Overcoming Java ForkJoinPool's Resource Exhaustion Issues
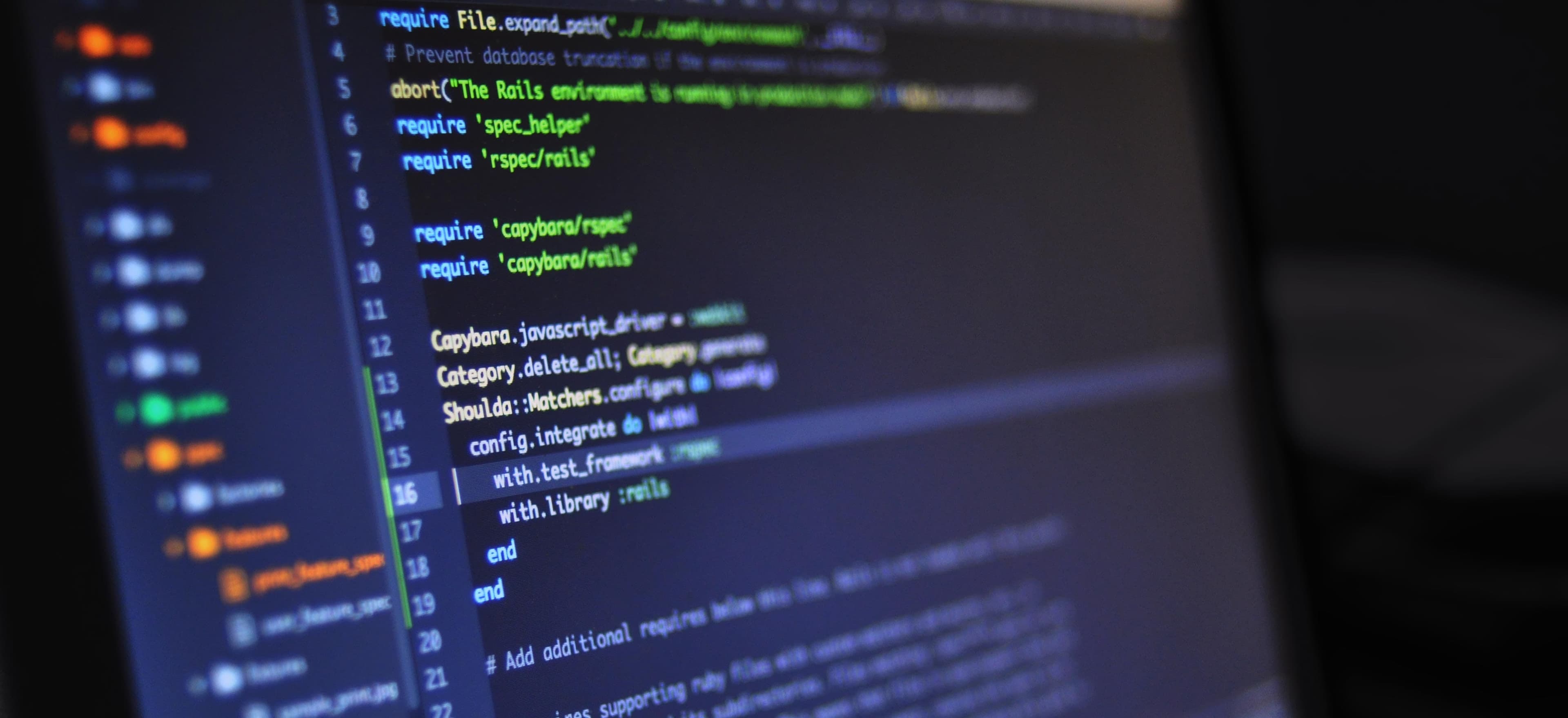
- Published on
Overcoming Java ForkJoinPool's Resource Exhaustion Issues
Java's concurrency framework has made significant strides in providing developers robust tools for effective multi-threading. Among these tools, the ForkJoinPool
stands out for its ability to efficiently manage a pool of worker threads designed to work on large-scale, parallelizable tasks. This post delves into the challenges of resource exhaustion in the ForkJoinPool
and how to overcome them.
Understanding ForkJoinPool
The ForkJoinPool
framework is part of the java.util.concurrent package and facilitates parallel processing by utilizing a work-stealing algorithm. The pool consists of worker threads that can execute tasks of varying complexity and depth concurrently, which enhances performance in suitable scenarios.
Key Features
- Work-Stealing Algorithm: Idle threads can steal work from other busy threads, thus enhancing efficiency.
- Recursive Task Execution: Particularly well-suited for divide-and-conquer algorithms, where tasks can be split into subtasks recursively.
A Simple Example
Consider a simple program that sums an array of integers using ForkJoinPool
.
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class SumArray extends RecursiveTask<Integer> {
private static final int THRESHOLD = 10;
private final int[] array;
private final int start;
private final int end;
public SumArray(int[] array, int start, int end) {
this.array = array;
this.start = start;
this.end = end;
}
@Override
protected Integer compute() {
if (end - start <= THRESHOLD) {
int sum = 0;
for (int i = start; i < end; i++) {
sum += array[i];
}
return sum;
}
int mid = (start + end) / 2;
SumArray leftTask = new SumArray(array, start, mid);
SumArray rightTask = new SumArray(array, mid, end);
leftTask.fork(); // asynchronously execute left task
int rightResult = rightTask.compute(); // execute right task
int leftResult = leftTask.join(); // join left task
return leftResult + rightResult;
}
public static void main(String[] args) {
int[] array = new int[100];
for(int i = 0; i < array.length; i++) {
array[i] = i + 1;
}
ForkJoinPool pool = new ForkJoinPool();
SumArray task = new SumArray(array, 0, array.length);
int result = pool.invoke(task);
System.out.println("Sum is: " + result);
}
}
In the example above, we create a recursive task to sum segments of an integer array. The compute
method splits the array until it reaches a manageable size specified by THRESHOLD
.
ForkJoinPool Resource Exhaustion Issues
Despite its advantages, the ForkJoinPool
is not without caveats. Resource exhaustion becomes a pressing concern under heavy loads. Here’s what you need to keep in mind:
-
Thread Limits: By default, the number of threads in a
ForkJoinPool
is the number of available processors, which may not be sufficient in all scenarios. -
Blocking Calls: If a task makes blocking calls, threads may remain idle, leading to overall slowdown and reduced throughput.
-
Task Queue Overload: Excessively deep recursion may lead to the task queue getting overwhelmed, causing increased contention and potential starvation of resources.
Signs of Resource Exhaustion
- High CPU usage with slow performance
- Increased latency on task execution
- Threads frequently being in a blocked state
- Out-of-memory errors when allocating new task objects
Strategies to Overcome Resource Exhaustion
1. Adjust Pool Size
You can modify the ForkJoinPool
size when creating the instance. Increasing the parallelism level can suit your workload better. For example:
ForkJoinPool pool = new ForkJoinPool(16); // create a pool with 16 threads
For optimal performance, adapt the number of threads based on the hardware environment and the nature of the tasks.
2. Avoid Blocking Calls
In parallel processing, blocking calls can significantly diminish the advantages of concurrency. Instead of using blocking I/O, consider using non-blocking I/O approaches or asynchronous programming techniques. Here's a small snippet demonstrating how to handle futures, which can aid non-blocking programming:
import java.util.concurrent.*;
public class NonBlockingExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(4);
CompletableFuture<Void> future = CompletableFuture.supplyAsync(() -> {
// simulate task
return "Result";
}, executor).thenAccept(result -> {
System.out.println("Processed: " + result);
});
future.join(); // wait for completion
executor.shutdown();
}
}
3. Divide and Conquer Tactically
When designing your tasks, keep them minimal and manageable. Use a recursive approach only when necessary, and ensure that tasks do not create excessive depth.
4. Handle Suboptimal Scenarios Gracefully
If tasks face blocking conditions, make use of ForkJoinPool
's mechanism to handle exceptions and manage task completion gracefully. Leverage the ForkJoinTask
’s exceptionally
method to handle errors without crashing the entire pool.
Example:
@Override
protected Integer compute() {
try {
// computation logic
} catch(Exception e) {
// handle exception
return 0; // or some value indicating failure
}
}
5. Resource Monitoring
Utilize Java's built-in management tools such as JMX, VisualVM, or any APM (Application Performance Monitoring) tool to monitor thread utilization, memory consumption, and CPU usage patterns. Observing resource metrics can help you make informed adjustments.
In Conclusion, Here is What Matters
The ForkJoinPool
is a powerful tool for concurrent processing in Java. However, wrongful implementation and resource management can lead to exhaustion challenges. By understanding the intricacies of thread management and applying best practices—such as optimizing pool sizes, avoiding blocking calls, dividing tasks intelligently, and monitoring resources—you can effectively mitigate these issues.
For further reading on concurrency and Java, consider the following resources:
- Java Concurrency in Practice (Book)
- Java 8 in Action (Book)
Ultimately, mastering ForkJoinPool
can greatly improve your application’s performance and responsiveness, enabling you to handle even the most demanding tasks efficiently. Enjoy coding!