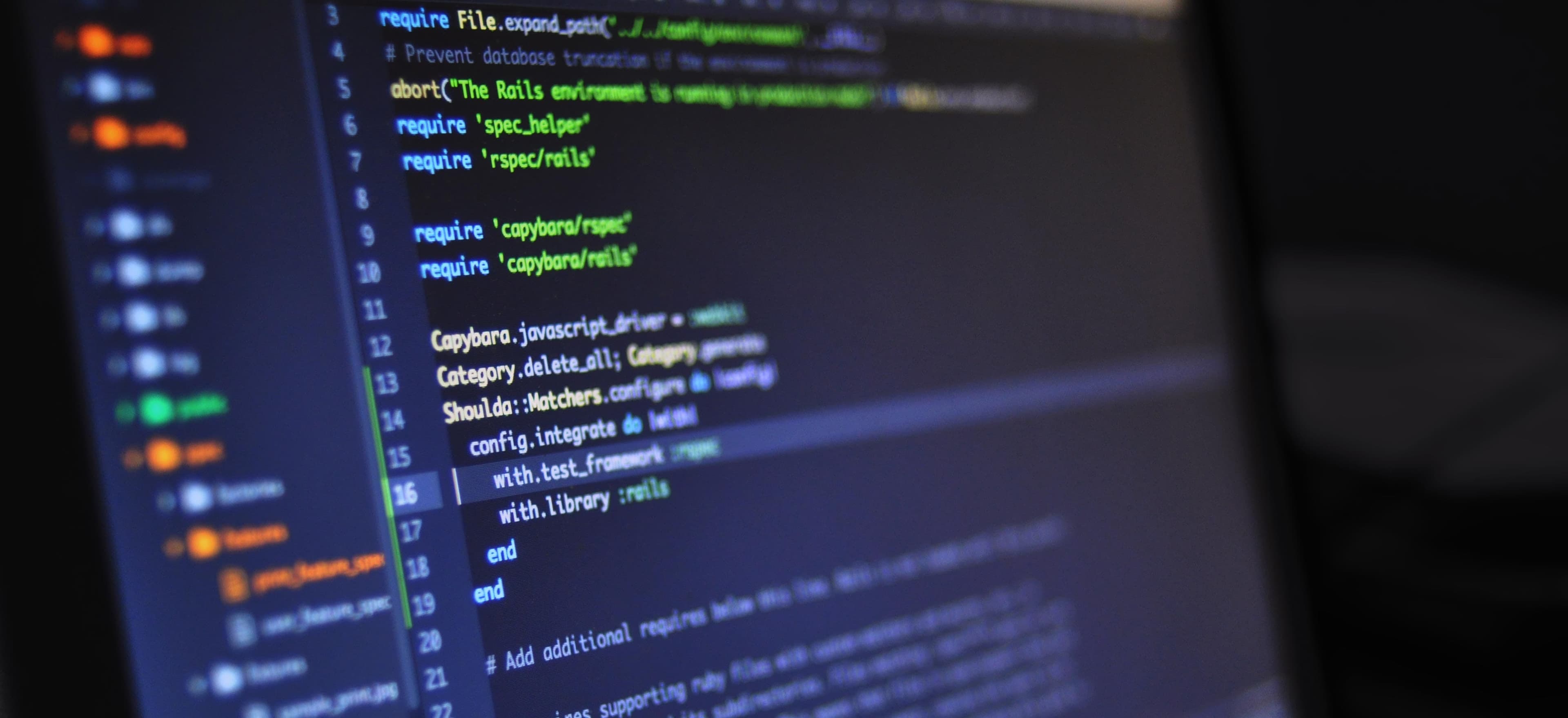
- Published on
Navigating Common JNI Edge Cases in Android Development
Java Native Interface (JNI) is a powerful framework that allows Java code running in the Java Virtual Machine (JVM) to call and be called by native applications and libraries written in C or C++. It’s commonly used in Android development when performance is critical—such as in game engines or processing heavy images.
Despite its advantages, working with JNI can introduce complexity and a variety of edge cases. This blog post explores some common edge cases you may encounter when using JNI in Android, providing you with practical code snippets and best practices to help you navigate these potential pitfalls.
Understanding JNI Basics
Before we dive into the common edge cases, let’s clarify what JNI is and how it functions. JNI serves as a bridge between Java and native code, allowing developers to leverage existing libraries or utilize lower-level system capabilities.
Here’s a simplified example of how JNI works:
- Load the Native Library: Use
System.loadLibrary("your_library")
in your Java code. - Declare Native Methods: Define the method with the
native
keyword. - Implement the Method in C/C++: Write the logic in a native C or C++ file.
- Compile the Native Code: Use the Android NDK to compile your code.
Here is a basic Java declaration and native method implementation:
public class MyNativeLibrary {
// Load the library
static {
System.loadLibrary("my_native_lib");
}
// Declare the native method
public native int add(int a, int b);
}
The corresponding C implementation may look like this:
#include <jni.h>
JNIEXPORT jint JNICALL Java_MyNativeLibrary_add(JNIEnv *env, jobject obj, jint a, jint b) {
return a + b;
}
Common Edge Cases in JNI
1. Memory Management Issues
One of the primary challenges with JNI is managing memory appropriately. Java handles memory with garbage collection, while C/C++ requires explicit memory management. This can lead to memory leaks or segmentation faults if not handled correctly.
Example:
When allocating memory in C/C++ and forgetting to free it can lead to memory leaks. Here’s how to safely manage memory:
JNIEXPORT jobject JNICALL Java_MyNativeLibrary_createObject(JNIEnv *env, jobject obj) {
jclass cls = (*env)->FindClass(env, "com/example/MyObject");
jmethodID constructor = (*env)->GetMethodID(env, cls, "<init>", "()V");
jobject myObject = (*env)->NewObject(env, cls, constructor);
// Perform operations...
// No need to manually free `myObject` - it will be garbage collected by Java.
return myObject;
}
Why?: The object created is automatically handled by the Java garbage collector. If you were to handle raw pointers manually, you’d have to ensure they're freed once done, which can be error-prone.
2. Incorrectly Handling JNI References
JNI uses local and global references to manage objects, and mismanagement can lead to application crashes.
- Local References: Created during a JNI call and valid only within that call.
- Global References: Persist beyond the current JNI call and must be explicitly released using
DeleteGlobalRef
.
JNIEXPORT void JNICALL Java_MyNativeLibrary_useGlobalRef(JNIEnv *env, jobject obj) {
jclass cls = (*env)->FindClass(env, "com/example/MyObject");
jobject globalRef = (*env)->NewGlobalRef(env, cls);
// Use the global reference...
// Clean up
(*env)->DeleteGlobalRef(env, globalRef);
}
Why?: Failure to release global references leads to memory leaks. Each global reference consumes memory, so it’s crucial to clean up after use.
3. Incompatible Data Types
Data type mismatches between Java and native code can cause crashes or incorrect behavior. Care must be taken when aligning data types, particularly with arrays and primitive types.
Example:
When passing arrays to native functions, consider their JNI specific types:
public native int[] processArray(int[] array);
And in your C code:
JNIEXPORT jintArray JNICALL Java_MyNativeLibrary_processArray(JNIEnv *env, jobject obj, jintArray array) {
jint *cArray = (*env)->GetIntArrayElements(env, array, 0);
jsize length = (*env)->GetArrayLength(env, array);
// Example to process data
for (jsize i = 0; i < length; i++) {
cArray[i] *= 2; // Example operation
}
// Create a new array for the result
jintArray result = (*env)->NewIntArray(env, length);
(*env)->SetIntArrayRegion(env, result, 0, length, cArray);
// Release cArray
(*env)->ReleaseIntArrayElements(env, array, cArray, 0);
return result;
}
Why?: The GetIntArrayElements
method retrieves a pointer to the data array, allowing for efficient manipulation. The ReleaseIntArrayElements
method ensures the memory is correctly released.
4. Threading Issues
JNI calls are not thread-safe, which means multiple threads manipulating the same JNI calls can lead to undefined behavior. Synchronization is critical when threading.
Example:
When accessing shared resources in JNI:
JNIEXPORT void JNICALL Java_MyNativeLibrary_threadSafeMethod(JNIEnv *env, jobject obj) {
// Assume mutex Lock is defined in your native code
pthread_mutex_lock(&mutex);
// Access shared resources...
pthread_mutex_unlock(&mutex);
}
Why?: Using mutex locks can prevent concurrent threads from executing the critical section at the same time, thus protecting shared resources from race conditions.
5. Mismanagement of Exception Handling
JNI provides ways to handle exceptions, but neglecting to manage exceptions can lead to frustrating errors. Always check for exceptions in your JNI code.
Example:
JNIEXPORT void JNICALL Java_MyNativeLibrary_divideNumbers(JNIEnv *env, jobject obj, jint a, jint b) {
if (b == 0) {
jclass exceptionCls = (*env)->FindClass(env, "java/lang/ArithmeticException");
(*env)->ThrowNew(env, exceptionCls, "Division by zero is not allowed");
return;
}
jint result = a / b;
// Do something with result...
}
Why?: Always handle edge cases that may throw exceptions. Failing to check can leave your application in an inconsistent state.
The Bottom Line
JNI is a robust method for integrating Java with native code but comes with its own set of challenges. By understanding and anticipating these common edge cases, you can build more reliable and efficient applications on the Android platform.
For more resources on JNI, you might find the official Java Native Interface Specification and Google's JNI best practices invaluable. By following best practices for exception handling, memory management, and type compatibility, you'll be well on your way to mastering JNI in your Android development projects. Happy coding!
Checkout our other articles