Common Pitfalls of Java Method Overloading and Autoboxing
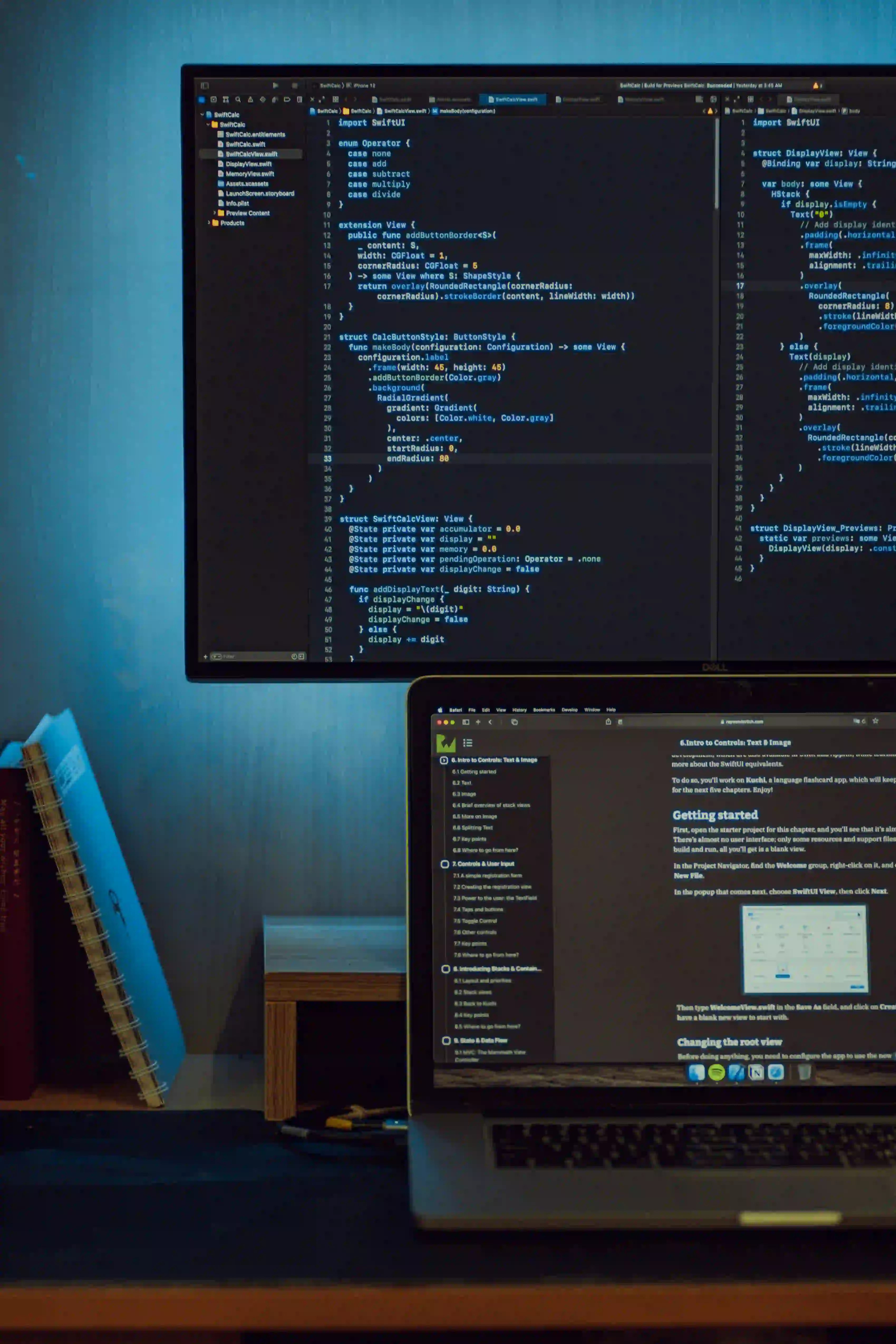
Common Pitfalls of Java Method Overloading and Autoboxing
Java is a powerful programming language that allows developers to create robust applications. Among its many features, method overloading and autoboxing represent capabilities that can enhance code efficiency and readability. However, they can also lead to confusion and potential bugs if not used correctly. This blog post will delve into the common pitfalls of these features, guiding you through best practices and pitfalls to avoid.
What is Method Overloading?
Method overloading allows you to create multiple methods within the same class that share the same name but differ in their parameter lists. This technique provides a way to perform similar functionalities with different inputs, making the code easier to understand and maintain.
Example of Method Overloading
Here's a simple example demonstrating method overloading:
class MathUtils {
// Method for adding two integers
int add(int a, int b) {
return a + b;
}
// Method for adding three integers
int add(int a, int b, int c) {
return a + b + c;
}
// Method for adding two double values
double add(double a, double b) {
return a + b;
}
}
In this example, the add
method has three variations: it can accept either two or three integer parameters or two double parameters. This design provides a clear approach to addition while maintaining a common method name.
Common Pitfalls of Method Overloading
While method overloading enhances code readability, developers often encounter pitfalls:
1. Ambiguous Method Calls
One of the significant pitfalls occurs when the compiler faces ambiguity. For instance:
void foo(float a, int b) {
System.out.println("float and int");
}
void foo(int a, float b) {
System.out.println("int and float");
}
foo(5, 10); // Error: ambiguous method call
In this case, when calling foo(5, 10)
, both methods are candidates since 5
is an int
and 10
is also an int
. There's no clear conversion path, leading to a compilation error. To resolve ambiguity, ensure that method signatures differ more distinctly.
2. Floating Point Precision
When overloading methods involving floating-point numbers, precision issues may arise. Consider the following:
void foo(double a) {
System.out.println("double");
}
void foo(float a) {
System.out.println("float");
}
foo(10); // Calls the double version due to widening conversion
The foo(10)
call will invoke the foo(double a)
method since 10
is implicitly promoted to double
for the best match. When designing overloaded methods, always be clear about the expected parameter types to prevent confusion.
3. Consistency in Parameter Types
When overloading, ensure coherent and predictable behavior across methods. Inconsistent behavior can lead to developer errors:
class Printer {
void print(String document) {
System.out.println("Printing String");
}
void print(int document) {
System.out.println("Printing Integer");
}
void print(Object document) {
System.out.println("Printing Object");
}
}
If a user passes an object of type Integer
, the last print
will be called, which might not be what the user intended. Strive for consistency in expected types.
4. Varargs and Overloading
Using varargs in overloaded methods can create further complexity. Consider the example:
class VarargsDemo {
void print(int... numbers) {
System.out.println("int varargs");
}
void print(int number) {
System.out.println("single int");
}
}
new VarargsDemo().print(1); // Calls the single int method
new VarargsDemo().print(1, 2, 3); // Calls the varargs method
This works, but it can easily lead to confusion if developers do not fully understand how varargs behave in the context of overload resolution.
What is Autoboxing?
Autoboxing is a feature introduced in Java 1.5 that automatically converts primitive types into their corresponding wrapper classes. For example, converting an int
to an Integer
. This facilitates easier handling of collections and enhances code readability.
Autoboxing Example
Consider the following:
List<Integer> intList = new ArrayList<>();
intList.add(5); // Autoboxing converts int 5 to Integer
In this case, the int
value 5
is automatically converted into an Integer
before being added to the list.
Common Pitfalls of Autoboxing
Despite its advantages, autoboxing can lead to several issues:
1. NullPointerException
One of the most frequent issues is NullPointerExceptions
. Here’s an example:
Integer num = null;
int value = num; // Throws NullPointerException
When attempting to assign a null
reference of Integer
to a primitive int
, a NullPointerException
is thrown. To avoid this, always check for null
before performing operations on potentially nullable types.
2. Performance Overhead
Autoboxing involves creating new objects for the wrapper classes. This can lead to performance issues, especially in tight loops or highly iterative applications:
for (int i = 0; i < 10000; i++) {
Integer num = i; // Autoboxing occurs here each iteration
}
Instead, if possible, refer to the primitive type to avoid unnecessary conversions:
for (int i = 0; i < 10000; i++) {
int num = i; // No autoboxing, direct usage of primitive type
}
3. Use of Collections
When using collections, be conscientious about autoboxing and unboxing. For example:
List<Integer> intList = new ArrayList<>();
intList.add(10); // Autoboxing
int value = intList.get(0); // Unboxing
While this seems seamless, be aware that mixing primitives and wrapper types in collections can lead to unexpected behavior and type mismatches.
4. Effects of Identity and In equality
Comparing Integer
objects can lead to counterintuitive results:
Integer a = 1000;
Integer b = 1000; // Two different Integer objects
System.out.println(a == b); // false, because they are different objects
Whereas:
Integer x = 100;
Integer y = 100; // Internally cached integer value
System.out.println(x == y); // true, because they point to the same cached object
Use .equals()
for comparing Integer
objects instead of ==
to avoid these pitfalls.
Conclusion
While Java's method overloading and autoboxing features enhance code readability and facilitate better design, they also introduce potential pitfalls. Understanding these pitfalls is crucial for developers looking to write efficient and maintainable code. By being aware of ambiguities in method calls, floating-point precision, coherence in parameter types, performance implications, and safe null handling, Java developers can harness the power of these features without falling into common traps.
For more information on method overloading, consult the official Java documentation, and explore additional resources on autoboxing here. Embrace these features with caution, and your Java experience will be much smoother.