Boost Java Performance: Tackling Reflection Overhead
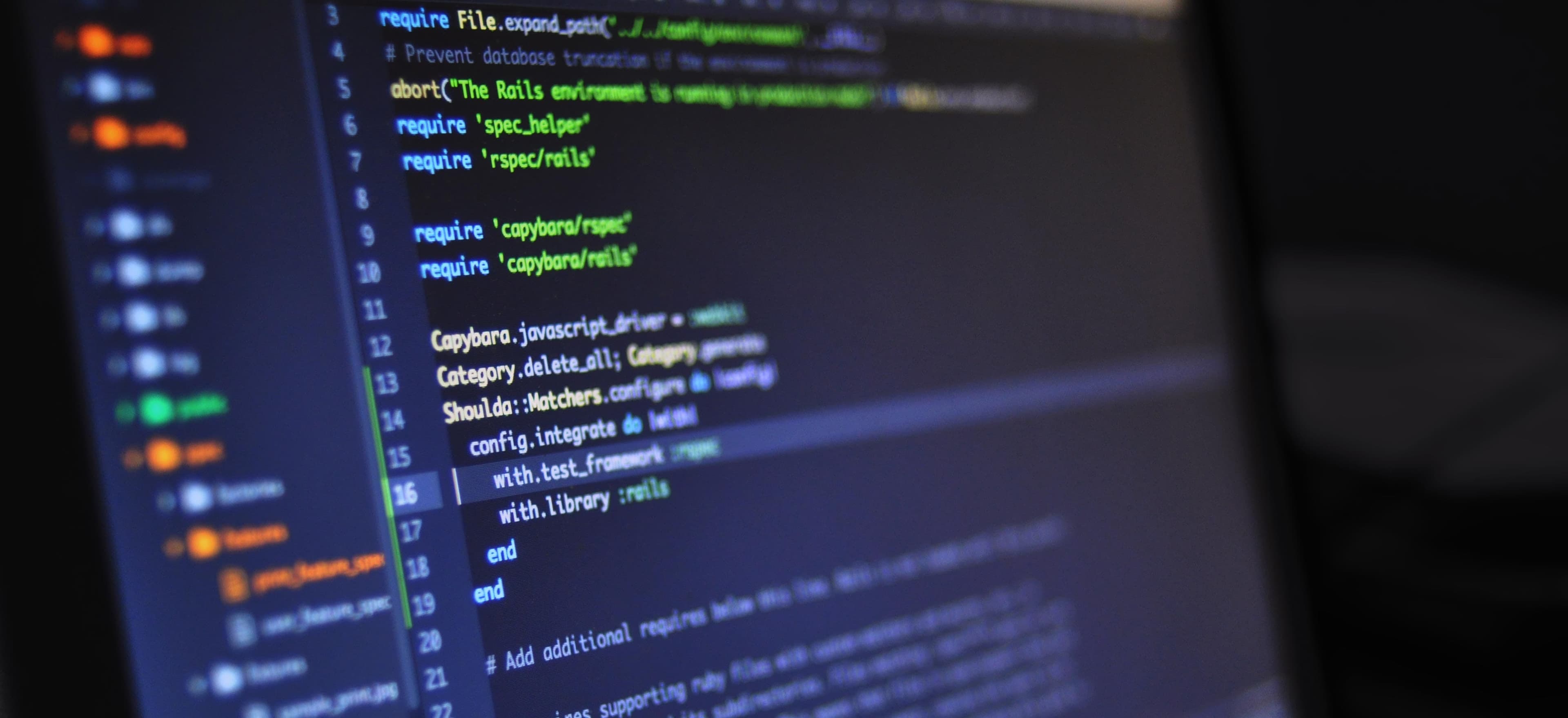
- Published on
Boost Java Performance: Tackling Reflection Overhead
Java is a powerful programming language known for its portability and rich feature set. However, with great power comes great responsibility, particularly when it comes to performance. One area that often causes performance issues in Java applications is reflection. In this blog post, we will explore what reflection is, how it affects performance, and how you can mitigate its overhead to boost your Java application's efficiency.
Understanding Reflection in Java
Reflection is a feature that allows a Java program to inspect and manipulate classes, methods, and fields at runtime. While this capability provides tremendous flexibility, it comes at a cost. Reflection is considerably slower than direct method invocations due to the overhead of dynamic type checking and the associated memory allocations.
Why Use Reflection?
Before diving into performance considerations, it is essential to understand why developers opt for reflection in the first place.
-
Dynamic Behavior: Reflection allows developers to implement dynamic features that might not be possible otherwise, such as creating instances of classes or invoking methods based on external configurations.
-
Frameworks: Many Java frameworks, such as Spring and Hibernate, heavily rely on reflection to provide a more dynamic and flexible API. For instance, Dependency Injection in Spring is facilitated through reflection.
A Quick Example of Reflection
To better understand how reflection is used in Java, consider the following code snippet:
import java.lang.reflect.Method;
public class ReflectionExample {
public void sayHello() {
System.out.println("Hello, World!");
}
public static void main(String[] args) {
try {
Class<?> clazz = Class.forName("ReflectionExample");
Object instance = clazz.getDeclaredConstructor().newInstance();
Method method = clazz.getMethod("sayHello");
method.invoke(instance);
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, we use reflection to call the sayHello
method dynamically. The Class.forName()
method fetches the class using its name, and Method.invoke()
is used to execute sayHello()
. While this is convenient, it can be costly in terms of performance.
The Performance Cost of Reflection
The performance drawbacks of reflection can be summarized as follows:
-
Dynamic Type Resolution: Each time you use reflection to invoke a method, the Java Virtual Machine (JVM) must resolve the method dynamically, which adds overhead.
-
Caching Limitations: Direct method calls can benefit from Just-In-Time (JIT) compilation optimizations. Reflection calls, on the other hand, bypass those optimizations and lead to increased execution times.
-
Access Modifiers: When accessing private methods or fields, reflection may require additional checks and permissions, further complicating and slowing down the execution.
Example of Performance Comparison
To better illustrate the performance difference, let's compare direct invocation with reflection:
public class PerformanceComparison {
public void directMethod() {
// Direct method call logic
}
public void reflectionMethod() throws Exception {
Method method = this.getClass().getMethod("directMethod");
method.invoke(this);
}
public static void main(String[] args) {
PerformanceComparison comparison = new PerformanceComparison();
long startTime = System.nanoTime();
comparison.directMethod(); // Direct call
long directDuration = System.nanoTime() - startTime;
startTime = System.nanoTime();
comparison.reflectionMethod(); // Reflection call
long reflectionDuration = System.nanoTime() - startTime;
System.out.println("Direct Method Duration: " + directDuration);
System.out.println("Reflection Method Duration: " + reflectionDuration);
}
}
When executing this code, you'll likely notice a significant time difference between the execution durations of the direct method call and the reflection method call. This reinforces the need to rethink the use of reflection, especially in performance-critical applications.
How to Mitigate Reflection Overhead
-
Use Reflection Sparingly: Limit the use of reflection to areas where it is undeniably necessary. If a task can be performed without reflection, it is usually best to avoid it.
-
Caching Methods and Fields: One effective strategy to mitigate some of the performance overhead is to cache method or field references. This way, you can avoid repeated reflective lookups and benefit from faster access when the same method is called multiple times.
private Method cachedMethod; public void invokeWithCache() throws Exception { if (cachedMethod == null) { cachedMethod = this.getClass().getMethod("directMethod"); } cachedMethod.invoke(this); }
-
Compile-Time Solutions: Consider using annotations or compile-time processing (such as Lombok) that generate bytecode, thereby avoiding reflection altogether. This can help you maintain flexibility without sacrificing performance.
-
Analyze Your Use Cases: If you're developing a framework, analyze the use cases to decide whether reflection is the best approach. Sometimes, simpler designs like the use of interfaces and polymorphism can suit the need without the performance penalty.
-
Use Alternatives: Investigate alternative libraries that provide similar functionalities without reliance on reflection, like Java’s built-in functions or third-party libraries that promote type-safe programming.
Final Thoughts
While reflection in Java is a powerful tool, it comes with a performance price tag that cannot be ignored. By understanding the implications of reflection and identifying best practices, you can optimize your Java applications and improve their overall performance.
Being mindful of how and when to implement reflection will help ensure that your code is not only functional but also efficient. Remember, sometimes a simpler solution can be a more robust solution.
For more insights on improving Java performance, consider visiting the Oracle Java Performance documentation or reviewing approaches for optimizing Java Memory Management.
With these strategies in mind, you can tackle reflection overhead and boost the performance of your Java applications. Happy coding!
Checkout our other articles