How to Effectively Handle Java Thread Interruptions
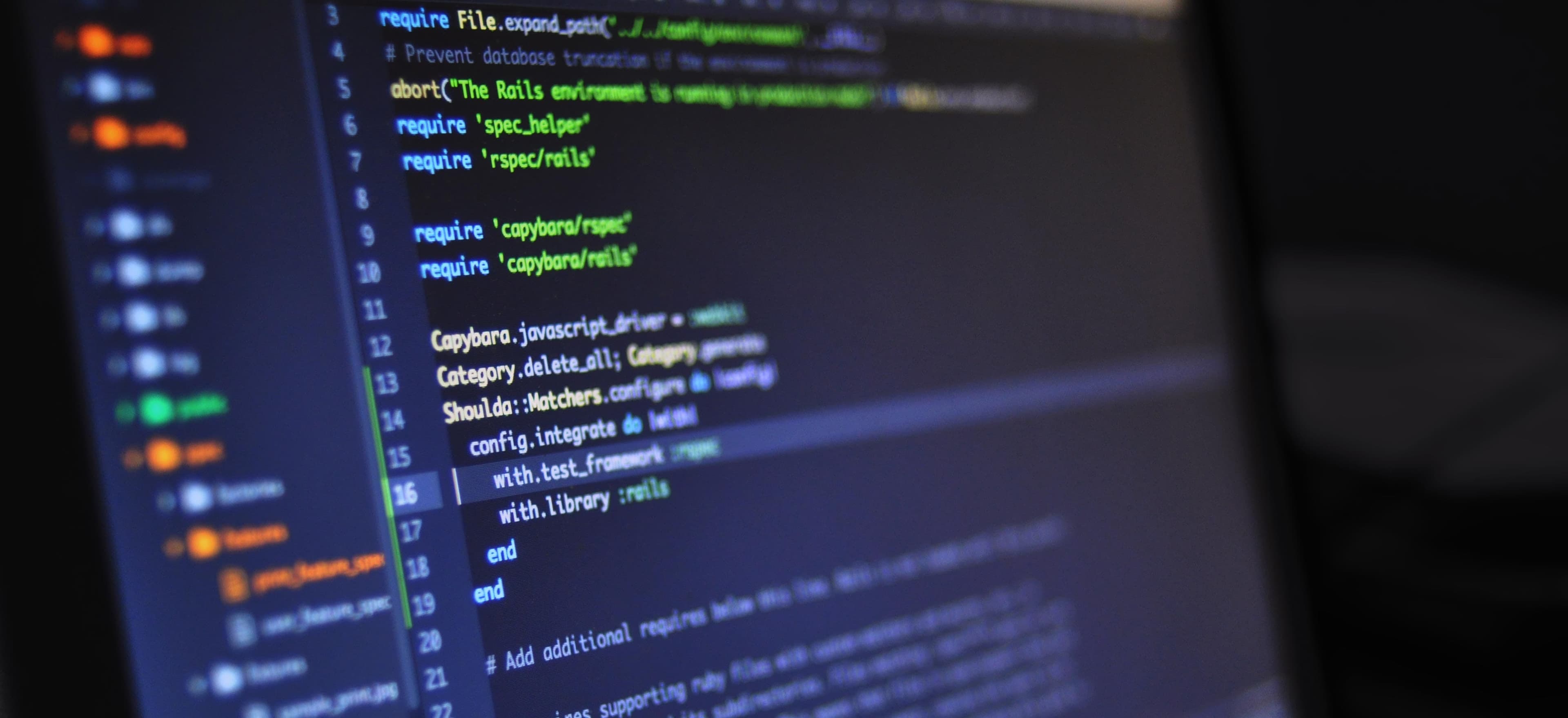
- Published on
How to Effectively Handle Java Thread Interruptions
Java, as an object-oriented programming language, is widely known for its multi-threading capabilities. One of the critical aspects of multi-threading in Java is handling thread interruptions effectively. Interruptions can signal threads to stop what they are currently doing or change their execution path. This blog post delves into the nuances of handling interruptions in Java threads, providing clear guidelines, best practices, and example code snippets to illustrate the concepts.
Understanding Thread Interruption
In Java, thread interruption is used as a cooperative mechanism to notify a thread that it should stop what it is doing. The interrupt mechanism is not inherently a "kill signal"; rather, it provides a way for threads to reactively decide how to proceed based on an interruption request.
When a threading interruption occurs, it is crucial to understand the state of the thread, which can be checked using the Thread.interrupted()
method or Thread.isInterrupted()
.
Why Use Thread Interruption?
-
Resource Management: Threads often handle resources like database connections or file streams. Proper interruption helps in releasing these resources gracefully.
-
Responsive Applications: UI applications or servers that need to remain responsive can use interruptions to handle user actions or shutdown requests effectively.
-
Graceful Shutdown: In systems where prolonged operations might need to be halted, interruptions can facilitate a graceful exit.
How to Interrupt a Thread
To interrupt a thread, a call to the interrupt()
method is necessary. Here’s how you can interrupt a thread:
public class InterruptExample {
public static void main(String[] args) {
Thread thread = new Thread(new Task());
thread.start();
// Interrupt the thread after some time
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
thread.interrupt(); // Sending interrupt signal
}
}
class Task implements Runnable {
@Override
public void run() {
while (!Thread.currentThread().isInterrupted()) { // Check if interrupted
try {
// Simulate some work
Thread.sleep(500);
System.out.println("Working...");
} catch (InterruptedException e) {
System.out.println("Thread was interrupted!");
Thread.currentThread().interrupt(); // Set the interrupt flag again
break; // Exit loop
}
}
System.out.println("Thread terminating gracefully.");
}
}
Code Explanation
- Thread Creation: The
Task
runnable performs a while loop checking for the interrupt status. - Sleep Handling: When a thread sleeps, it can throw
InterruptedException
if an interrupt occurs. This situation is handled gracefully by catching the exception and breaking out of the loop. - Re-Interrupted Flag:
Thread.currentThread().interrupt();
is called to preserve the interrupt status after catchingInterruptedException
.
Handling Interruption in Long-running Tasks
In scenarios involving long-running or blocking methods (like network calls), it’s essential to implement a strategy for interruptions. Here’s an example:
public class LongRunningTask implements Runnable {
@Override
public void run() {
try {
// Simulate long-running task
for (int i = 0; i < 10; i++) {
System.out.println("Processing " + i);
Thread.sleep(1000);
}
} catch (InterruptedException e) {
// Handling the interruption
System.out.println("Task interrupted during sleep.");
Thread.currentThread().interrupt(); // Preserve interrupt status
} finally {
System.out.println("Cleanup resources if necessary.");
}
}
}
Explanation
- Loop: The task processes in a loop for a simulated workload.
- Sleep Interruption: The thread can be interrupted while sleeping, in which case it exits gracefully.
- Finally Block: Always clean up resources when dealing with long-running tasks.
Best Practices for Handling Thread Interruptions
-
Always Check for
isInterrupted()
: Do this at logical points in your thread's execution. It prevents unintentional resource locks. -
Use
InterruptedException
Properly: When catching this exception, it’s a best practice to restore the interrupt status to ensure that the interrupt isn’t lost. -
Understand Blocking Methods: When using blocking methods (e.g., I/O operations), be aware that these methods may throw
InterruptedException
if interrupted. -
Document Code Behavior: Clearly document the expected behavior upon receiving an interrupt for ease of maintenance.
-
Cooperative Interruptions: Design tasks to be responsive to interruptions. Avoid indefinite waits or operations that do not periodically check for interruptions.
A Final Look
Handling thread interruptions is critical for creating responsive and robust multi-threaded applications in Java. It empowers your application to manage resources effectively and exit gracefully from long-running processes. By adhering to best practices and employing proper techniques, you can build systems that respond dynamically to user and system events.
For further reading on Java concurrency and threading, check out the official Java Concurrency API Documentation. Learning how to navigate Java's concurrency model will help you build more performant applications while ensuring they behave in predictable ways when faced with interruptions.
Remember, effective thread interruption management is not just about stopping threads—it's about letting them finish their tasks responsibly and gracefully. Happy coding!