Mitigating Java XML Parsing Vulnerabilities Effectively
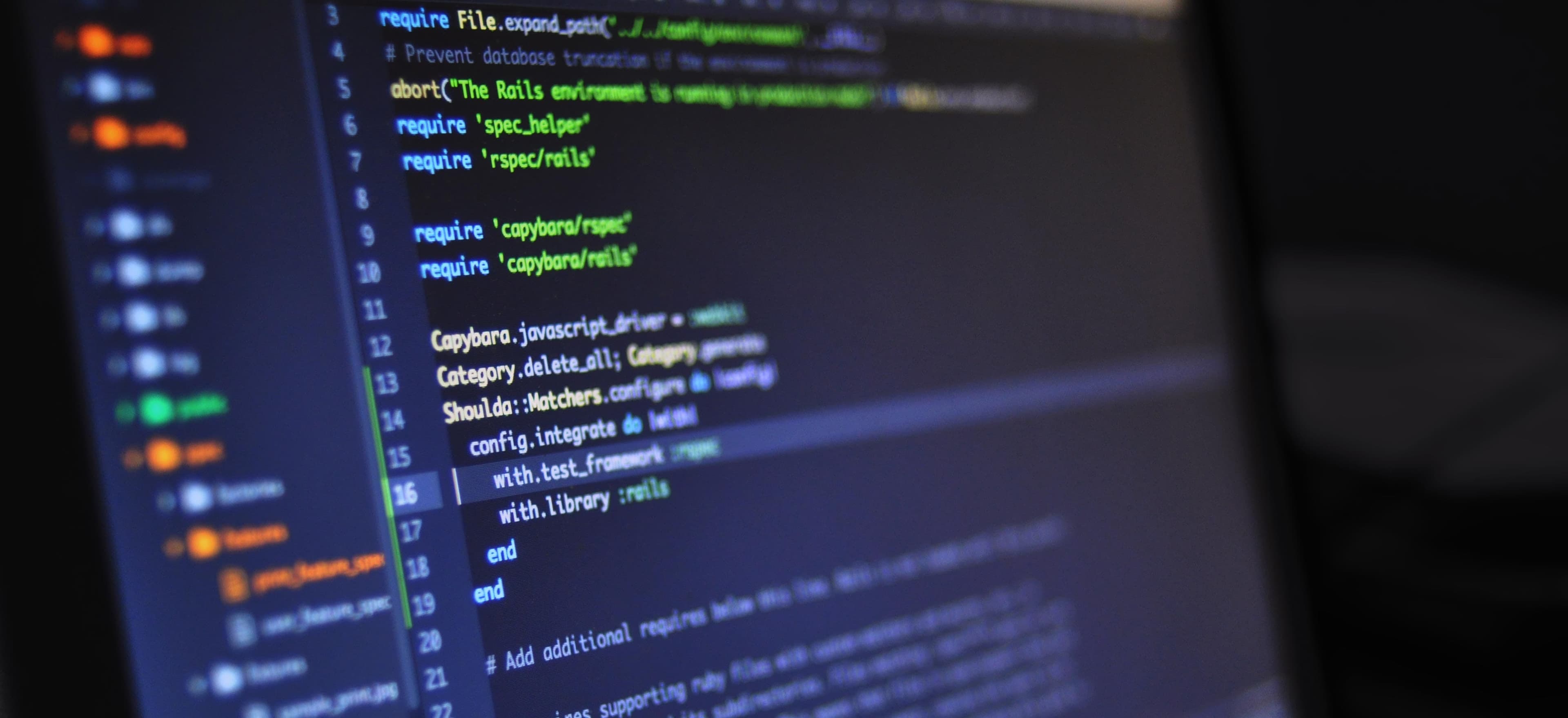
- Published on
Mitigating Java XML Parsing Vulnerabilities Effectively
Java provides robust libraries for XML processing, notably the Document Object Model (DOM), Simple API for XML (SAX), and the Java Architecture for XML Binding (JAXB). However, XML parsing inherent complexities introduce vulnerabilities such as Denial of Service (DoS) via XML External Entities (XXE) or Entity Expansion attacks. This blog post will discuss the vulnerabilities associated with Java XML parsing and provide practical mitigation strategies.
Understanding XML Parsing Vulnerabilities
1. XML External Entity (XXE) Attacks
XXE attacks are a common vulnerability that arises when XML input containing a reference to an external entity is processed by a vulnerable XML parser. This can lead to significant security issues, such as unauthorized file access or even network requests.
2. Entity Expansion Attacks
Entity expansion attacks, also known as the "billion laughs" attack, exploit the recursive nature of XML entities to create excessively large XML documents that can overwhelm systems and lead to DoS attacks.
3. Other XML Parsing Risks
- Injection Attacks: Malicious XML can manipulate application behavior.
- Data Exfiltration: Unauthorized access to sensitive files.
Mitigation Strategies
To safeguard applications from XML parsing vulnerabilities, comprehensive mitigation strategies must be employed. Here are some best practices:
1. Disable External Entities
When parsing XML in Java, disabling external entities is an essential step. Most XML parsers have options to disable DTDs (Document Type Definitions), which prevent the use of external entities.
Example with Java's DocumentBuilder:
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
public class SafeXMLParser {
public static Document parseXML(String xmlData) throws ParserConfigurationException {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
// Disable DTD processing
factory.setFeature("http://xml.org/sax/features/external-general-entities", false);
factory.setFeature("http://xml.org/sax/features/external-parameter-entities", false);
factory.setFeature("http://apache.org/xml/features/disallow-doctype-decl", true);
DocumentBuilder builder = factory.newDocumentBuilder();
return builder.parse(xmlData);
}
}
Why This Matters: By disabling external entity processing, we reduce the risk of XXE attacks significantly. The various features set to false ensure that the parser cannot access external resources, thus protecting sensitive data.
2. Use Secure Defaults
When configuring XML parsers, ensure all security features are set to secure defaults. For instance, in JAXB, you can turn off DTD processing via system properties.
Example for JAXB Context:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Unmarshaller;
import java.io.StringReader;
public class SecureJAXBParser {
public static Object parseXML(String xml) throws Exception {
JAXBContext context = JAXBContext.newInstance(MyObject.class);
Unmarshaller unmarshaller = context.createUnmarshaller();
// Disable DTD processing
System.setProperty("com.sun.xml.bind.v2.context.factory", "com.sun.xml.bind.v2.ContextFactory");
unmarshaller.setProperty("jaxb.formatted.output", true);
unmarshaller.setProperty("javax.xml.XMLConstants/property/accessExternalSchema", "all");
try (StringReader reader = new StringReader(xml)) {
return unmarshaller.unmarshal(reader);
}
}
}
Why This Matters: Adjusting system properties within JAXB provides another layer of security, ensuring that the parser adheres to safe practices even when dealing with potentially malicious XML content.
3. Implement Input Validation
Always validate the XML input against a schema (XSD). This validates the structure and ensures that the XML contains expected data types, which helps prevent injection attacks.
Example of Validation:
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
import javax.xml.XMLConstants;
import javax.xml.validation.Validator;
import org.xml.sax.SAXException;
import java.io.StringReader;
import javax.xml.transform.stream.StreamSource;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
public class XMLValidator {
public static void validateXML(String xmlData, String schemaPath) throws SAXException {
SchemaFactory factory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
Schema schema = factory.newSchema(new StreamSource(schemaPath));
Validator validator = schema.newValidator();
validator.validate(new StreamSource(new StringReader(xmlData)));
}
}
Why This Matters: Validating XML ensures that only correctly structured and intended data is processed. By outlining expectations with a schema, unexpected structures or data injections can be caught early.
4. Limit Resource Consumption
Configure the XML parser to limit resource consumption to mitigate DoS attacks. Setting limits on entity expansion and total XML document size can help prevent applications from consuming excessive memory or CPU.
Example with SAX Parser:
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
public class SafeSAXParser {
public void parse(String xml) throws Exception {
SAXParserFactory factory = SAXParserFactory.newInstance();
factory.setFeature("http://xml.org/sax/features/external-general-entities", false);
factory.setFeature("http://xml.org/sax/features/external-parameter-entities", false);
// Setting limits on resources
factory.setFeature("http://apache.org/xml/features/limit/entity-parameter", 100000);
factory.setFeature("http://xml.org/sax/features/limit/document", 100000);
SAXParser saxParser = factory.newSAXParser();
saxParser.parse(xml, new MyHandler());
}
}
Why This Matters: By implementing limits, you reduce the risk of overwhelming the system with large input files, preventing DoS conditions that affect performance and uptime.
5. Stay Updated with Security Patches
Regularly update your Java libraries. Security vulnerabilities in XML parsers may lead to severe issues, and most libraries frequently release patches to mitigate identified risks.
A Final Look
Mitigating Java XML parsing vulnerabilities requires a multi-faceted approach. By disabling external entities, setting secure defaults, validating input, limiting resource consumption, and staying updated on security patches, developers can significantly reduce the risk and impact of XML parsing vulnerabilities.
Incorporating secure coding practices into the development lifecycle is essential for maintaining the integrity and safety of applications. Ensure your team regularly reviews these practices and tailors them to suit your specific use cases.
By understanding both the risks and the methodologies available for mitigation, you can build XML parsing solutions that are secure, efficient, and resilient against threats.
For further reading on best practices for secure coding in Java, you may refer to the OWASP Java Coding Guidelines and Secure Coding Practices.
In implementing these practices, you can protect both your applications and users from potential security vulnerabilities related to XML parsing in Java. Take action today, and keep your software secure!