Navigating Java's Locale Pitfalls: Common Mistakes to Avoid
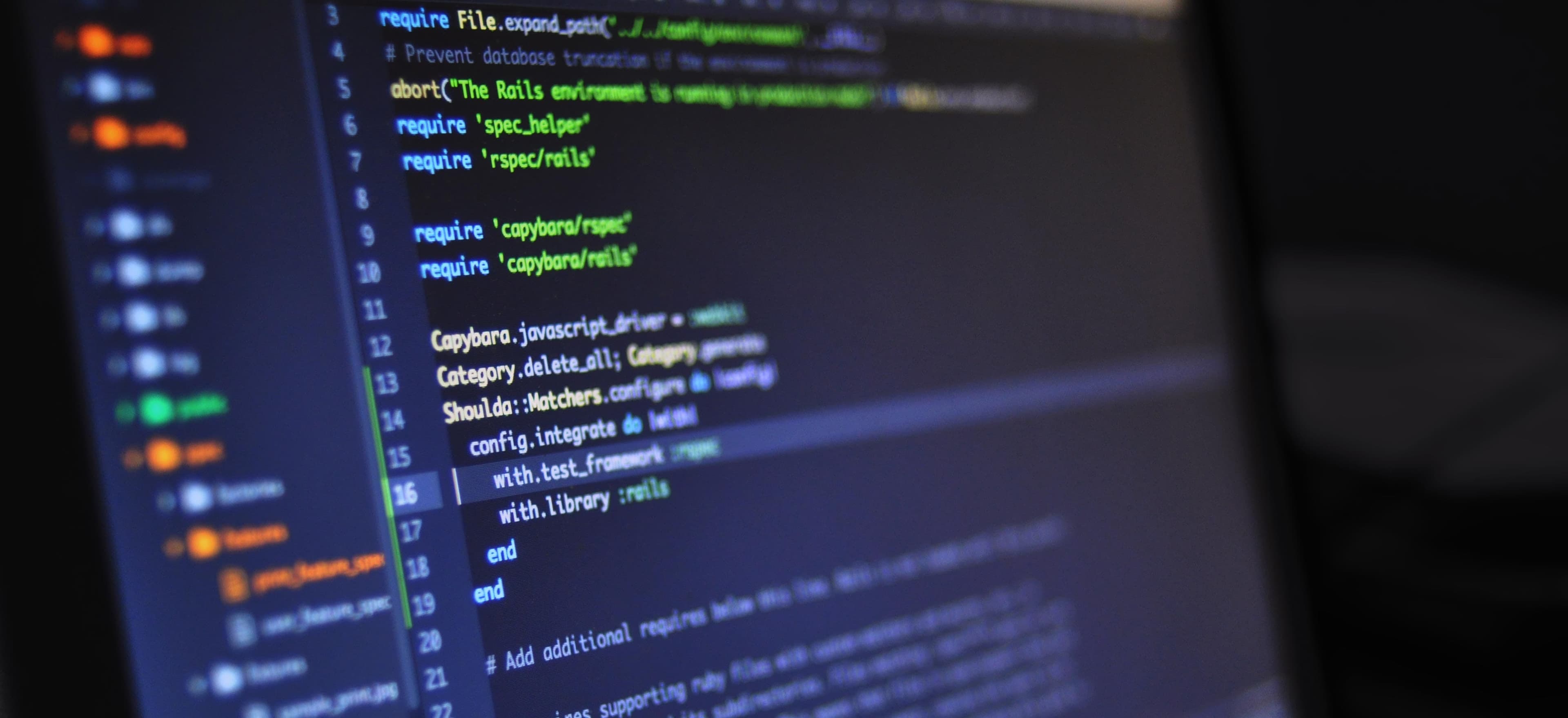
- Published on
Navigating Java's Locale Pitfalls: Common Mistakes to Avoid
Java, as one of the most widely-used programming languages, supports internationalization (i18n) through its Locale
class. However, many developers often encounter problems while working with locales, leading to bugs and user experience issues. In this blog post, we will explore common mistakes made with Java's Locale
and how to avoid them. By the end, you'll have a clearer understanding of how to implement locales correctly, resulting in more robust applications.
Understanding Locale in Java
The Locale
class is part of the java.util
package primarily used to tailor your program to the user's language and regional preferences. It consists of three main components:
- Language: The language code (e.g., "en", "fr").
- Country: The country code (e.g., "US", "FR").
- Variant: A code to specify dialects or regional differences (e.g., "POSIX").
Creating a Locale
object in Java is straightforward:
Locale locale = new Locale("en", "US");
This code initializes a new Locale
for English speakers in the United States. You can also create a Locale
using predefined constants:
Locale locale = Locale.US;
Knowing how to create locales is crucial. However, let's delve into the common pitfalls developers face.
Common Mistakes When Using Locale
1. Ignoring Default Locale
Mistake: Many developers forget that Java has a default locale. If no explicit locale is defined, the program falls back on the system's default locale, which can lead to unexpected behavior.
Solution: Always define a specific Locale
when dealing with user-facing functionalities like formatting dates, numbers, or messages.
Locale locale = Locale.forLanguageTag("fr-FR");
DecimalFormat df = DecimalFormat.getInstance(locale);
System.out.println(df.format(1234567.89)); // Outputs: 1 234 567,89
Defining the locale ensures consistent formatting regardless of the user's system settings.
2. Creating Locale Instances with Incorrect Language Codes
Mistake: Using incorrect or undefined language codes can lead to fallback to the default locale.
Solution: Always verify the language codes against the ISO 639 and country codes against the ISO 3166 standards.
Example of correctly creating locales:
Locale locale1 = new Locale("es", "ES"); // Spanish in Spain
Locale locale2 = new Locale("de", "DE"); // German in Germany
Using proper codes ensures the correct behavior of your application across different regions.
3. Hardcoding Locale Strings
Mistake: Hardcoding locale strings (like "en_US"
) makes the code inflexible and difficult to maintain.
Solution: Use the Java Locale
class to handle locale codes dynamically. This enhances readability and reduces errors.
String lang = "en";
String country = "US";
Locale locale = new Locale(lang, country); // Flexible approach
Keeping code dynamic allows changes without modifying hardcoded strings.
4. Misunderstanding Locale Sensitivity in Date Formatting
Mistake: Not accounting for locale differences in date formatting can severely impact user interpretation.
Solution: Utilize SimpleDateFormat
with the Locale
parameter. This guarantees the formatted date respects local conventions.
SimpleDateFormat sdf = new SimpleDateFormat("dd/MM/yyyy", Locale.FRANCE);
Date date = new Date();
System.out.println(sdf.format(date)); // Outputs: 10/10/2023 in France
Properly formatting dates considering locale can prevent confusion.
5. Failing to Localize Resource Bundles
Mistake: Developers may neglect to create resource bundles for different locales, causing all users to see the same hardcoded strings.
Solution: Define resource bundles for various locales. Use properties files for each locale (e.g., messages_en_US.properties
, messages_fr_FR.properties
).
A sample resource bundle might look like:
messages_en_US.properties
:
greeting=Hello
farewell=Goodbye
messages_fr_FR.properties
:
greeting=Bonjour
farewell=Au revoir
Load the bundle based on the user's locale:
ResourceBundle bundle = ResourceBundle.getBundle("messages", Locale.getDefault());
System.out.println(bundle.getString("greeting"));
This guarantees users see messages in their preferred language.
6. Summarizing Numbers Without Locale
Mistake: Formatting numbers without considering Locale
leads to misrepresentation of large values and decimals.
Solution: Rely on NumberFormat
which is sensitive to locale.
NumberFormat nf = NumberFormat.getCurrencyInstance(Locale.US);
System.out.println(nf.format(1234567.89)); // Outputs: $1,234,567.89
Always format numbers based on user locale to present data correctly.
7. Overlooking Locale in Collections and Sorting
Mistake: Developers sometimes ignore locale sensitivity when sorting strings, leading to incorrect order.
Solution: Use Collator
for locale-sensitive sorting.
Collator collator = Collator.getInstance(Locale.CHINESE);
List<String> names = Arrays.asList("张三", "李四", "王五");
Collections.sort(names, collator);
System.out.println(names); // Correctly sorted in Chinese order
Using Collator
ensures that you respect local customs in data presentations.
Summary
Understanding how to correctly manipulate Locale
objects is critical for developing internationalized applications in Java. Below is a quick recap of common pitfalls and their solutions:
- Set a Default Locale: Avoid relying on system defaults.
- Use Valid Language and Country Codes: Cross-check with ISO standards.
- Avoid Hardcoding: Keep locale strings dynamic for easier maintenance.
- Respect Formatting Rules for Dates and Numbers: Always use appropriate formatting based on locale.
- Localize Resource Bundles: Ensure all strings are appropriately translated for different locales.
- Utilize Locale-Sensitive Sorting: Use
Collator
for sorting.
By staying vigilant with these common pitfalls and embracing best practices, you'll ensure that your Java applications provide a seamless experience for users all over the globe.
Further Reading
Happy coding! With a stronger grasp of Locale
, your applications will be ready for a global audience.
Checkout our other articles