Decoding Java's Happen-Before: Avoiding Common Pitfalls
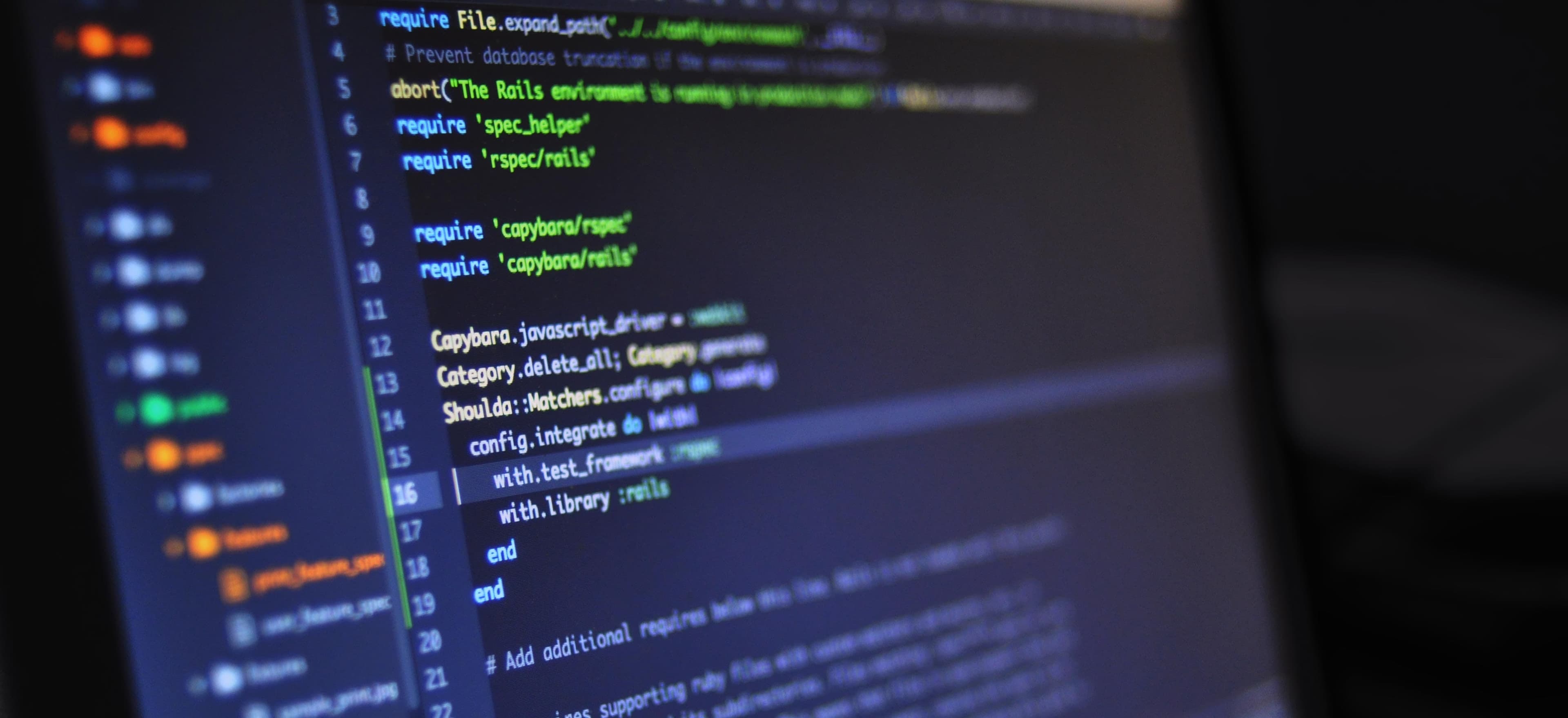
- Published on
Decoding Java's Happen-Before: Avoiding Common Pitfalls
Java’s memory model is one of the core foundations of building robust multi-threaded applications. The concept of “happen-before” relationships in Java is crucial to ensuring a program's correctness. In this blog post, we will decode the happen-before principle, how it affects your Java applications, and common pitfalls to avoid.
Understanding Happen-Before Relationships
The term "happen-before" denotes a set of rules that regulate the order of operations within multi-threaded environments. This principle states that if one event happens-before another, the first event is visible to the second, and therefore, it can be safely assumed that the outcomes of the first event will precede that of the second.
Key Happen-Before Rules
- Program Order Rule: Statements within a single thread occur in the order they appear in the program.
- Monitor Lock Rule: A call to
lock
on a monitor happens-before subsequent calls tounlock
on the same monitor. - Volatile Variable Rule: A write to a
volatile
variable happens-before every subsequent read of that variable by another thread. - Thread Start/Join: A thread that successfully starts another thread happens-before any actions in the started thread. Similarly, when one thread successfully joins another, all actions in the joined thread happen-before the join returns.
Importance of Happen-Before in Java
Understanding happen-before relationships is a matter of communication in a concurrent environment. It ensures visibility and ordering of shared data across threads, which is essential for avoiding concurrency issues such as race conditions.
Common Pitfalls to Avoid
1. Ignoring Volatile Variables
Many developers underutilize volatile
variables due to misconceptions about their performance or thread visibility characteristics.
public class Counter {
private volatile int count = 0;
public void increment() {
count++; // This is not atomic!
}
public int getCount() {
return count;
}
}
In the example above, while count
is marked as volatile
, the operation count++
is not atomic, meaning two threads could read and increment it simultaneously, leading to incorrect counts.
Why? The increment operation involves three steps: reading the value, incrementing, and writing it back. To secure this increment operation, consider using AtomicInteger
instead:
import java.util.concurrent.atomic.AtomicInteger;
public class SafeCounter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet(); // Atomic operation!
}
public int getCount() {
return count.get();
}
}
2. Misusing Synchronized Blocks
Synchronized methods work under the hood using monitors. However, developers often misuse them by locking too much code or locking on the wrong object.
public synchronized void unsafeMethod() {
// do some work
}
Why? This locks the entire unsafeMethod
, potentially leading to performance bottlenecks.
Instead, limit the synchronization to only shared mutable state:
private final Object lock = new Object();
public void safeMethod() {
synchronized (lock) {
// Only critical section code here
}
}
3. Forgetting Thread Order
Thread interleaving can lead to unexpected behavior, where the execution order impacts how soon a thread can see changes made by another thread.
public class SharedData {
private int data = 0;
public void writer() {
data = 1;
// No happen-before relationship established to the reader
}
public void reader() {
int temp = data; // Might see an outdated value!
}
}
Why? Without proper synchronization, reader
might see a stale version of data
. Use volatile
or synchronization mechanisms to ensure visibility:
private volatile int data = 0;
public void writer() {
data = 1;
}
public void reader() {
int temp = data; // Now sees updated value due to volatile
}
Leveraging Java Concurrency Utilities
Java provides several concurrent collections and utility classes in the java.util.concurrent
package that enforce happen-before relationships. For example:
- ConcurrentHashMap
- CountDownLatch
- CyclicBarrier
These classes manage the complexities of synchronization and visibility automatically.
Example of Using CountDownLatch
Here's a simple demonstration of how to use CountDownLatch
:
import java.util.concurrent.CountDownLatch;
public class Example {
public static void main(String[] args) throws InterruptedException {
CountDownLatch latch = new CountDownLatch(3);
for (int i = 0; i < 3; i++) {
new Thread(new Task(latch)).start();
}
// Wait until all threads finish
latch.await();
System.out.println("All tasks finished!");
}
}
class Task implements Runnable {
private final CountDownLatch latch;
public Task(CountDownLatch latch) {
this.latch = latch;
}
@Override
public void run() {
// Task execution
System.out.println(Thread.currentThread().getName() + " is working.");
latch.countDown(); // Decrementing the latch
}
}
Why? Using CountDownLatch
ensures you can coordinate multiple threads and establish a clear happens-before relationship once the count reaches zero.
Wrapping Up
Java's happen-before relationship is essential for ensuring data integrity and consistency in multi-threaded applications. By understanding the implications of the happen-before rules and sidestepping these common pitfalls, you can build more reliable and maintainable systems.
For further readings and advanced topics on concurrency in Java, check out Java Concurrency In Practice and the official Java Documentation on Concurrency.
Understanding and properly implementing concurrency is crucial in modern software engineering. Avoiding the common pitfalls discussed in this article will ensure your Java applications are safe, efficient, and scalable.
Happy coding!
Checkout our other articles