Resolving Memory Leaks with Java Phantom References
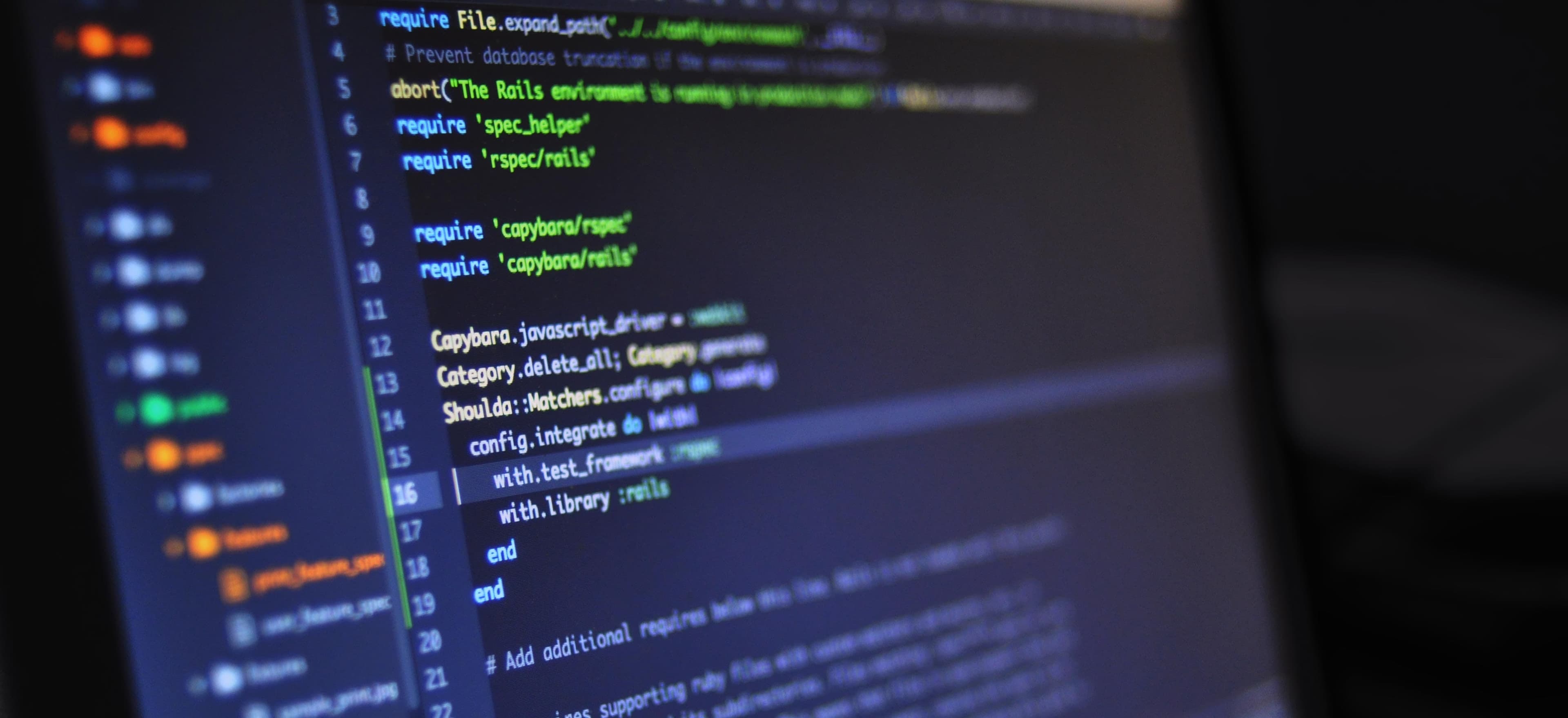
- Published on
Resolving Memory Leaks with Java Phantom References
Memory management in Java is a critical aspect that every developer must master. One of the most insidious challenges that can plague even the best-written applications is the phenomenon of memory leaks. Despite Java's automatic garbage collection, there are scenarios where objects remain in memory longer than necessary, leading to performance degradation and potential application crashes.
In this blog post, we will explore how Phantom References can serve as an effective tool for managing memory leaks and ensuring resource cleanup in Java applications.
Understanding Memory Leaks in Java
Before diving into Phantom References, it is essential to understand memory leaks. In Java, memory leaks typically happen when:
- Objects are unintentionally held in memory due to lingering references.
- Listeners or callbacks are not removed when no longer needed.
- Static collections are used but never cleared.
In cases like these, although the garbage collector is present, it cannot reclaim memory because there are still references held by the application.
Introducing References in Java
Java provides several types of references to manage the lifecycle of objects, which include:
-
Strong References: The default reference type. The garbage collector ignores these references, and thus, the object cannot be collected.
Object obj = new Object(); // Strong Reference
-
Weak References: Allow the garbage collector to reclaim the object when no strong references exist.
WeakReference<Object> weakRef = new WeakReference<>(new Object());
-
Soft References: Useful for caching, they are reclaimed only when the JVM is low on memory.
SoftReference<Object> softRef = new SoftReference<>(new Object());
-
Phantom References: Unlike the other reference types, Phantom References do not prevent the object from being collected, allowing for cleanup after the object’s memory has been reclaimed.
Phantom References are especially useful for performing cleanup actions after an object has been finalized and collected.
When to Use Phantom References
Phantom References come into play when you need to perform cleanup but don't want to interfere with the object's lifecycle. Here’s a common scenario:
- You have allocated native memory (e.g., using JNI) or you are holding onto some resources that need explicit release.
- After the garbage collector has finished its work, you need to perform these cleanup actions.
Setup of Phantom References
To create and manage Phantom References, Java provides the PhantomReference
class along with a ReferenceQueue
. This setup allows the developer to register a cleanup action to be notified when the Referent is ready to be reclaimed.
Basic Example of Phantom Reference
Let's see how to use Phantom References.
import java.lang.ref.PhantomReference;
import java.lang.ref.ReferenceQueue;
class Resource {
// A class representing some resource
int[] memory = new int[100000000]; // Simulating a large memory allocation
}
public class PhantomReferenceExample {
public static void main(String[] args) throws Exception {
ReferenceQueue<Resource> referenceQueue = new ReferenceQueue<>();
// Create a phantom reference for the Resource object
Resource resource = new Resource();
PhantomReference<Resource> phantomRef = new PhantomReference<>(resource, referenceQueue);
// Release original reference
resource = null;
// Force garbage collection
System.gc();
// Check the queue for the phantom reference
PhantomReference<Resource> refFromQueue = (PhantomReference<Resource>) referenceQueue.poll();
if (refFromQueue != null) {
System.out.println("Resource has been collected, performing cleanup.");
// Cleanup actions can be performed here
} else {
System.out.println("Resource is still alive.");
}
}
}
Code Explanation
- We create an instance of
Resource
, which holds a significant amount of memory. - A
PhantomReference
is established with aReferenceQueue
. When theResource
object is no longer strongly reachable, it will be placed into theReferenceQueue
. - The strong reference to the
Resource
is set tonull
to make it eligible for garbage collection. - We call
System.gc()
to prompt the garbage collector to run. - Finally, we check if the phantom reference has been enqueued, indicating that our resource was collected and we can now perform cleanup.
Benefits of Using Phantom References
-
Guaranteed Cleanup: Phantom References provide a callback mechanism through their associated
ReferenceQueue
, ensuring that cleanup happens after an object’s memory has been reclaimed. -
Memory Management: They help manage memory explicitly when dealing with resources requiring specific cleanup logic, such as I/O streams or network connections.
-
No Interference with Object Lifecycle: Using Phantom References does not interfere with the garbage collection process since they do not prevent the object from being collected.
Real-World Applications
Phantom References can be beneficial in scenarios such as:
- Caching Libraries: Where cleanup of cached objects is required without worrying about their lifecycle.
- Working with Native Resources: For example, when interfacing with C/C++ code using JNI, where you must free the native memory once the Java object is no longer reachable.
- Managing Temporary Resources: Such as temporary files or sockets that require explicit closure.
For further reading on memory management and garbage collection in Java, you can check out these links:
- Java Memory Management
- Understanding Java Garbage Collection
Final Considerations
Java's memory management is a complex and nuanced area, but with the appropriate use of Phantom References, developers can tackle memory leaks effectively. By ensuring that resources are cleaned up after use, you can maintain high performance and reliability in your applications.
While Phantom References might seem advanced at first, they are indispensable tools in a Java developer's toolkit. By understanding their use cases and implementation, you can prevent memory leaks and optimize the performance of your applications.
Implement these strategies in your code to take full advantage of Java's garbage collection capabilities without compromising on resource management. Happy coding!
Checkout our other articles