Fixing the NoSuchFieldError in Java: Common Causes and Solutions
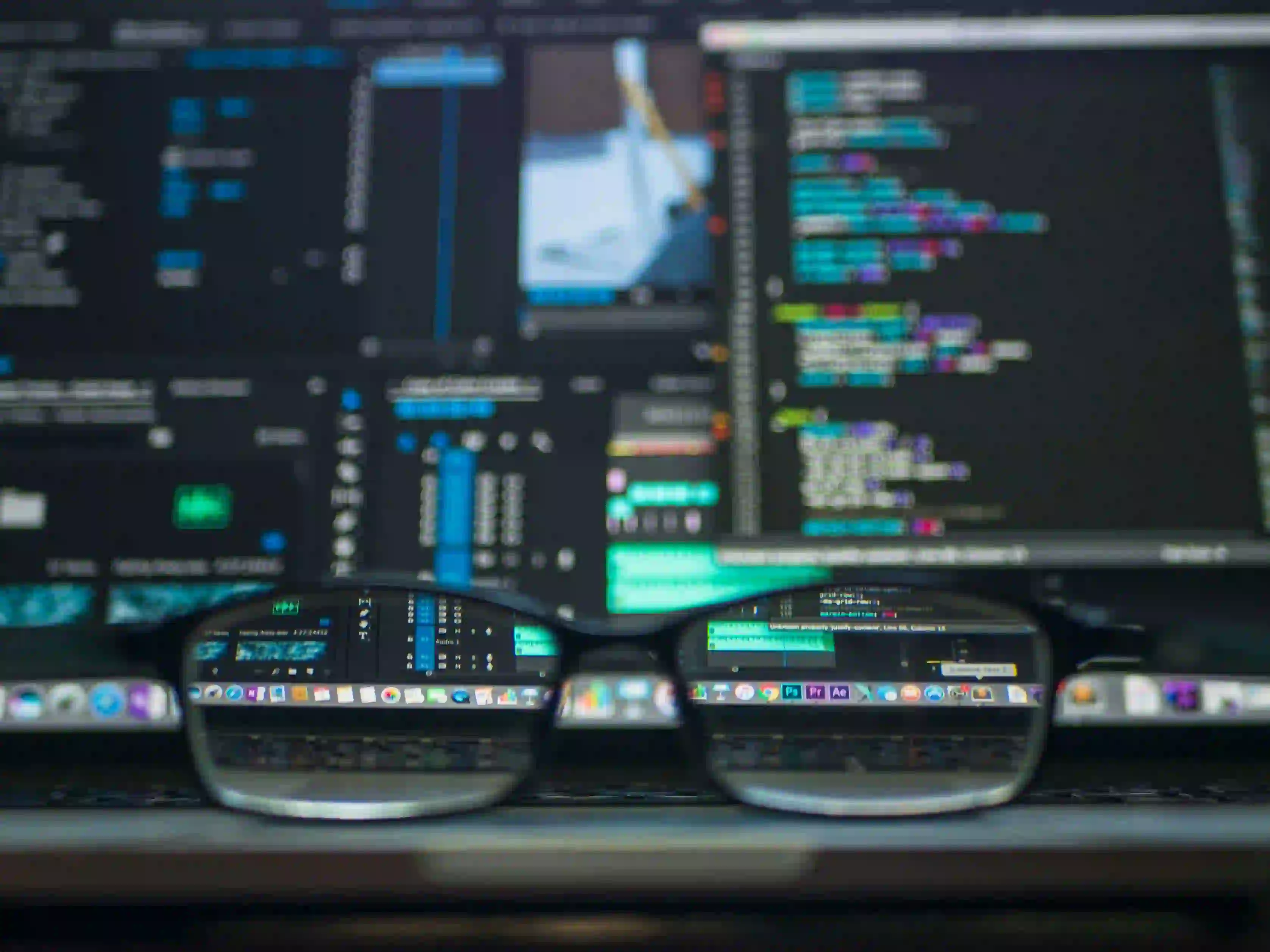
Fixing the NoSuchFieldError in Java: Common Causes and Solutions
In the world of Java programming, encountering errors is inevitable. One such error that developers may come across is the NoSuchFieldError
. This error can be perplexing, especially for those new to Java or working on complex projects. In this blog post, we will delve into what NoSuchFieldError
is, its common causes, and practical solutions to fix it.
Understanding NoSuchFieldError
NoSuchFieldError
is a runtime error that occurs in Java when the Java Virtual Machine (JVM) tries to access a field of a class but cannot find it. This typically happens when the class's bytecode has been updated, but the code attempting to access that field is still referring to an outdated or incompatible version of the class.
When Does NoSuchFieldError Occur?
The error usually surfaces under these conditions:
- Field Removal: When a field is removed from a class, but other parts of the code are still trying to access it.
- Inconsistent Builds: When different parts of your application reference mismatched versions of the same class.
- Reflection Operations: When using reflection to access fields that do not exist in the class at runtime.
// Example of NoSuchFieldError in a class
public class User {
// Imagine the 'age' field was removed in a refactored version
private String name;
// private int age; // This field has been removed
}
// Somewhere in the application code
public void printUserInfo(User user) {
// This will throw NoSuchFieldError if 'age' field no longer exists.
System.out.println("User's age: " + user.age);
}
In this scenario, if the age
field is removed from the User
class, any code attempting to access it will throw a NoSuchFieldError
.
Common Causes of NoSuchFieldError
1. Project Dependency Issues
One of the most common causes of NoSuchFieldError
is a discrepancy between the libraries or modules being used in a project. When using multiple dependencies, it’s crucial that they are all compatible with each other.
2. Classpath Confusion
Classpath confusion can occur when different versions of the same class exist on the classpath. This often happens in large projects or when collaborating with multiple libraries.
3. Refactoring Problems
After refactoring code, you may remove or rename a field that is still referenced elsewhere in the codebase. This can lead to this elusive error during runtime.
4. Inconsistent IDE Builds
Often, Integrated Development Environments (IDEs) do not automatically refresh the build. This can lead to stale class files that reference old or renamed fields.
How to Fix NoSuchFieldError
Now that we know what causes NoSuchFieldError
, let’s look into the potential solutions for tackling it.
1. Clean and Rebuild Your Project
The first approach to resolve this error is to perform a clean and rebuild of your project. The process clears out all previously compiled files and regenerates them.
For example, in Maven, you can do this using:
mvn clean install
In IntelliJ IDEA or Eclipse, use the Clean option from the build menu.
2. Check Project Dependencies
Inspect your project’s dependencies to ensure that they are all compatible. Run dependency checks to identify version conflicts and resolve them.
In Maven, you can see a dependency tree by running:
mvn dependency:tree
Resolve any issues appearing there. For Gradle users, the command is:
./gradlew dependencies
3. Use Reflection Carefully
If you are utilizing reflection, it is paramount to ensure that the fields you are trying to access exist in the runtime classes. Utilize checks and exception handling to catch and resolve runtime errors:
import java.lang.reflect.Field;
public void accessField(Object obj, String fieldName) {
try {
Field field = obj.getClass().getDeclaredField(fieldName);
field.setAccessible(true);
System.out.println("Field value: " + field.get(obj));
} catch (NoSuchFieldException e) {
System.out.println("Field '" + fieldName + "' does not exist!");
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
This method checks if the field exists before trying to access it, thus avoiding the NoSuchFieldError
.
4. Check Classpath and Build Systems
Ensure that your build system does not have conflicting or duplicate .class files. You can manually check your classpath to verify that you are not including outdated or incorrect versions of classes.
5. Use IDE Features for Synchronizing Classes
Most IDEs have options to synchronize your classes, pulling in the latest changes. Use these features if you notice any inconsistencies after updating your codebase.
Final Thoughts
Facing a NoSuchFieldError
in Java can be frustrating, particularly when working on large projects or collaborating with others. Understanding the causes of this error, from classpath issues to field removals, is key to diagnosing the problem effectively.
By employing strategies like cleaning builds, checking dependencies, and ensuring that your IDE settings are correct, you can eliminate this error and improve your development process.
For further reading, consider checking out Java's official documentation and explore resources on dependency management with Maven and Gradle.
By following these guidelines, you can fortify your code against NoSuchFieldError
, ensuring a smoother development experience in Java programming. Happy coding!