Conquering Java OOM Errors: Taming Memory Leaks
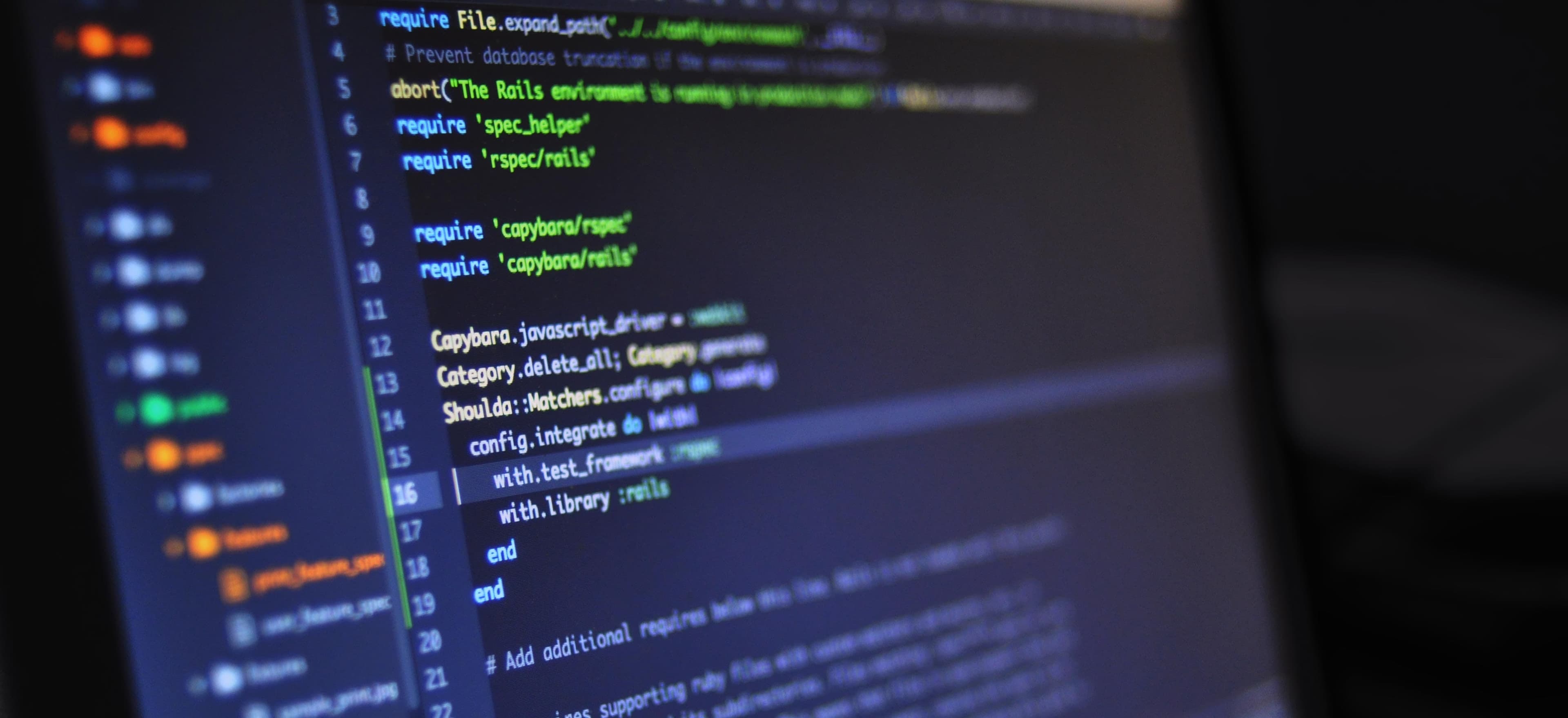
- Published on
Conquering Java OOM Errors: Taming Memory Leaks
In the world of Java programming, encountering an OutOfMemoryError (OOM) can be a frustrating experience. These errors indicate that the Java Virtual Machine (JVM) has run out of memory, causing applications to crash or misbehave. In this blog post, we will discuss memory leaks, identify their potential causes, and offer strategies to combat OOM errors effectively.
Understanding OutOfMemoryError
Before we move on to memory leaks, it's essential to understand what OOM errors are. The JVM manages memory allocation for Java applications. When an application attempts to use more memory than allocated—whether due to memory leaks, heavy object creation, or inadequate JVM configurations—it throws an OOM error.
Key forms of OutOfMemoryError include:
- Java heap space: Insufficient space in the Java heap.
- Metaspace: Direct memory allocated for classes and methods.
- GC overhead limit exceeded: The garbage collector is taking too long to recover memory.
Why Memory Leaks Occur
Memory leaks occur when objects are no longer needed but still referenced. These references prevent the garbage collector from reclaiming memory, resulting in increased memory consumption over time. Common causes of memory leaks in Java include:
- Static fields: Holding references to objects that are no longer in use.
- Listeners and callbacks: Failing to unregister listeners or callbacks can retain references.
- Inner classes: Non-static inner classes automatically hold a reference to their containing class.
It’s important to identify and mitigate these issues proactively. But first, let’s see how to diagnose the problem.
Diagnosing Memory Leaks
To effectively address memory leaks, you must first identify and track them down. Here’s how you can accomplish this:
1. Monitoring Memory Usage
Use Java tools like JVisualVM or JConsole to monitor your application’s memory usage in real time. These tools are bundled with the JDK:
jvisualvm
These applications provide insights into heap memory usage, allowing you to visualize and analyze memory consumption.
2. Profiling the Application
Java profiling tools, such as YourKit or Eclipse Memory Analyzer (MAT), can help identify potential memory leaks. For example, MAT allows you to analyze heap dumps to determine which objects are consuming most of the memory.
Heap dumps can be generated using the following command:
jmap -dump:live,format=b,file=heap_dump.hprof <PID>
Replace <PID>
with the process ID of your Java application.
Strategies for Managing Memory
1. Avoid Unnecessary Object Retention
It’s crucial to ensure that references to objects that are no longer needed are released. Here’s an example:
class Example {
private List<String> strings = new ArrayList<>();
public void addString(String str) {
strings.add(str);
}
public void clearStrings() {
strings.clear(); // Release references
}
}
In this example, clearStrings()
method releases references in the list, allowing the garbage collector to reclaim memory.
2. Utilize Weak References
Java provides the WeakReference
and WeakHashMap
classes to create objects that can be garbage collected more easily. Consider using them when appropriate:
import java.lang.ref.WeakReference;
public class WeakReferenceExample {
private WeakReference<Object> weakRef;
public void createWeakReference(Object obj) {
weakRef = new WeakReference<>(obj);
}
public void useWeakReference() {
Object obj = weakRef.get(); // Will return null if object is collected
if (obj != null) {
// Use the object
} else {
// The object has been collected
}
}
}
Weak references can significantly help reduce memory consumption in applications that handle large datasets or caches.
3. Properly Implement Listeners and Callbacks
Always ensure that you unregister listeners and callbacks when they are no longer needed:
class EventSource {
private List<Listener> listeners = new ArrayList<>();
public void addListener(Listener listener) {
listeners.add(listener);
}
public void removeListener(Listener listener) {
listeners.remove(listener); // Important to prevent memory leaks
}
public void notifyListeners() {
for (Listener listener : listeners) {
listener.update();
}
}
}
By removing listeners when they are no longer relevant or needed, you can avoid retaining unnecessary references.
4. Optimize JVM Memory Settings
Sometimes, you can improve your application's memory usage by tuning the JVM. This can be done using several options:
-Xms
sets the initial heap size.-Xmx
sets the maximum heap size.-XX:MaxMetaspaceSize
controls the maximum size of metaspace.
Example JVM arguments:
java -Xms512m -Xmx2048m -XX:MaxMetaspaceSize=512m -cp yourApp.jar your.main.Class
Ensure that your settings align with your application’s needs.
A Final Look
Memory management in Java is a crucial skill for developers seeking to optimize application performance and reliability. OutOfMemoryErrors can cripple applications and result in poor user experience. However, by understanding the root causes of memory leaks and employing various strategies—such as managing references, using weak references, and optimizing JVM settings—you can effectively combat these issues.
For further reading on memory management in Java, consider exploring the Java Documentation and Java Concurrency in Practice.
By becoming adept at identifying and resolving memory issues, you will not only enhance your own programming proficiency but also ensure that your applications remain robust and efficient.
Happy coding!
Checkout our other articles