Solving Java Enum Serialization: Common Pitfalls Explained
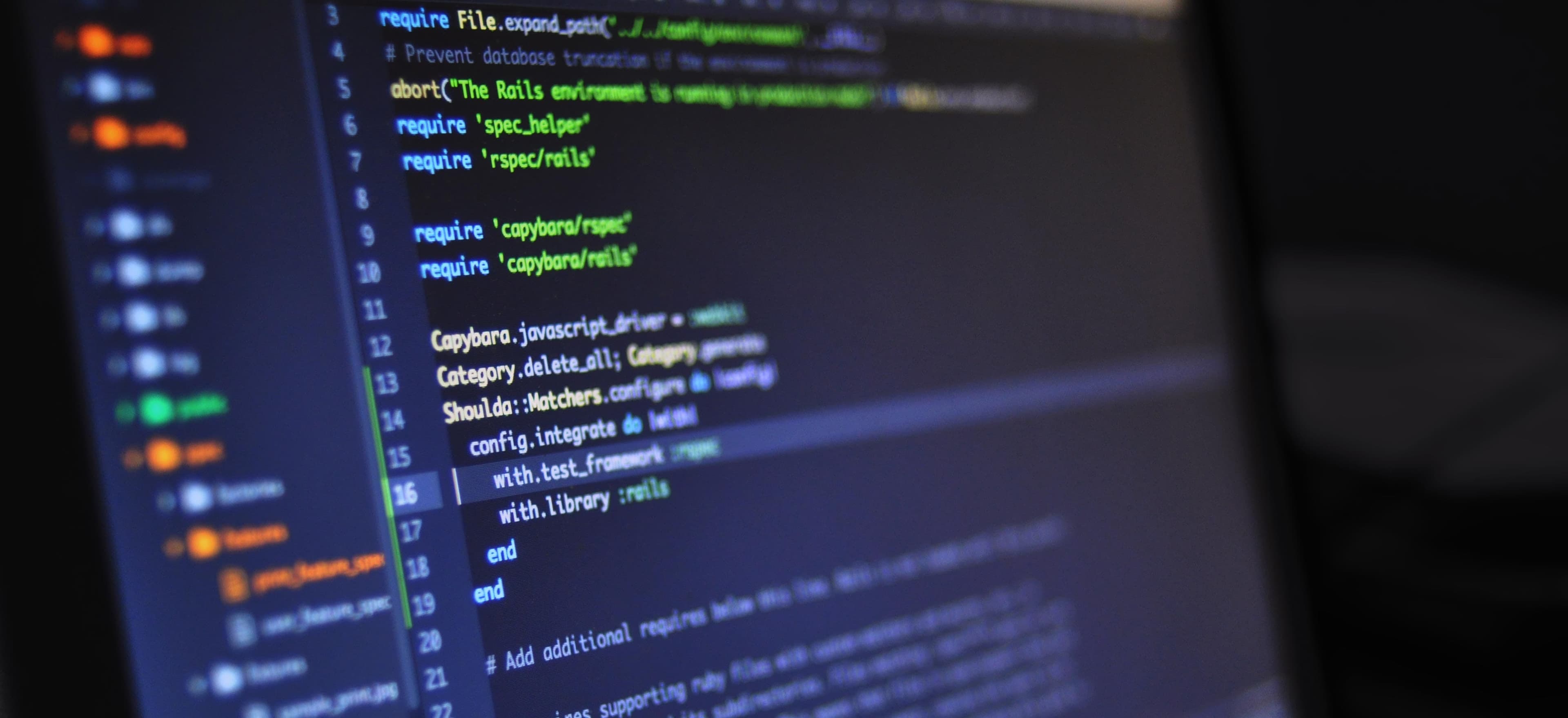
- Published on
Solving Java Enum Serialization: Common Pitfalls Explained
Serialization in Java allows an object to be converted into a byte stream, making it easy to save and retrieve objects. Enumerations (enums) in Java are a vital feature that allows developers to create a fixed set of constants. However, when it comes to serialization, enums can present unique challenges. In this post, we will explore common pitfalls associated with Java enum serialization and how to address them effectively.
What is Enum Serialization?
Before diving into the pitfalls, it's essential to understand what we mean by enum serialization. In Java, serialization is handled with the Serializable
interface. An enum automatically implements this interface, allowing its instances to be serialized without additional code. However, there are nuances that developers need to be aware of.
Why Use Enums?
Enums offer type safety, easy maintenance, and the ease of use in switch statements. Instead of using strings or integers to represent specific values, enums improve code readability.
public enum Days {
SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY;
}
In this snippet, we define an enum Days
that lists the days of the week. This straightforward structure promotes code clarity.
Common Pitfalls in Enum Serialization
1. Ignoring Serialization Versioning
One of the most frequent issues occurs when enums are modified. Since enums are singletons, any change to an enum's definition can break serialization compatibility. For example, if you add a new constant to an existing enum, deserialization of older serialized objects will fail.
Solution: Control Version with serialVersionUID
To overcome this, you can manually define a serialVersionUID
for your enums. Although enums already have this field by default, explicitly declaring it helps manage version control.
public enum Seasons implements Serializable {
WINTER, SPRING, SUMMER, FALL;
private static final long serialVersionUID = 1L; // Control versioning
}
By controlling your serialVersionUID
, you can manage deserialization exceptions that arise after an enum update.
2. Not Handling Enums as Constants
Enums should primarily represent immutable constants. If mutable state is introduced into enum instances, serialization can lead to unpredictable behavior. Avoid adding properties in enums that can change.
public enum Status {
ACTIVE("active"), INACTIVE("inactive");
private String value;
private Status(String value) {
this.value = value;
}
public String getValue() {
return value;
}
}
The above example is fraught with complications. Although it might seem functional, it introduces state into the enum. This can cause inconsistency if the state changes during serialization.
Solution: Keep Enums Stateless
Refrain from adding mutable attributes to your enums to ensure their immutability. Instead, use static methods or classes alongside your enums for similar objectives.
3. Custom Serialization and De-serialization
If you rely on specific serialization mechanisms (say, for additional fields), it’s essential to understand how to implement custom serialization methods using writeObject
and readObject
.
private void writeObject(ObjectOutputStream oos) throws IOException {
oos.defaultWriteObject(); // Calls default serialization
// Additional custom serialization logic here
}
private void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException {
ois.defaultReadObject(); // Calls default deserialization
// Additional custom deserialization logic here
}
While not common, if you have to include complex logic, ensure to maintain correct flow similar to above.
4. Using Enums in Switch Cases
While enums enhance switch case statements, it's vital to remember that the addition of new enums can affect switch logic. If your switch statements don't cover the new enum value, it may lead to unexpected behaviors or runtime exceptions.
public void printDay(Days day) {
switch(day) {
case MONDAY:
System.out.println("Start of week");
break;
// Missing cases may lead to unhandled enum values
}
}
Solution: Always Handle Default Case
When adding new enum values, it’s best to include a default case.
public void printDay(Days day) {
switch(day) {
case MONDAY:
System.out.println("Start of week");
break;
default:
System.out.println("Other day");
}
}
This approach ensures that you handle any unforeseen additions gracefully.
Serialization Best Practices for Enums
To effectively serialize enums, follow these best practices:
-
Define a Serial Version UID: Always have a
serialVersionUID
in your enums when they are expected to evolve. -
Maintain Immutability: Ensure your enums contain only final fields, avoiding mutable properties.
-
Minimize Changes: Try not to change enums frequently. If changes are unavoidable, consider alternatives or provide backward compatibility.
-
Validation during Deserialization: Implement validation in your custom
readObject
method to ensure that incoming serialized data adheres to expected rules. -
Error Handling: Add error handling logic to gracefully manage issues that arise during serialization and deserialization.
In Conclusion, Here is What Matters
Enums are powerful tools for creating a set of constants in Java. However, their serialization comes with specific pitfalls that can break applications if not handled correctly. By understanding enum serialization challenges and implementing best practices, developers can take full advantage of enums while avoiding common pitfalls.
If you are looking for a deep dive into Java serialization, consider checking out the Java Serialization documentation for more detailed insights.
Ultimately, maintaining enum integrity ensures your applications remain efficient, robust, and easy to maintain. Happy coding!