Preventing Memory Leaks in Java ClassLoader Usage
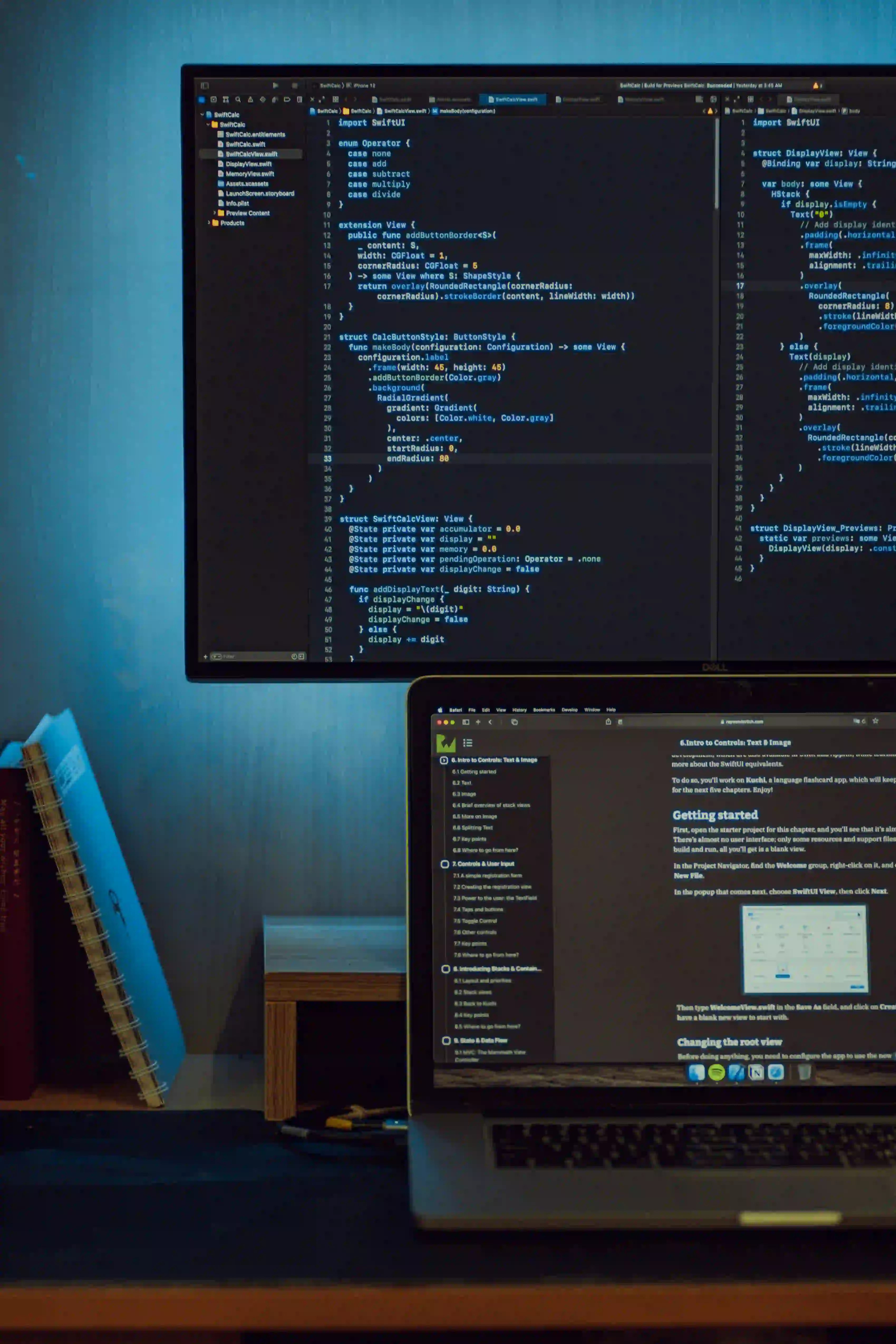
Preventing Memory Leaks in Java ClassLoader Usage
When dealing with dynamic class loading in Java, understanding how ClassLoader
works is crucial. While ClassLoader
is an essential aspect of Java's architecture that allows for modularity and dynamic code execution, improper usage can lead to memory leaks. In this post, we will explore how to use ClassLoader
effectively, identify common pitfalls that lead to memory leaks, and provide best practices to avoid issues in your Java applications.
Understanding ClassLoader
Before diving into memory leaks, it's essential to understand what a ClassLoader
is and how it operates. In Java, a ClassLoader
is responsible for loading classes at runtime. It handles the conversion of class files into Class
objects that the JVM can work with.
Java provides several built-in class loaders:
- Bootstrap ClassLoader: Loads core Java classes located in
jre/lib
. - Extension ClassLoader: Loads classes from the
jre/lib/ext
directory. - Application ClassLoader: Loads classes from the application's classpath.
// Example of obtaining the application class loader
ClassLoader classLoader = ClassLoader.getSystemClassLoader();
The Danger of Memory Leaks
When using custom class loaders, memory leaks can occur mainly due to the following reasons:
- Unreferenced Classes: Classes loaded by a custom
ClassLoader
will not be garbage collected if they are still being referenced. - Strong References: If your application maintains strong references to the
ClassLoader
or the classes it loads, it can prevent garbage collection.
Common Scenarios Leading to Memory Leaks
Let's look at some scenarios that can lead to memory leaks when using ClassLoader
.
1. Retaining References to Loaded Classes
When you load classes dynamically, retaining references to instances of these classes can lead to a buildup of uncollectable objects.
public class MemoryLeakExample {
private List<Object> instances = new ArrayList<>();
public void loadClass() throws Exception {
ClassLoader customClassLoader = new CustomClassLoader();
Class<?> clazz = customClassLoader.loadClass("com.example.SomeClass");
Object instance = clazz.getDeclaredConstructor().newInstance();
// Storing reference in a list
instances.add(instance); // Causes memory leak
}
}
In this example, even if SomeClass
is no longer needed, the reference in the instances
list prevents it from being garbage collected.
2. ClassLoader Leaks in Web Applications
Java web applications, particularly those developed using frameworks like Spring or JSP, are notorious for ClassLoader
leaks due to the lifecycle of the ClassLoader
.
public class MyServlet extends HttpServlet {
private ClassLoader myClassLoader;
@Override
public void init() {
myClassLoader = new CustomClassLoader();
myClassLoader.loadClass("com.example.SomeClass");
}
@Override
public void destroy() {
// Forget to nullify the ClassLoader reference
// myClassLoader = null; // This should be done to avoid leaks
}
}
In the destroy
method, if you do not nullify myClassLoader
, it holds onto the classes and all instances forever, thus leading to memory leaks.
Best Practices to Prevent Memory Leaks
Now that we've identified key areas that can lead to memory leaks when using ClassLoader
, let's explore best practices to mitigate these risks.
1. Deregister ClassLoader References
Always nullify any references to your custom ClassLoader
when they are no longer needed.
@Override
public void destroy() {
// Properly release references
myClassLoader = null;
}
2. Use Weak References
Consider using WeakReference
if you must retain references to loaded classes or class loader instances. This way, they can be collected by the garbage collector when memory is low.
import java.lang.ref.WeakReference;
public class WeakReferenceExample {
private WeakReference<Object> weakInstance;
public void loadClass(ClassLoader classLoader) throws Exception {
Class<?> clazz = classLoader.loadClass("com.example.SomeClass");
Object instance = clazz.getDeclaredConstructor().newInstance();
weakInstance = new WeakReference<>(instance); // Uses weak reference
}
}
3. Avoid Static Variables for Class References
Avoid using static variables for holding onto class references within your application, as they persist across application lifecycle and can lead to memory consumption.
4. Clean Up Resources
Always clean up resources during the application's shutdown procedure. Use ServletContextListener
in web applications to release resources and class loaders properly.
public class AppContextListener implements ServletContextListener {
@Override
public void contextDestroyed(ServletContextEvent sce) {
// Perform cleanup
MyServlet servlet = (MyServlet) sce.getServletContext().getAttribute("myServlet");
if (servlet != null) {
servlet.destroy(); // Clean up resources
}
}
}
Tools for Detecting Memory Leaks
Utilizing memory profiling tools can significantly aid in identifying memory leaks in Java applications:
- VisualVM: A tool that provides monitoring and profiling capabilities for Java applications Learn more here.
- Eclipse Memory Analyzer: A powerful tool to analyze memory dumps and find memory leaks in your applications Check it out here.
By regularly profiling your applications, you can catch leaks early and mitigate possible performance impacts.
Key Takeaways
Leaking memory through improper use of ClassLoader
can severely impact the performance and scalability of your Java applications. By understanding how class loaders function and adhering to best practices, developers can prevent these leaks effectively.
Employing strategies such as using weak references, properly managing class loader lifecycles, and utilizing profiling tools will help ensure that your applications remain efficient and responsive.
For further reading, explore the official documentation on Java ClassLoader to deepen your understanding.
By following the guidelines mentioned above, you can create robust Java applications that avoid the pitfalls of memory leaks. Dive deeper into your projects and assess how class loading is utilized; it may be the key to making your application more efficient.