Preventing ByteBuffer Underflow and Overflow in Java
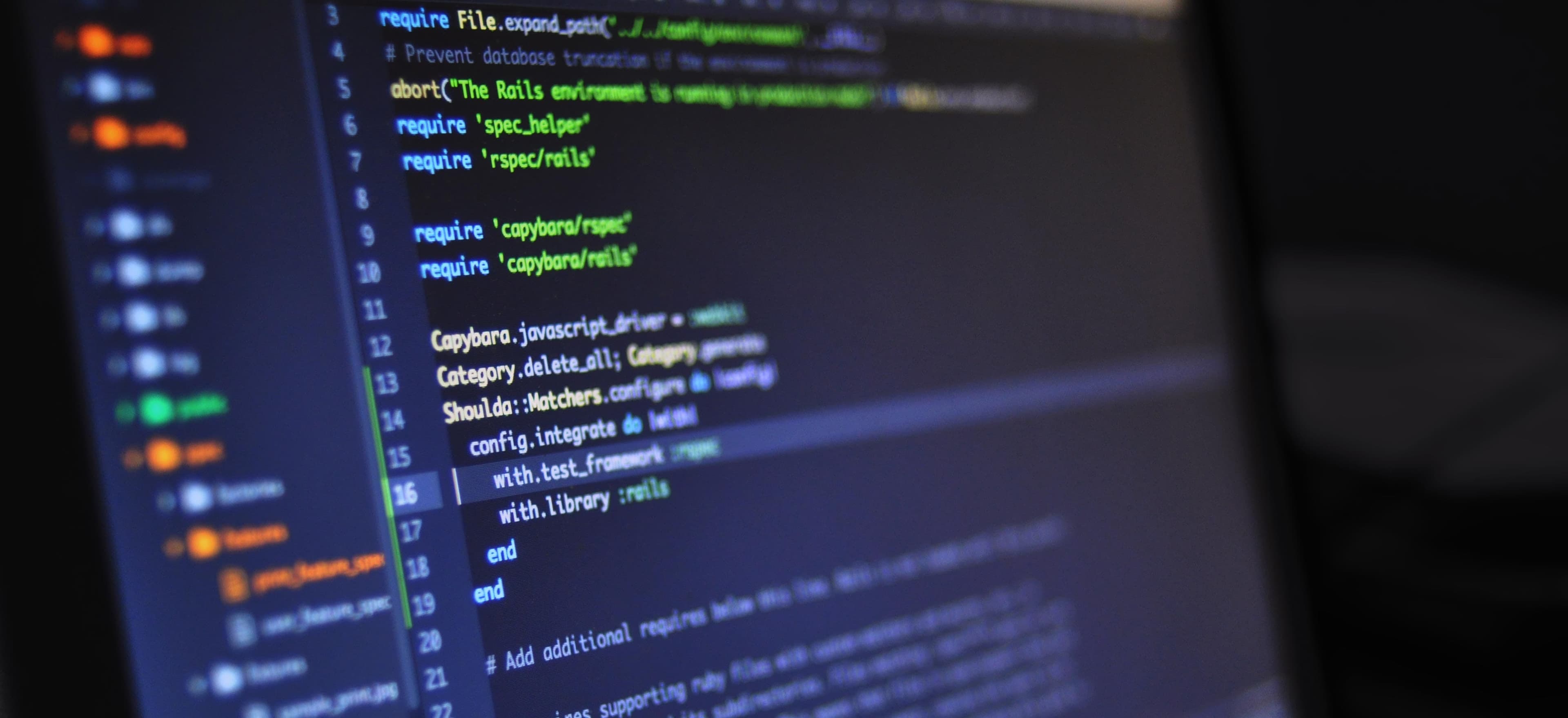
- Published on
Preventing ByteBuffer Underflow and Overflow in Java
Buffer management is crucial when working with low-level data processing in Java. The ByteBuffer
class, introduced as part of the Java NIO (New Input/Output) package, is high-performance and flexible for byte manipulation. However, improper usage can lead to bugs like underflow and overflow, which can cause unexpected behavior or program crashes. In this article, we will explore the concepts of underflow and overflow in ByteBuffer
, provide best practices to avoid these issues, and include code snippets to illustrate these points.
Understanding ByteBuffer
Before we can effectively prevent underflow and overflow, we must understand what ByteBuffer
is and how it works.
ByteBuffer
allows you to read and write bytes to and from a buffer. It offers various methods to manage the position, limit, and capacity of the buffer.
Key Concepts
- Position: The index of the next byte to be read or written.
- Limit: The index at which the buffer can no longer be read or written to.
- Capacity: The total number of bytes the buffer can hold.
When you read data from a ByteBuffer
, you might encounter underflow if you attempt to read beyond the available data. Conversely, overflow occurs when you write more data than the buffer can accommodate.
ByteBuffer Underflow
Underflow happens when you attempt to read from a ByteBuffer
that's depleted of data. The resulting BufferUnderflowException
indicates that you've tried to read more bytes than were available.
Preventing Underflow
-
Check Remaining bytes: Always check how many bytes remain before reading.
ByteBuffer buffer = ByteBuffer.allocate(10); // Suppose we want to read 5 bytes // First, check if there are enough bytes available if (buffer.remaining() >= 5) { byte[] bytes = new byte[5]; buffer.get(bytes); } else { System.out.println("Not enough bytes to read!"); }
In the code snippet above, we first check how many bytes remain in the buffer using the remaining()
method. If the available bytes are fewer than expected, we prevent an underflow by avoiding the read operation.
-
Use
hasRemaining()
method: This method returns true if there are more bytes to read.if (buffer.hasRemaining()) { byte nextByte = buffer.get(); } else { System.out.println("Buffer is empty, cannot read."); }
ByteBuffer Overflow
Overflow occurs when you write more data into the buffer than it can hold, leading to a BufferOverflowException
.
Preventing Overflow
-
Check Remaining Capacity: Applying a check before writing to ensure there’s enough capacity is essential.
ByteBuffer buffer = ByteBuffer.allocate(10); byte[] data = new byte[12]; // Data we want to write // Check if our buffer has enough space if (buffer.remaining() >= data.length) { buffer.put(data); } else { System.out.println("Not enough capacity to write data!"); }
In this example, the code checks the remaining space in the buffer before attempting to write data, thus preventing an overflow condition.
-
Limit the Buffer: You can also set the buffer's limit to prevent writing beyond it.
ByteBuffer buffer = ByteBuffer.allocate(10); buffer.limit(5); // Set limit to 5 bytes try { byte[] excessData = new byte[6]; buffer.put(excessData); // This will throw BufferOverflowException } catch (BufferOverflowException e) { System.out.println("Attempted to write beyond the set limit."); }
In the above example, the buffer's limit is set to 5 bytes, so an overflow will occur if we try to write more than that.
Advanced Buffer Management Techniques
Using Mark and Reset
The mark()
and reset()
methods in ByteBuffer
can be quite useful in managing where to read from or write to in your buffer.
ByteBuffer buffer = ByteBuffer.allocate(10);
buffer.put((byte) 1);
buffer.put((byte) 2);
buffer.mark(); // Mark the current position
buffer.put((byte) 3); // Further write beyond the mark
buffer.reset(); // Reset to the marked position
// Now the buffer will read starting from position marked earlier
byte firstByte = buffer.get(); // Retrieves byte 1
By using mark()
and reset()
, you can avoid issues that involve unwanted position changes that can lead to unexpected overflows or underflows.
Slicing the Buffer
Another useful feature is slicing, allowing you to create a new buffer view on the existing buffer. This can help manage buffer space better without risking underflow or overflow conditions.
ByteBuffer original = ByteBuffer.allocate(10);
for (int i = 0; i < 10; i++) {
original.put((byte) (i));
}
original.flip(); // Reset position to start reading
ByteBuffer slice = original.slice(); // Create a view on the original
// Modify slice without affecting original
slice.put((byte) 10);
The Last Word
Preventing underflow and overflow conditions in a ByteBuffer
requires proactive buffer management and discipline. Always check the limits and size of your buffer and employ methods such as mark()
, reset()
, and slicing to manage your data streams effectively.
In conclusion, the power of Java's NIO and the ByteBuffer
class lies in their flexibility. Understand these principles, and you will harness the full potential of the ByteBuffer while ensuring your application remains robust against common errors such as underflows and overflows.
For further reference, consider checking out the official Java NIO documentation for insights on the entire NIO package.
Happy coding!
Checkout our other articles