Boosting Java Streams: Tackling Performance Pitfalls
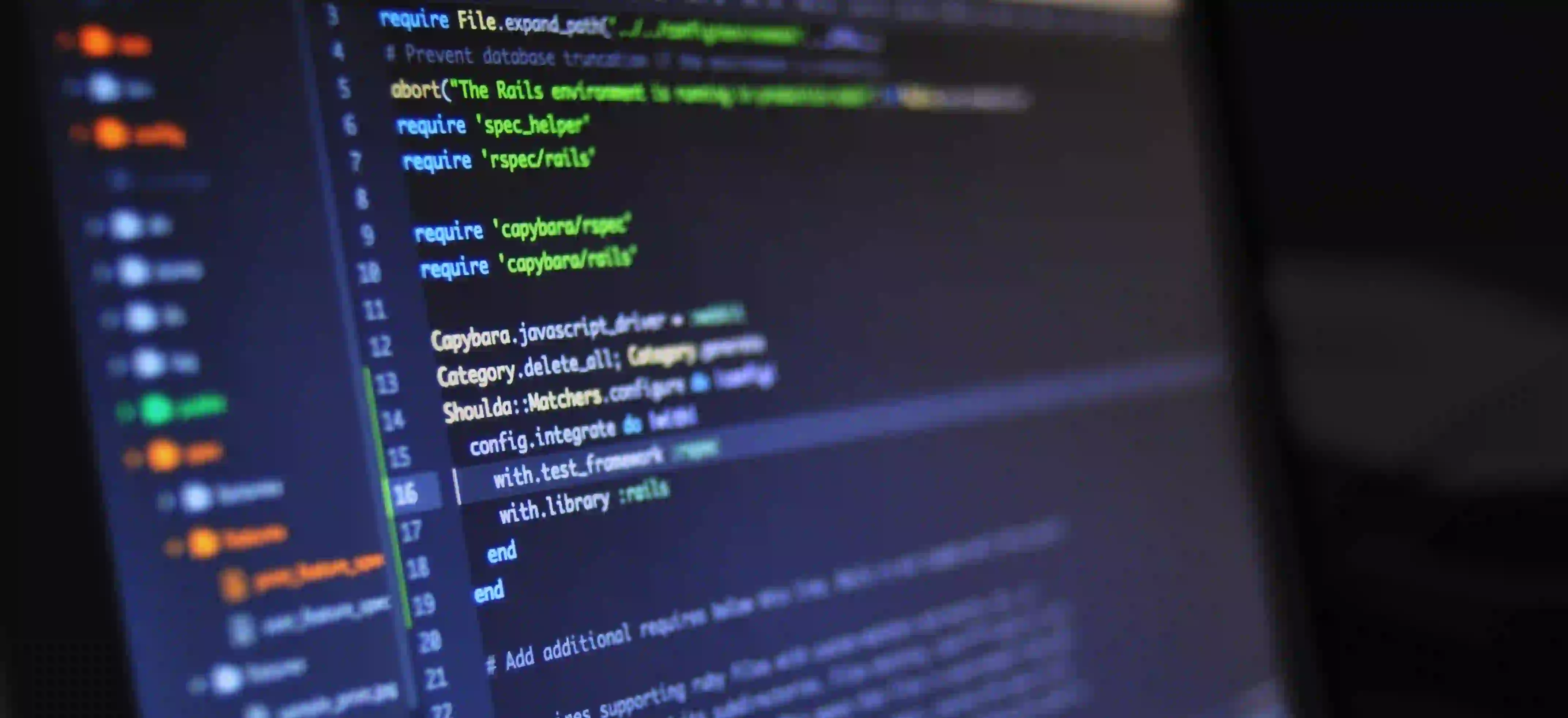
Boosting Java Streams: Tackling Performance Pitfalls
Java Streams, introduced in Java 8, revolutionized the way we work with collections by providing a powerful way to process sequences of elements. However, like any powerful tool, they come with their own set of performance pitfalls. In this guide, we will explore common performance issues developers face when working with Java Streams and how to overcome them. Through effective example code snippets, we will provide insights into improving performance while maintaining the readability that Streams offer.
Understanding Java Streams
Before delving into performance concerns, it's important to grasp what Streams are. A Stream represents a sequence of elements supporting sequential and parallel aggregate operations. Streams are not data structures; instead, they provide a view of data and can be generated from Collections, Arrays, I/O channels, or even generated values.
The primary operations on Streams can be categorized into:
- Intermediate operations (such as
filter
,map
, andsorted
) which return a new Stream. - Terminal operations (like
collect
,forEach
, andcount
) which produce a result and may traverse the Stream.
Key Benefits of Using Streams
- Conciseness: The ability to express complex operations in a single line of code.
- Parallel Processing: Built-in capabilities to run operations concurrently, leveraging multi-core processors.
- Lazy Evaluation: Streams evaluate elements as needed, which can lead to performance improvements.
Common Performance Pitfalls
While Java Streams can enhance productivity and code readability, improper use can lead to unexpected performance issues. Here are some prevalent pitfalls and how to tackle them.
1. Overhead of Stream Creation
Creating a Stream can incur overhead, especially if done in tight loops or repeatedly. Every time you create a Stream, a new state is instantiated, leading to potential performance degradation.
Example
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
List<String> names = List.of("Alice", "Bob", "Charlie");
// Ineffective creation of streams within a loop
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
Improvement
Instead, create the Stream once outside of loops or frequently called methods.
import java.util.List;
import java.util.stream.Collectors;
List<String> names = List.of("Alice", "Bob", "Charlie");
Stream<String> nameStream = names.stream();
List<String> filteredNames = nameStream.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
2. Not Using Short-Circuiting Operations
Not leveraging short-circuiting operations can lead to processing unnecessary elements, which can significantly impact performance, particularly in large datasets.
Example
import java.util.List;
List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6);
// This will process all elements, even after finding the first match
boolean anyMatch = numbers.stream()
.filter(n -> n > 4)
.findFirst()
.isPresent();
Improvement
Using the anyMatch
can short-circuit the operation when a condition is met, saving time.
import java.util.List;
List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6);
// Short-circuits after finding the first match
boolean anyMatch = numbers.stream()
.anyMatch(n -> n > 4);
3. Lack of Parallelism When Beneficial
Java Streams support parallel execution, which, when properly utilized, can lead to significant performance gains. This is particularly true for computations that are CPU-bound.
Example
import java.util.List;
List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6);
// Sequential processing
List<Integer> squaredNumbers = numbers.stream()
.map(n -> n * n)
.collect(Collectors.toList());
Improvement
When processing large datasets, consider using parallel streams. However, be cautious and measure the performance, as not all operations will benefit from parallelization due to the overhead involved.
import java.util.List;
List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6);
// Parallel processing
List<Integer> squaredNumbers = numbers.parallelStream()
.map(n -> n * n)
.collect(Collectors.toList());
4. Autoboxing Costs
Using primitive operations with Streams can incur autoboxing costs, which leads to decreased performance. Streams operate on objects, and converting primitives (e.g., int
, double
) to their corresponding wrapper classes (e.g., Integer
, Double
) can slow things down.
Example
import java.util.List;
import java.util.stream.Collectors;
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
// Autoboxing happens when using Stream with wrapper classes
List<Integer> squares = numbers.stream()
.map(n -> n * n)
.collect(Collectors.toList());
Improvement
Utilize IntStream
, LongStream
, or DoubleStream
to directly work with primitives.
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
// Use IntStream to avoid autoboxing costs
List<Integer> squares = IntStream.rangeClosed(1, 5)
.map(n -> n * n)
.boxed()
.collect(Collectors.toList());
5. Excessive Use of Intermediate Operations
Chaining multiple intermediate operations can lead to performance overhead, especially if not carefully designed. Each intermediate operation is lazy, but the more operations you have, the more complex the computation becomes.
Example
import java.util.List;
import java.util.stream.Collectors;
List<String> names = List.of("Alice", "Bob", "Charlie");
// Excessive intermediate operations
List<String> result = names.stream()
.filter(name -> name.length() > 3)
.map(String::toUpperCase)
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
Improvement
Try to combine operations where possible, or use a single pass to reduce the number of operations.
import java.util.List;
import java.util.stream.Collectors;
List<String> names = List.of("Alice", "Bob", "Charlie");
// Combining operations to reduce complexity
List<String> result = names.stream()
.filter(name -> name.length() > 3 && name.startsWith("A"))
.map(String::toUpperCase)
.collect(Collectors.toList());
Final Thoughts
Java Streams provide an elegant and efficient way to process collections but can lead to performance pitfalls if misused. By understanding the common challenges discussed in this guide and employing the suggested optimizations, you can harness the full potential of Java Streams.
Remember, the key to performance is thoughtful design and careful coding practices. As a best practice, always measure your changes; each application is unique, and performance optimizations should be validated against real-world use cases.
Further Reading
- Java Streams Tutorial
- Effective Java (3rd Edition) by Joshua Bloch
- Java Performance Tuning
By applying these strategies, you can significantly boost the performance of Java Streams in your applications. Happy coding!