Navigating Null: Mastering Java Optional Edge Cases
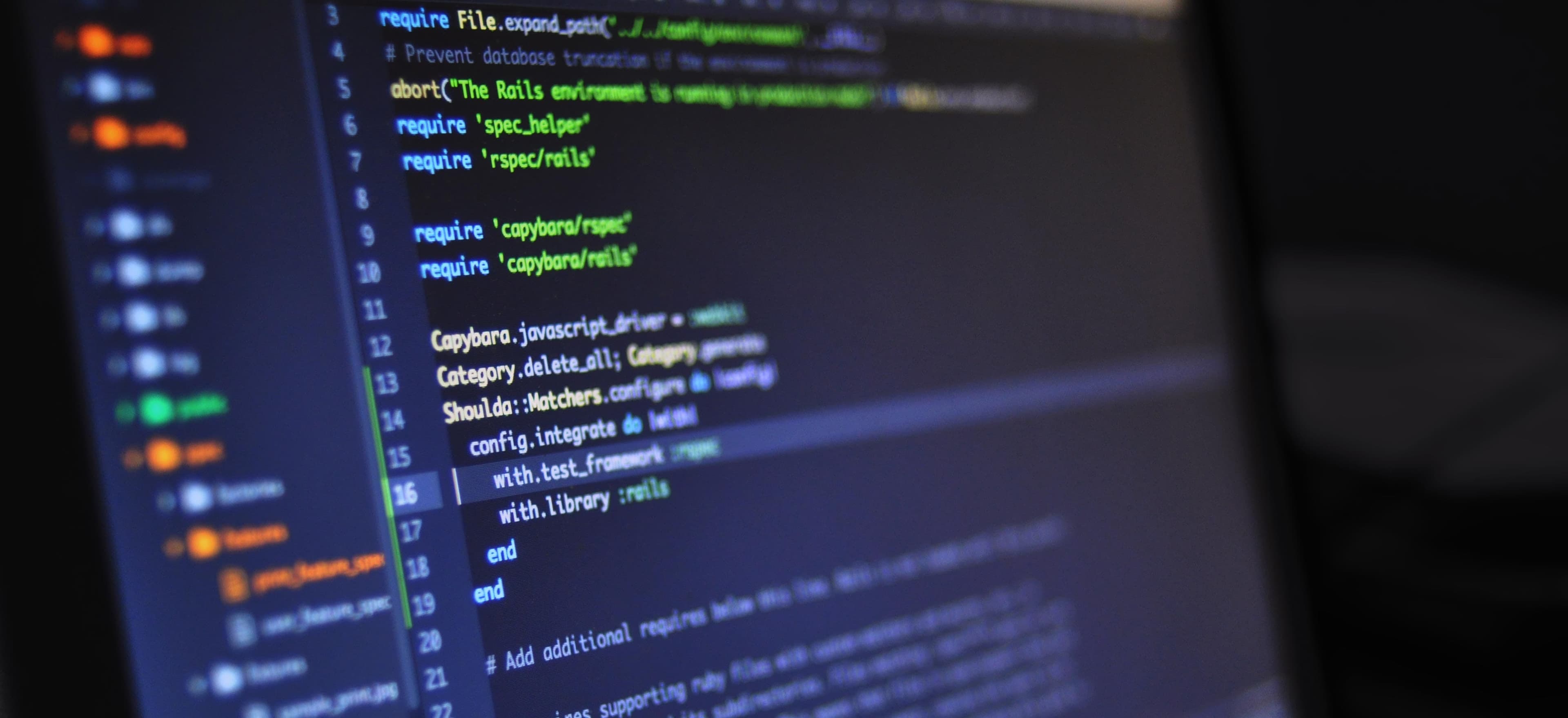
- Published on
Navigating Null: Mastering Java Optional Edge Cases
Java developers often face the challenge of handling null
values that can lead to NullPointerException
and make the code less readable and maintainable. As Java evolved, the introduction of the Optional
class gave developers a powerful tool to address this issue. In this post, we will dive deep into Java’s Optional
, its usage, and some edge cases you may encounter.
What is Java Optional?
Introduced in Java 8, the Optional
class is a container that may or may not contain a non-null value. It is intended to represent a potential absence of a value, promoting a more expressive and safer way of handling null scenarios. Using Optional
can lead to cleaner code and help you steer clear of NullPointerException
.
The Basic Usage of Optional
Here is a simple example demonstrating how to create an Optional
object:
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
// Creating an Optional with a non-null value
Optional<String> optionalName = Optional.of("John Doe");
// Creating an Optional that can be empty
Optional<String> emptyOptional = Optional.empty();
// Accessing the value
System.out.println(optionalName.get()); // prints "John Doe"
System.out.println(emptyOptional.isPresent()); // prints "false"
}
}
Why Use Optional Instead of Null?
- Improved Code Clarity: The
Optional
type clearly communicates that a value may be absent, leading to more readable code. - Eliminates NullPointerException: No more need for multiple null checks.
- Encourages Functional Programming:
Optional
integrates seamlessly with lambda expressions, enhancing the functional approach to programming.
Creating an Optional
You can create an Optional
in several ways:
Optional.of(value)
: Wraps a non-null value.Optional.ofNullable(value)
: Wraps a value that may be null.Optional.empty()
: Creates an emptyOptional
.
Understanding Optional.ofNullable
Consider the following example that demonstrates how Optional.ofNullable
can handle null values:
public class NullableExample {
public static void main(String[] args) {
String name = null;
Optional<String> optionalName = Optional.ofNullable(name);
// Safely access the value
optionalName.ifPresent(n -> System.out.println("Name is: " + n));
// Prints nothing as name is null
}
}
In this code snippet, ifPresent
is a method provided by Optional
that only executes the provided lambda if the value is present.
Accessing Optional Values
While Optional
helps avoid null checks, you still need a way to safely access the value it contains. Here are some common methods:
1. get()
This method retrieves the value contained in the Optional
. However, if the Optional
is empty, it will throw a NoSuchElementException
.
String value = optionalName.get(); // Unsafe if optionalName is empty!
2. orElse()
This method retrieves the value if present, otherwise returns a default value. It provides a safe way to handle absent values.
String name = optionalName.orElse("Default Name");
System.out.println(name); // Prints "Default Name" if optionalName is empty
3. orElseGet()
Like orElse
, but takes a supplier functional interface, allowing you to delay the evaluation of the default value.
String name = optionalName.orElseGet(() -> "Generated Name");
4. orElseThrow()
This method allows you to throw a custom exception if the value is absent.
String name = optionalName.orElseThrow(() -> new RuntimeException("Name is not present"));
Chaining Optional
Optional
supports a functional approach that allows you to chain method calls, leading to cleaner and more expressive code.
Example of Chaining with map and flatMap
When you want to transform the value of an Optional
, you can use the map
method:
Optional<String> optionalName = Optional.of("John Doe");
// Transform the value to its length
Optional<Integer> length = optionalName.map(String::length);
System.out.println(length.orElse(0)); // prints 8
If the operation might return another Optional
, use flatMap
to avoid nesting:
Optional<Optional<String>> nestedOptional = Optional.of(Optional.of("Nested"));
Optional<String> flatOptional = nestedOptional.flatMap(Optional::stream).findFirst();
Handling Edge Cases
1. Performance Concerns
While Optional
is a powerful tool, using it as a method parameter can have performance drawbacks. For example:
public void process(Optional<String> name) {
// code
}
Prefer avoiding Optional
as method parameters and use it for return types instead.
2. Serialization Issues
Using Optional
in a serialization context can lead to issues. Many serialization frameworks (like Jackson) don't support Optional
out of the box. Always ensure you handle serialization appropriately.
3. Legacy Code Compatibility
When integrating Optional
into legacy codebases, be cautious. Mixed usage of null
and Optional
can lead to confusion. Make a conscious decision to migrate fully or use Optional
judiciously.
Lessons Learned
Mastering Optional
in Java helps you write cleaner, more expressive code, avoiding the dreaded NullPointerException
. As you move forward, keep in mind the best practices discussed above, including creating Optional
instances appropriately, accessing values safely, and avoiding common pitfalls.
For further reading on Optional
and its best practices, check out the Java Documentation on Optional and explore more advanced use cases in your Java applications.
In the world of Java, navigating null
has become significantly easier with Optional
. Embrace it, and let it guide your quest for cleaner, bug-free code. Happy coding!
Checkout our other articles