Stop Memory Leaks: Master Anonymous Inner Classes!
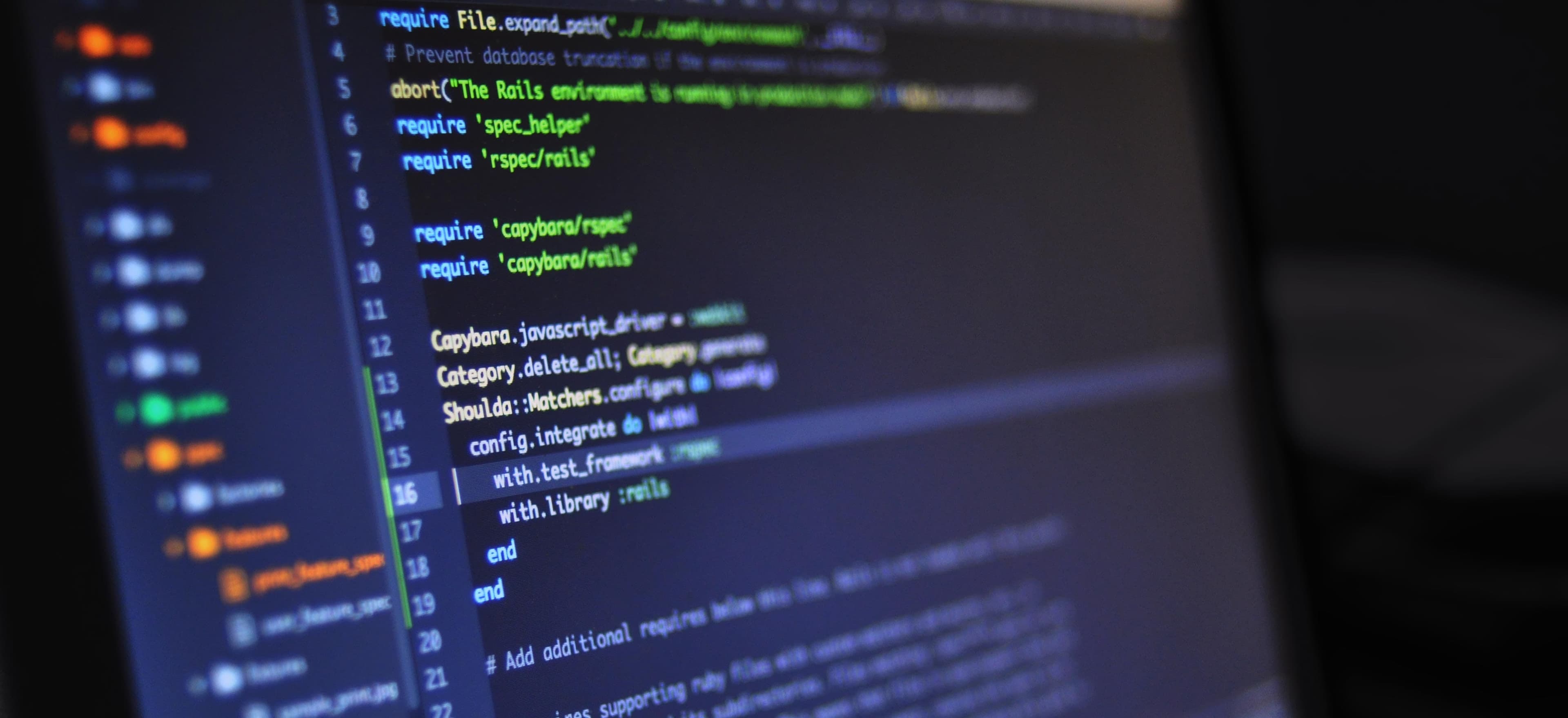
- Published on
Stop Memory Leaks: Master Anonymous Inner Classes!
Memory leaks can cripple an application, leading to performance issues, crashes, and poor user experiences. In Java, one common source of memory leaks is improper handling of anonymous inner classes. This post will delve into how to efficiently use anonymous inner classes while avoiding memory leaks.
Understanding Anonymous Inner Classes
An anonymous inner class is a class without a name that is defined within the body of a method. They enable concise coding and can be particularly useful when creating event listeners or running threads. They are defined in a single expression and can be useful for implementing interfaces or extending classes on-the-fly.
Here’s a basic example of an anonymous inner class:
public class OuterClass {
public void doSomething() {
// Creating an instance of an anonymous inner class
Runnable runnable = new Runnable() {
public void run() {
System.out.println("Anonymous Inner Class is running!");
}
};
new Thread(runnable).start();
}
}
Why Use Anonymous Inner Classes?
- Conciseness: Reduces boilerplate code. You don't need to explicitly define a separate class.
- Scoped Access: They can access the enclosing class's members, making them convenient for certain tasks.
- Encapsulation: They can help keep your code organized and maintainable.
The Memory Leak Dilemma
Despite their advantages, anonymous inner classes can cause memory leaks, particularly when they hold a reference to their enclosing class instance. This retention prevents the outer class from being garbage-collected, which can become problematic if the outer class is no longer needed.
Consider the following scenario:
public class MyActivity {
private String resource = "Resource";
public void createListener() {
// Memory leak alert! This anonymous inner class holds a reference to MyActivity
Button button = new Button();
button.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
System.out.println(resource);
}
});
}
}
In this case, if MyActivity
is no longer in use, and if the button still exists, the resource will never be eligible for garbage collection.
How to Avoid Memory Leaks with Anonymous Inner Classes
-
Use Static Nested Classes: If the additional functionality does not need access to the outer class, consider using static nested classes. They do not hold a reference to the outer class instance.
-
Weak References: Use
WeakReference
for instances of the outer class in your anonymous inner class. This way, the outer class can be collected if no strong references remain.
Code Snippet: Using Static Nested Class
Here’s an implementation that uses a static nested class to avoid memory leaks:
public class MyActivity {
private String resource = "Resource";
public void createListener() {
Button button = new Button();
button.setOnClickListener(new MyClickListener(this));
}
private static class MyClickListener implements OnClickListener {
private final WeakReference<MyActivity> activityReference;
public MyClickListener(MyActivity activity) {
activityReference = new WeakReference<>(activity);
}
@Override
public void onClick(View v) {
MyActivity activity = activityReference.get();
if (activity != null) {
System.out.println(activity.resource);
}
}
}
}
Analysis of the Code
In this example:
- WeakReference: It allows
MyActivity
to be garbage-collected if no other references exist. - Encapsulation: The click listener is now independent, enhancing modularity.
This approach effectively addresses potential memory leaks while allowing proper access to the outer class's resources.
Best Practices for Using Anonymous Inner Classes
To further master anonymous inner classes and their management in Java, consider adhering to these best practices:
-
Limit Scope: Keep anonymous inner classes short and simple. If the logic becomes complex, consider defining a named class.
-
Remove References: Ensure that anonymous inner classes do not hold strong references to the outer class’s context if they outlive the lifecycle of the outer class.
-
Utilize Lambdas: In Java 8 and above, utilize lambda expressions which provide a clearer and more concise alternative to anonymous inner classes, especially for functional interfaces.
Example of Lambda Expression
Here’s how you can simplify the code using a lambda expression:
public class MyActivity {
private String resource = "Resource";
public void createListener() {
Button button = new Button();
button.setOnClickListener(v -> System.out.println(resource));
}
}
Lambda expressions do not create a new instance of a class but rather implement the functional interface, making them a great way to avoid unnecessary references.
Final Considerations
In the world of Java, anonymous inner classes can be a double-edged sword. While they promote clean and concise code, improper use can lead to memory leaks that hinder application performance. By understanding the nuances of managing their lifecycles, leveraging best practices, and embracing modern alternatives like lambda expressions, Java developers can significantly reduce the risk of memory leaks in their applications.
For more detailed insights into Java memory management, consider exploring The Java Tutorials or the Java Memory Management documentation.
By mastering the art of using anonymous inner classes, you can not only write better code but also maintain a robust and high-performance application.
Checkout our other articles