Uncovering Java Serialization Security Risks
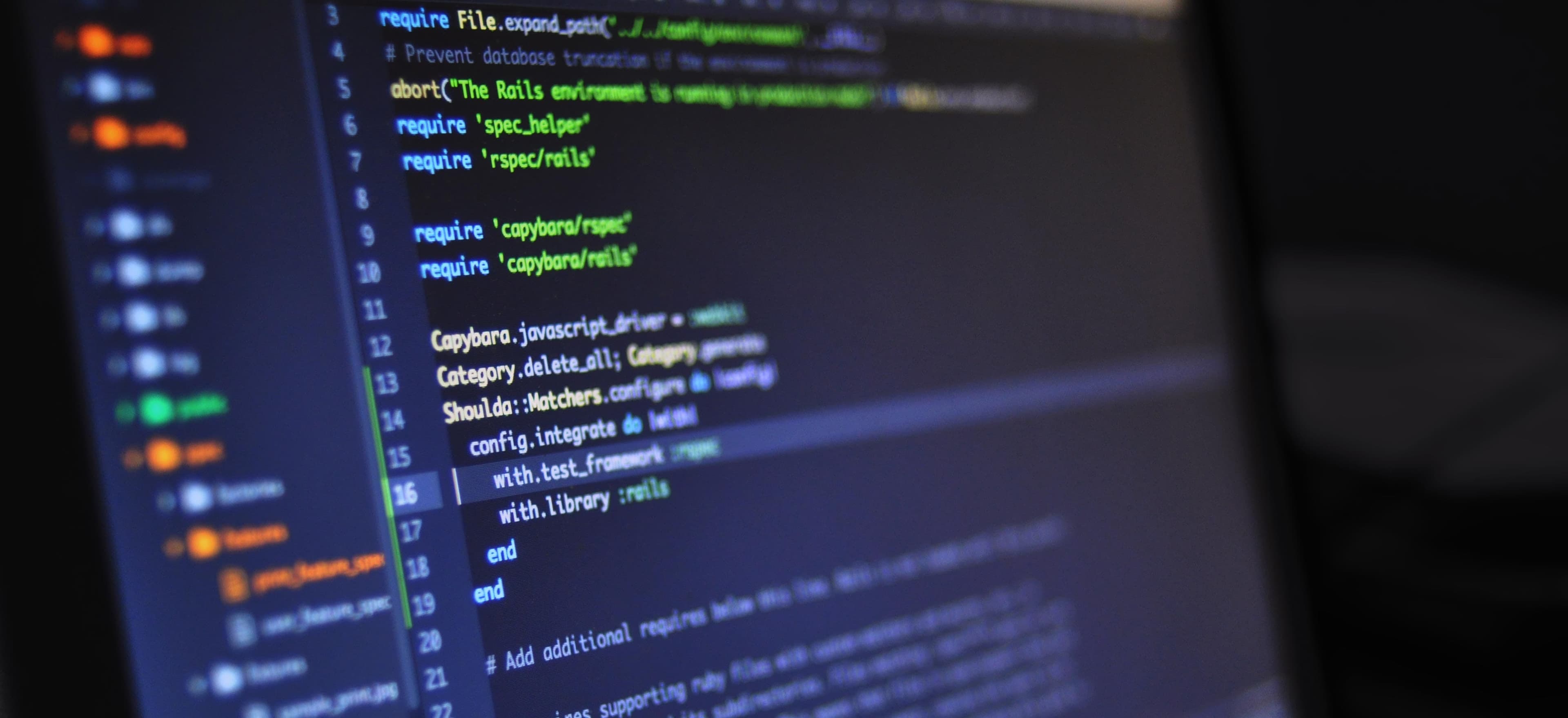
- Published on
Uncovering Java Serialization Security Risks
Serialization is a fundamental feature of the Java programming language, allowing developers to convert complex objects into a byte stream for storage or transmission. The ability to persist object state simplifies many programming tasks, particularly in distributed systems and Java-based web applications. However, this convenience comes with its own set of security risks. This post aims to uncover the potential dangers associated with Java serialization and how developers can mitigate these threats effectively.
What is Serialization?
Serialization in Java refers to the process of converting an object into a byte stream, which can then be written to a disk or transmitted over a network. Conversely, deserialization is the process of reconstructing the original object from the byte stream. Consider the following simple class that implements serialization:
import java.io.Serializable;
public class User implements Serializable {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Getters and setters omitted for brevity
}
In this example, the User
class implements the Serializable
interface, marking it as serializable. This enables instances of User
to be serialized and deserialized, for example, for saving user session data or sending user credentials across a network.
The Risks of Serialization
While serialization serves important purposes, it carries several security risks, particularly when it comes to deserialization. Here are the major vulnerabilities associated with Java serialization:
1. Deserialization Attacks
One of the most critical risks is the possibility of deserialization attacks. This occurs when untrusted data is deserialized by an application. A malicious user might manipulate the serialized byte stream, crafting it to exploit certain classes in the application. Popular methods of exploiting deserialization include:
- Creating instances of unexpected classes: Attackers can create arbitrary objects, potentially leading to remote code execution if those objects are handled improperly.
- Manipulating internal states: Attackers can alter the state of an object to disrupt the normal flow of the application, leading to denial-of-service (DoS) conditions.
2. Java Library Vulnerabilities
Certain Java libraries are known for their serialization vulnerabilities. An attacker may leverage these flaws to exploit your application. Libraries that do not adequately validate or sanitize input data are particularly susceptible to these risks. For example, the Apache Commons Collections
library has been implicated in multiple attacks due to unsafe deserialization.
3. Exposure of Sensitive Information
Serialized objects can inadvertently expose sensitive information. If a class contains sensitive attributes (like passwords), serializing and then deserializing that object can express those credentials in the application. An attacker who gains access to the serialized data can extract this information.
Mitigating Security Risks
Mitigating serialization risks requires a multi-pronged approach. Here are several strategies developers can implement:
1. Avoid Serialization When Possible
Whenever possible, avoid using Java serialization. Consider alternative strategies like:
- Manual Encoding/Decoding: Instead of relying on Java serialization, implement manual encoding processes using formats like JSON (using libraries such as Gson or Jackson). This will not only reduce the risk associated with arbitrary object creation but also make the data human-readable.
import com.google.gson.Gson;
public class User {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Convert to JSON
public String toJson() {
Gson gson = new Gson();
return gson.toJson(this);
}
// Create User from JSON
public static User fromJson(String json) {
Gson gson = new Gson();
return gson.fromJson(json, User.class);
}
}
In this approach, the User
class uses the Gson library for encoding user data securely into JSON, reducing the chance of unauthorized access to sensitive data.
2. Use a readObject
Method
If serialization is necessary, consider overriding the readObject
method. This method can be used to validate the contents of the serialized object before it gets deserialized. For example:
private void readObject(java.io.ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
// Validate the object state
if (username == null || password == null) {
throw new InvalidObjectException("Invalid User object");
}
}
This code checks that neither the username nor password is null after deserialization, improving security and preventing unexpected states.
3. Implement whitelisting
Another approach to secure deserialization is to implement a whitelisting mechanism, ensuring that only known, trusted classes can be deserialized. You can apply this by validating the object's class against a pre-defined list of accepted classes.
4. Limit Serialization Scope
Limit the implementation of Serializable
to objects that absolutely require it. By constraining the serialization and deserialization processes to a few key classes, you reduce the points of attack available to a potential adversary.
5. Update Dependencies Regularly
Security patches and updates frequently address serialization vulnerabilities in frameworks and libraries. Always ensure you are using up-to-date versions of libraries to minimize security risks. For more information on securing Java applications, refer to the Java Security Documentation.
Closing the Chapter
Java serialization presents substantial benefits to developers for object state persistence. However, it is crucial to remain vigilant about its associated security risks. By understanding the dangers of deserialization attacks and implementing proactive measures, you can create more secure Java applications. Consider switching to safer serialization techniques, validating incoming data, and minimizing the scope of serialization to maintain robust security in your coding practices.
By following these guidelines, developers can protect their applications from malicious actors while harnessing Java serialization's capabilities. Always prioritize security, remain updated with best practices, and consider alternative frameworks when it comes to handling sensitive data. Happy coding!
Checkout our other articles