Solving Java AWT Threading Issues: A Developer's Guide
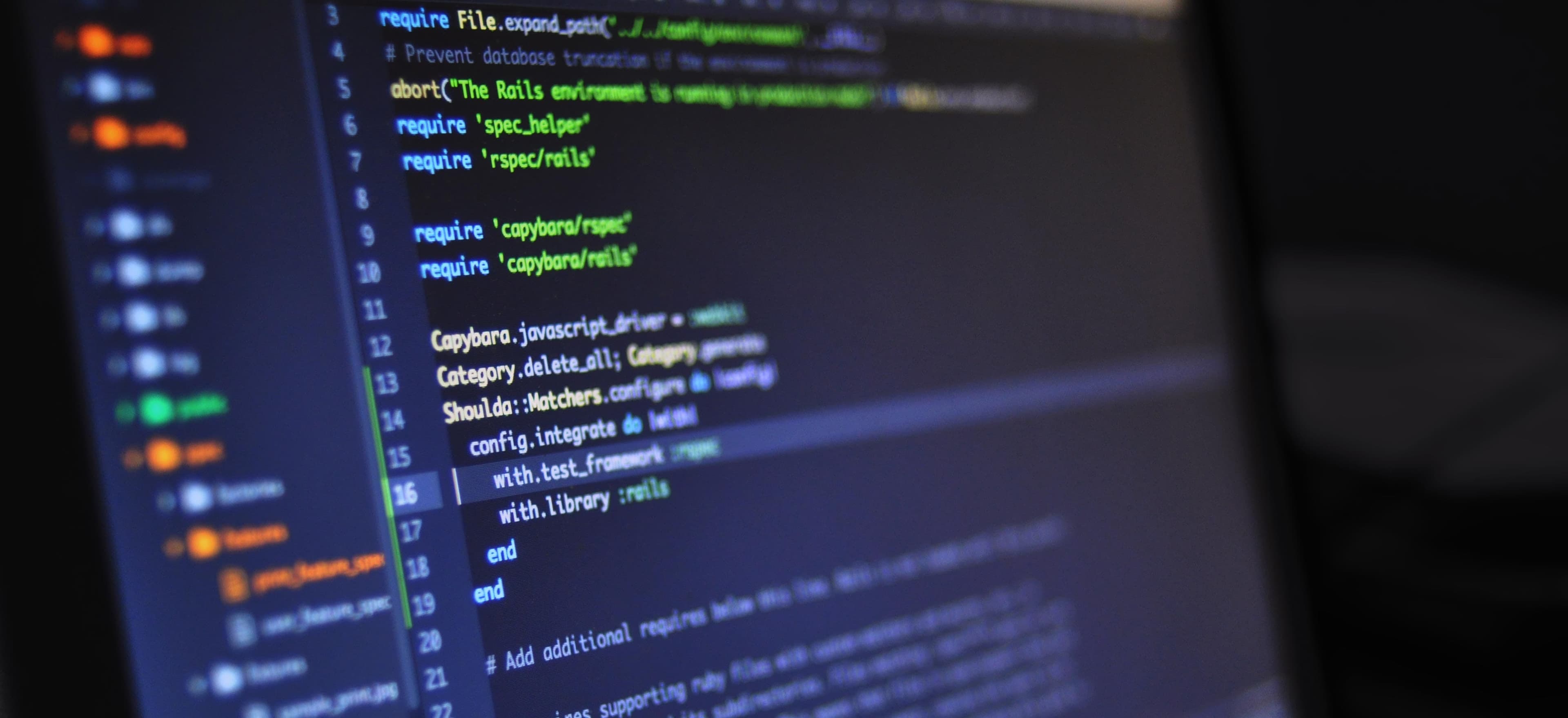
- Published on
Solving Java AWT Threading Issues: A Developer's Guide
Java AWT (Abstract Window Toolkit) provides a powerful framework for building graphical user interfaces (GUIs). However, when it comes to handling threading in AWT, developers often encounter challenges. In this post, we will explore common AWT threading issues and provide solutions, tips, and best practices to tackle them.
Understanding Threading in AWT
AWT is single-threaded by design. All the AWT components, such as buttons, text fields, and frames, must be manipulated on the Event Dispatch Thread (EDT). The EDT is responsible for handling all GUI-related tasks, including drawing components and processing user inputs.
When you perform long-running tasks (like fetching data from a database or a lengthy computation) on the EDT, you risk freezing the GUI, leading to a poor user experience. Hence, it is essential to understand how to manage threading correctly within AWT.
Key Concepts
- Event Dispatch Thread (EDT): This thread handles all GUI events. It should not be blocked for extended periods.
- Background Threads: These threads are used for long-running tasks to ensure the EDT remains responsive.
- SwingUtilities: A utility class that provides methods to manage painting and event handling on the EDT.
Common Threading Issues
Let's discuss some common threading issues and how to resolve them.
1. GUI Freezing
One of the most common issues developers face is GUI freezing when performing intensive operations directly on the EDT. For instance, consider the following code snippet:
import java.awt.*;
import javax.swing.*;
public class FreezingExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Freezing Example");
JButton button = new JButton("Start Long Task");
button.addActionListener(e -> {
// Performing a long task on the EDT
for (int i = 0; i < 1000000000; i++) {
// Simulating some work
}
System.out.println("Task Completed");
});
frame.getContentPane().add(button);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
In this example, the button click initiates a long-running task on the EDT, causing the GUI to freeze. The application becomes unresponsive until the task finishes.
Solution: Use Background Threads
To avoid GUI freezing, you can use a background thread. The SwingWorker
class is an effective way to perform background tasks. Here’s how you can modify the previous example:
import java.awt.*;
import javax.swing.*;
public class NonFreezingExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Non-Freezing Example");
JButton button = new JButton("Start Long Task");
button.addActionListener(e -> {
SwingWorker<Void, Void> worker = new SwingWorker<Void, Void>() {
@Override
protected Void doInBackground() throws Exception {
for (int i = 0; i < 1000000000; i++) {
// Simulating some work
}
return null;
}
@Override
protected void done() {
System.out.println("Task Completed");
}
};
worker.execute(); // Start the background task
});
frame.getContentPane().add(button);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
In this modified example, the long-running task is executed in the doInBackground()
method of SwingWorker
, allowing the EDT to remain responsive.
2. Updating GUI from Background Threads
Another common issue arises when developers try to update GUI components directly from a background thread. This can lead to exceptions or erratic behavior.
Example of Incorrect Usage:
button.addActionListener(e -> {
new Thread(() -> {
// Long task
button.setText("Task Completed"); // Attempting to update from a thread
}).start();
});
Solution: Use SwingUtilities.invokeLater()
The correct way to update GUI components from a background thread is by using SwingUtilities.invokeLater()
. This schedules the update to be run on the EDT. Here is the corrected version of the above code:
button.addActionListener(e -> {
new Thread(() -> {
// Long task
SwingUtilities.invokeLater(() -> {
button.setText("Task Completed"); // Safe update from EDT
});
}).start();
});
3. Handling Multiple Threads
In more complex applications, you may need to manage multiple threads performing various tasks. Care must be taken to ensure that these threads do not interfere with each other or the EDT.
Solution: Use SwingWorker
with Progress Notification
To manage multiple tasks and keep the progress updated, you can utilize the publish()
and process()
methods within the SwingWorker
. Here’s an illustrative example:
import java.awt.*;
import javax.swing.*;
import java.util.List;
public class MultiThreadExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Multi-Threading Example");
JButton button = new JButton("Start Tasks");
JProgressBar progressBar = new JProgressBar(0, 100);
button.addActionListener(e -> {
SwingWorker<Void, Integer> worker = new SwingWorker<Void, Integer>() {
@Override
protected Void doInBackground() throws Exception {
// Simulating multiple tasks
for (int i = 0; i <= 100; i++) {
Thread.sleep(50); // Simulate work
publish(i); // Send current progress
}
return null;
}
@Override
protected void process(List<Integer> chunks) {
int latestProgress = chunks.get(chunks.size() - 1);
progressBar.setValue(latestProgress); // Update progress bar
}
@Override
protected void done() {
System.out.println("All tasks completed!");
}
};
worker.execute();
});
frame.setLayout(new BorderLayout());
frame.add(button, BorderLayout.NORTH);
frame.add(progressBar, BorderLayout.SOUTH);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
In this example, we use publish()
to send intermediate progress during the execution of the long task, and process()
to update the GUI safely.
Best Practices for AWT Threading
-
Keep UI Updates on the EDT: Always ensure that GUI manipulations occur on the Event Dispatch Thread.
-
Use SwingWorker for Background Tasks: Utilize
SwingWorker
for long-running tasks that need to report progress or update the GUI upon completion. -
Avoid Blocking Operations: Never perform blocking operations on the EDT to maintain application responsiveness.
-
Leverage SwingUtilities: For any updates coming from background threads, use
SwingUtilities.invokeLater()
orSwingUtilities.invokeAndWait()
. -
Use Thread Safety Mechanisms: Use synchronized blocks or other thread safety mechanisms when necessary to prevent inconsistencies in shared data.
To Wrap Things Up
Threading in Java AWT can be tricky, but understanding the key concepts and applying best practices will significantly improve the user experience of your applications. By using SwingWorker
, and managing the EDT properly, you can create responsive and efficient GUI applications.
For further reading on AWT and Swing, check out Java Official Documentation or Java Swing Tutorial.
By following the strategies outlined in this guide, you can become adept at managing threading issues in Java AWT. Happy coding!