Mastering Precision: Common Pitfalls with Java BigDecimal
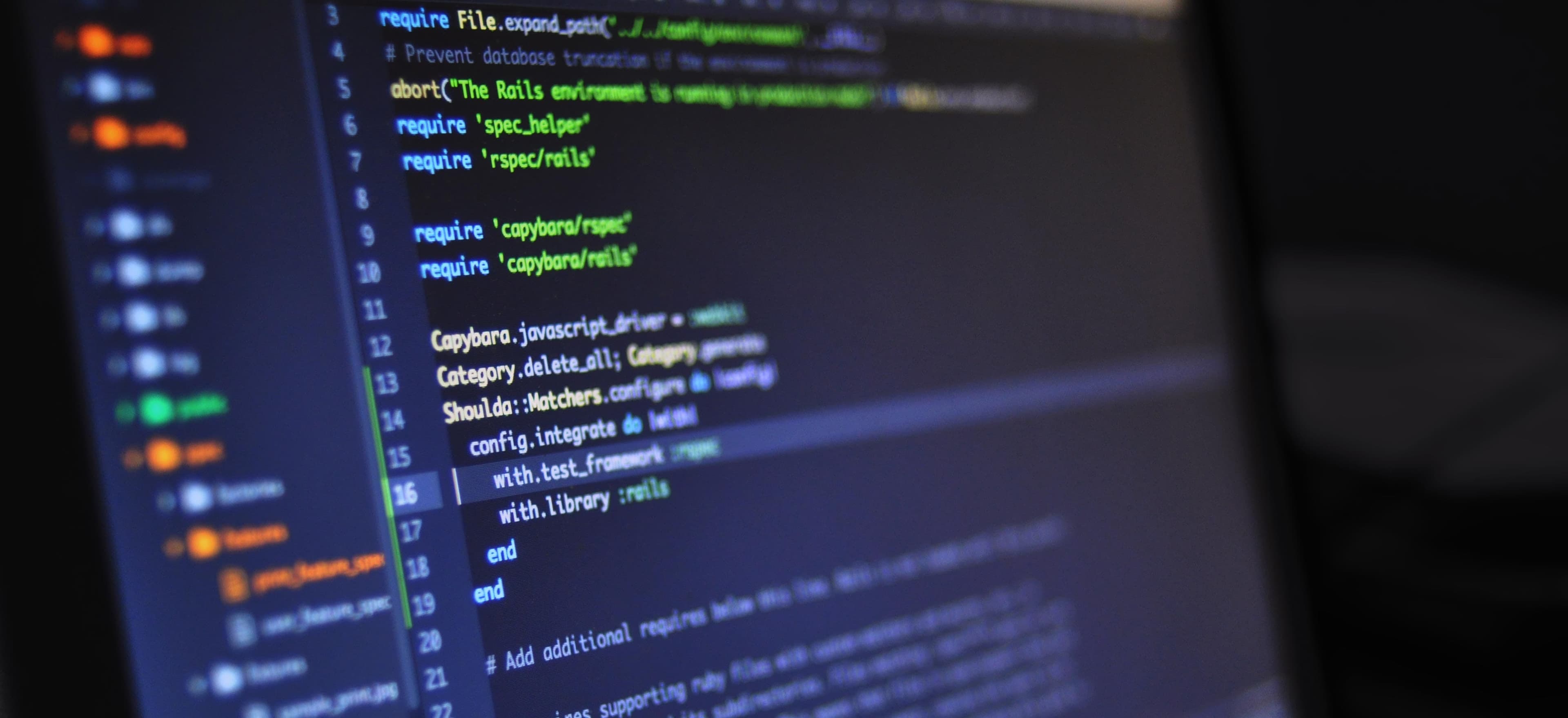
- Published on
Mastering Precision: Common Pitfalls with Java BigDecimal
Java provides a robust way to deal with numbers, especially those requiring high precision—such as currency. The BigDecimal
class is Java’s go-to solution for representing immutable, arbitrary-precision decimal numbers. While incredibly powerful, improper usage can lead to common pitfalls that developers face when working with monetary values. This blog post dives into these pitfalls and offers strategies to avoid them, ensuring that you master precision in your Java applications.
Understanding BigDecimal
Before we jump into the pitfalls, let’s briefly review what BigDecimal
is and why it’s preferred over primitive types like float and double.
The BigDecimal
class is part of the java.math
package and is a significant improvement over floating-point types for situations requiring exact decimal representation. This is crucial in applications like financial transactions, where precision is paramount.
For instance, creating a BigDecimal
from a string is straightforward:
import java.math.BigDecimal;
public class BigDecimalExample {
public static void main(String[] args) {
BigDecimal price = new BigDecimal("19.99");
System.out.println("Price: " + price);
}
}
In the example above, using a string to initialize BigDecimal
is critical to avoid precision loss, which can occur if we use floating-point literals.
Common Pitfalls with BigDecimal
1. Incorrect Initialization
As highlighted above, initializing a BigDecimal
with a double can lead to unexpected results:
BigDecimal value = new BigDecimal(0.1); // Not recommended
Why? The double representation of 0.1 isn't exact, which can lead to unexpected values, such as:
import java.math.BigDecimal;
public class IncorrectInitialization {
public static void main(String[] args) {
BigDecimal value = new BigDecimal(0.1);
System.out.println("Value: " + value); // Output might be 0.100000000000000005551115123125782707118
}
}
Solution: Always use the string constructor:
BigDecimal value = new BigDecimal("0.1");
2. Comparing BigDecimal Instances
When comparing two BigDecimal
instances, developers might incorrectly use the ==
operator, which checks for reference equality instead of value equality.
Instead of this:
BigDecimal a = new BigDecimal("0.1");
BigDecimal b = new BigDecimal("0.1");
if (a == b) {
System.out.println("They're equal.");
}
Do this:
if (a.compareTo(b) == 0) {
System.out.println("They're equal.");
}
Why? The compareTo
method allows comparison based on value, returning:
- 0 if they are equal,
- -1 if this is less than the other instance, and
- 1 if this is greater.
3. Improper Arithmetic Operations
Often, users fail to specify the scale and rounding mode when performing arithmetic operations.
BigDecimal price = new BigDecimal("10.00");
BigDecimal tax = new BigDecimal("0.07");
BigDecimal total = price.add(tax); // Might not give expected results
Solution: Always define a scale and rounding mode:
BigDecimal total = price.add(tax).setScale(2, BigDecimal.ROUND_HALF_UP);
4. Forgetting to Set Scale in Division
Another common mistake is encountering ArithmeticException
due to a non-terminating division. For example:
BigDecimal a = new BigDecimal("1");
BigDecimal b = new BigDecimal("3");
BigDecimal c = a.divide(b); // Throws an exception
Solution: Always define a scale and rounding mode when dividing:
BigDecimal c = a.divide(b, 2, BigDecimal.ROUND_HALF_UP); // Gives 0.33
5. Misusing Scale
The scale
of a BigDecimal
refers to the number of digits to the right of the decimal point. Mismanaging the scale leads to significant rounding errors.
For instance:
BigDecimal amount = new BigDecimal("7.123456");
BigDecimal scaledAmount = amount.setScale(2, BigDecimal.ROUND_HALF_UP);
System.out.println("Scaled Amount: " + scaledAmount); // Outputs 7.12
Why? Understanding the significance of scale helps maintain accuracy. Always ensure you know the scale required for your specific use case.
6. Ignoring the Immutable Nature
One crucial aspect of BigDecimal
is that it is immutable. This means that every arithmetic operation returns a new instance rather than modifying the existing instance.
Consider the following:
BigDecimal value = new BigDecimal("22.0");
value.add(new BigDecimal("5.0")); // This does nothing to `value`
System.out.println(value); // Output: 22.0
Solution: Always capture the result:
value = value.add(new BigDecimal("5.0")); // Now `value` correctly updates
7. Neglecting Thread Safety
While BigDecimal
is immutable, its usage in multi-threaded settings requires careful handling. If one thread modifies the state of a containing object, it may lead to unexpected behavior when another thread accesses it.
Best Practice: Consider using synchronization mechanisms or concurrent data structures to prevent inconsistencies when BigDecimal
is part of a shared resource.
In Conclusion, Here is What Matters
While the BigDecimal
class offers an essential solution for precise decimal arithmetic in Java, its misuse can lead to significant pitfalls. By following best practices for initialization, comparison, arithmetic operations, and understanding the implications of scale and immutability, you can master precision in your Java applications.
For further learning, explore the Java documentation on BigDecimal or take a deeper dive into related topics such as Numerical Precision in Java.
Explore More
If you found this post helpful, consider checking out:
Happy coding!
Checkout our other articles