Mastering Exception Handling in Java Lambda Expressions
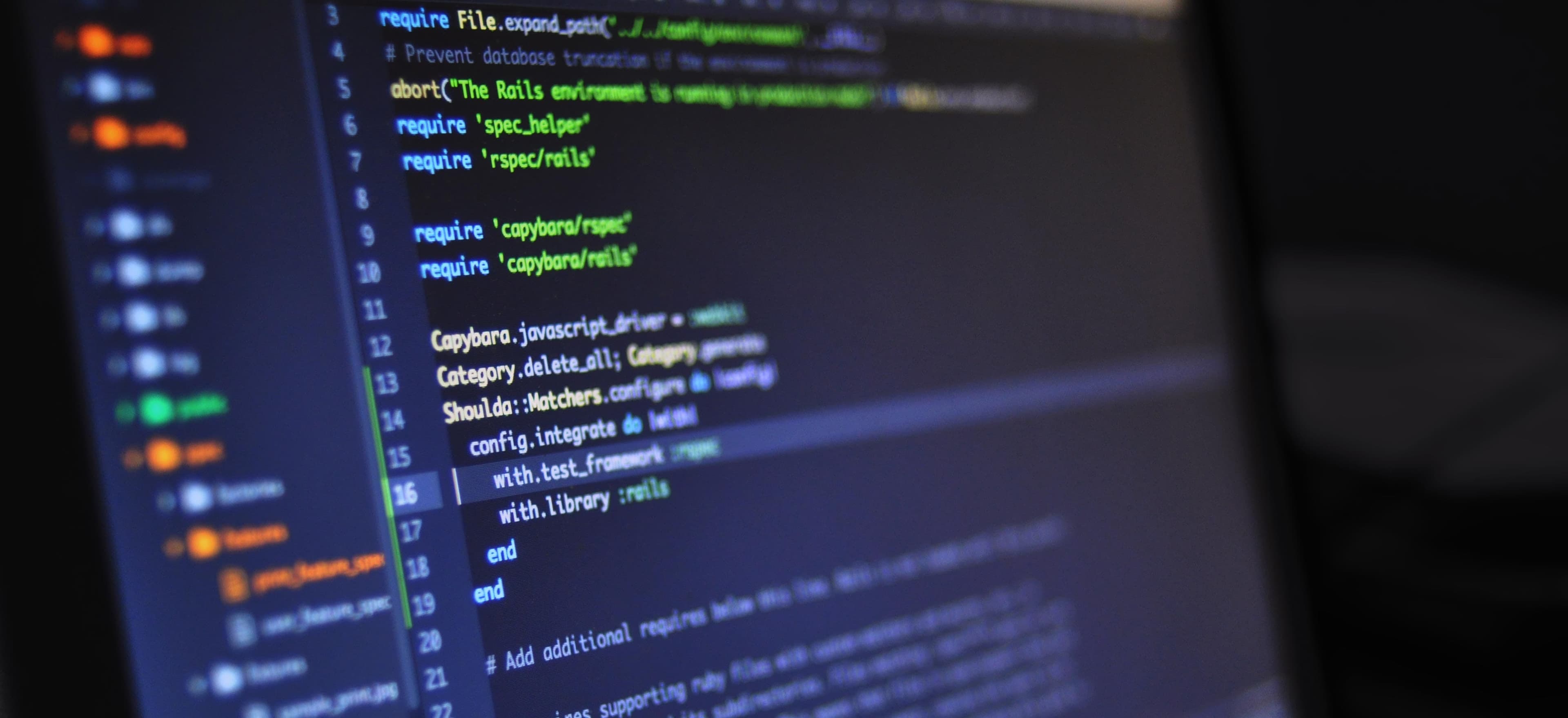
- Published on
Mastering Exception Handling in Java Lambda Expressions
With the rise of Java 8, lambda expressions have transformed the way developers write Java code. They offer a concise and functional approach to programming, promoting cleaner code and enhancing productivity. However, one challenge that comes with these elegant syntax forms is the effective handling of exceptions. In this blog post, we will delve deep into how to manage exceptions in Java's lambda expressions, ensuring that you write robust code while retaining the beauty of lambda functionality.
What are Lambda Expressions?
Before we dive into exception handling, let's quickly recap what lambda expressions are.
Lambda expressions provide a way to implement functional interfaces, which are interfaces with a single abstract method. The syntax can be summarized as follows:
(parameters) -> expression
Here's a simple example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
In this example, the forEach
method takes a lambda expression that prints each name in the list. While this is straightforward, handling errors within these expressions can be tricky.
Understanding the Exception Dilemma
In traditional Java code, exception handling is straightforward using try-catch blocks. With lambda expressions, however, exceptions are not handled automatically. If an exception occurs in a lambda expression, it is thrown back to its caller. This is where things get a bit complicated, especially when working with functional interfaces that do not declare checked exceptions.
For example:
Runnable runnable = () -> {
throw new Exception("Checked Exception");
};
This code will fail to compile because Runnable
does not declare any checked exceptions.
Strategies for Exception Handling in Lambda Expressions
1. Wrapping Checked Exceptions
One common strategy to handle exceptions in lambda expressions is to wrap checked exceptions in unchecked exceptions (if feasible). This allows you to use lambda expressions without worrying about the checked exceptions.
public static void executeWithExceptionHandling(Runnable runnable) {
try {
runnable.run();
} catch (RuntimeException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
Now, you can create a lambda expression using this method:
executeWithExceptionHandling(() -> {
throw new RuntimeException("Runtime Exception thrown");
});
2. Use of Custom Functional Interfaces
Another approach is to create your own functional interfaces that declare checked exceptions. This allows you to throw and catch exceptions more naturally.
@FunctionalInterface
interface CheckedRunnable {
void run() throws Exception;
}
public static void executeWithCustomInterface(CheckedRunnable runnable) {
try {
runnable.run();
} catch (Exception e) {
System.out.println("Caught checked exception: " + e.getMessage());
}
}
Now you can use this interface with lambda expressions:
executeWithCustomInterface(() -> {
throw new Exception("This is a checked exception");
});
3. Using Utility Methods
You can create utility methods that handle exceptions for you, which can significantly reduce boilerplate code across your application. This involves a pattern where lambda expression calls are made through a utility method that handles exceptions.
Here's an example:
public class ExceptionUtil {
public static void wrapUnchecked(CheckedRunnable action) {
try {
action.run();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
Now you can simply use:
ExceptionUtil.wrapUnchecked(() -> {
// Simulating a checked exception
throw new Exception("Checked Exception occurred.");
});
4. Functional Programming Libraries
Various third-party libraries such as Vavr provide additional capabilities to work with functional interfaces and exception handling. Vavr supports handling exceptions more gracefully, allowing you to return either successfully or with a failure encapsulated in a result type.
Here's how you can use Vavr:
import io.vavr.control.Try;
Try<String> result = Try.of(() -> riskyOperation());
result.onFailure(ex -> System.out.println("Error occurred: " + ex.getMessage()));
result.onSuccess(resultValue -> System.out.println("Operation successful: " + resultValue));
This approach allows you to chain various operations conditioned on success or failure.
Best Practices
While dealing with exceptions in lambda expressions, consider the following best practices:
- Prefer Unchecked Exceptions: If possible, convert checked exceptions to unchecked ones to leverage the simplicity of lambda expressions.
- Use Custom Interfaces: Create your own functional interfaces when needed, allowing you to declare exceptions directly.
- Centralize Exception Handling: Use utility methods to handle exceptions uniformly across your application.
- Leverage Libraries: Consider using libraries like Vavr for expanded functionalities that make functional programming smoother in Java.
Summary
Exception handling in lambda expressions can be complex, but by utilizing the strategies mentioned above, you can write clean, efficient, and reliable code. Whether it is wrapping checked exceptions, using custom functional interfaces, or leveraging third-party libraries, you have multiple avenues to ensure robust exception management.
Incorporating these best practices into your Java programming will not only streamline your error handling procedures but also enhance the overall quality of your code.
For further reading on Java's functional programming features, consider checking out Oracle's Java Documentation and the Vavr Documentation.
Now go ahead and embrace the power of lambda expressions in your Java projects, while keeping exception handling in check! Happy coding!