Boosting Java String Concatenation: Avoiding Performance Pitfalls
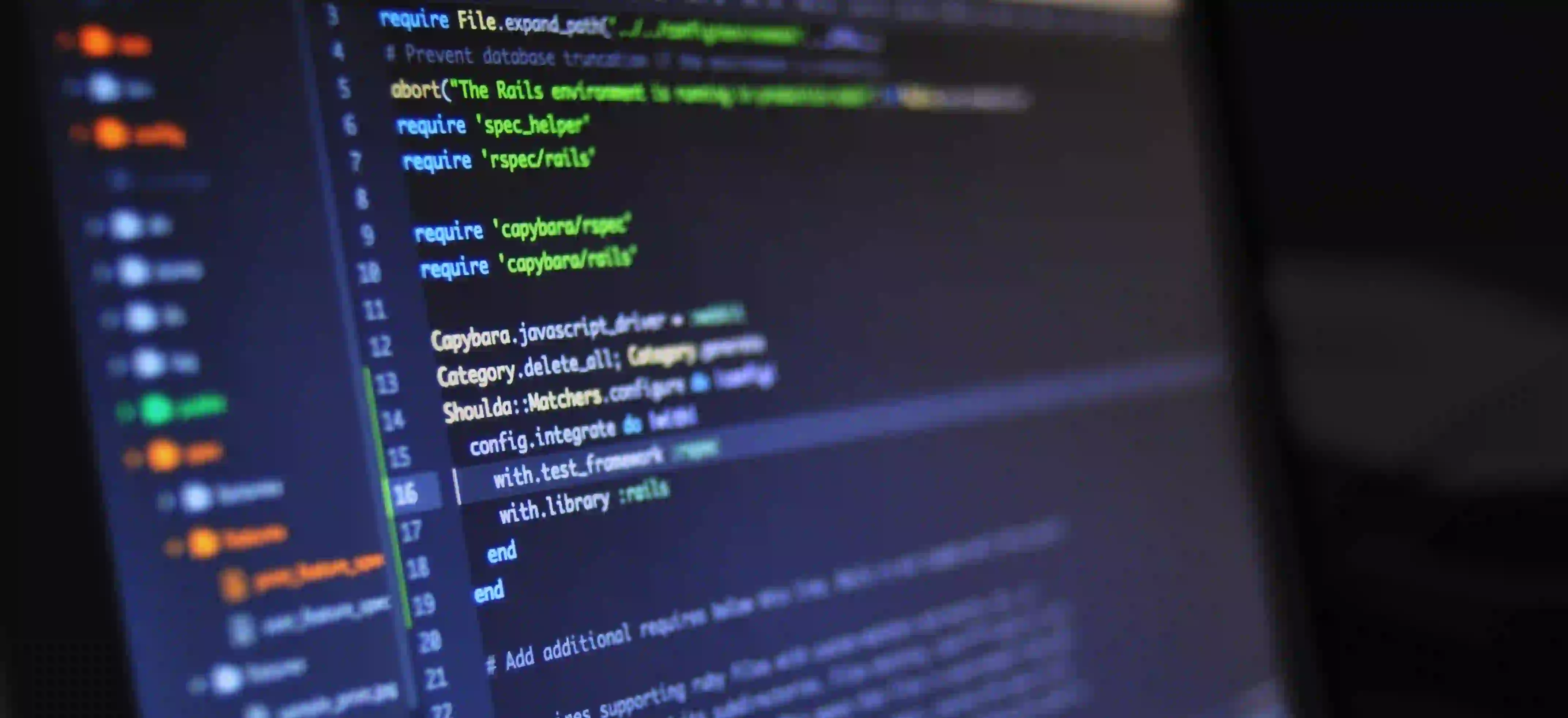
Boosting Java String Concatenation: Avoiding Performance Pitfalls
String concatenation in Java is a common yet often misunderstood aspect of programming. Many developers use the +
operator or String.concat()
method without recognizing the underlying implications on performance. If not handled thoughtfully, string concatenation can lead to inefficient code. In this blog post, we will delve into various techniques for concatenating strings in Java, highlight performance nuances, and discuss best practices.
Understanding String Immutability
Before diving into performance, it's essential to understand that in Java, strings are immutable. This means that once a String
object is created, it cannot be changed. Each time a string is manipulated (e.g., concatenated), a new string object is created in memory and the original remains unchanged. This characteristic is one of the primary reasons why improper concatenation can lead to poor performance.
Example: Simple Concatenation
Here's a straightforward example of string concatenation using the +
operator:
String str1 = "Hello";
String str2 = "World";
String result = str1 + " " + str2; // "Hello World"
In the above scenario, every concatenation creates a new String
object, leading to unnecessary memory consumption and increased processing time.
Performance Implications of Concatenation
Using the +
operator for concatenating strings in a loop is particularly detrimental. Let’s analyze a simple loop that concatenates strings:
String result = "";
for (int i = 0; i < 1000; i++) {
result += i;
}
In this case, the process involves creating multiple intermediate String
objects. The result? Performance degradation and excessive memory overhead.
Why This Matters
When you concatenate strings multiple times in a loop, you create a string reshaping problem where each new String
requires the entire previous string plus the new addition. This can quickly exhaust memory resources and lead to OutOfMemoryError
.
What Should You Use Instead?
StringBuilder: The Efficient Alternative
The most efficient way to perform string concatenation in Java is to use StringBuilder
. This mutable class allows you to modify string contents without creating new objects repeatedly.
Example: Using StringBuilder
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append(i); // Mutable and efficient
}
String result = sb.toString(); // Convert to String at the end
Why Use StringBuilder?
- Memory Efficiency:
StringBuilder
allocates memory in chunks, reducing the overhead of creating newString
objects. - Performance Gain: Append operations are significantly faster, especially in loops, since there’s no need to create and discard intermediary strings.
When to Use StringBuffer?
It may be tempting to use StringBuffer
, which is another mutable class like StringBuilder
. However, StringBuffer
is synchronized, making it thread-safe but slower under most circumstances. Choose StringBuilder
unless your application specifically requires thread safety.
Alternative Methods
While using StringBuilder
is generally the best option, there are a few other ways to concatenate strings that are worth mentioning:
Using String.join() Method
For simple cases where you need to join multiple strings, String.join()
can come in handy:
String[] words = {"Hello", "World"};
String result = String.join(" ", words); // "Hello World"
Why Use String.join()?
- Clarity: This method immediately conveys the intention behind your code.
- Convenience: It handles multiple strings elegantly, without needing to write custom loops.
Java 8 Stream API
If you're using Java 8 or later, you can also use the Stream API for concatenation:
List<String> list = Arrays.asList("Hello", "World");
String result = list.stream()
.collect(Collectors.joining(" ")); // "Hello World"
Why This Matters
- Functional Approach: It promotes functional programming principles, improving code readability.
- Enhancements: The ability to apply filters and transformations while concatenating.
Best Practices for String Concatenation
- Use StringBuilder: Opt for
StringBuilder
for most string concatenation needs, especially in loops. - Be Cautious with + Operator: Avoid it in performance-sensitive contexts, such as within loops or in tight performance constraints.
- Consider Immutability: Evaluate the necessity of creating new strings, especially in scenarios with high volume data processing.
- Profile Your Code: Always test and profile your code under realistic conditions to gauge performance changes caused by string manipulations.
Closing the Chapter
String concatenation, while simple, can lead to significant performance pitfalls in Java if handled carelessly. By understanding string immutability and adopting efficient practices like using StringBuilder
, developers can avoid unnecessary memory overhead and ensure that their applications run smoothly.
For more insights on Java performance, consider exploring resources from the official Java Documentation and Oracle's Java Tutorials.
Additional Reading
- Performance Benefits of Using StringBuilder
- Effective Java: Best Practices for Avoiding Performance Pitfalls
With these strategies in mind, you can take control of your Java string manipulations and enhance your application's efficiency significantly!