Avoiding Deadlocks in Java ProcessBuilder: Key Strategies
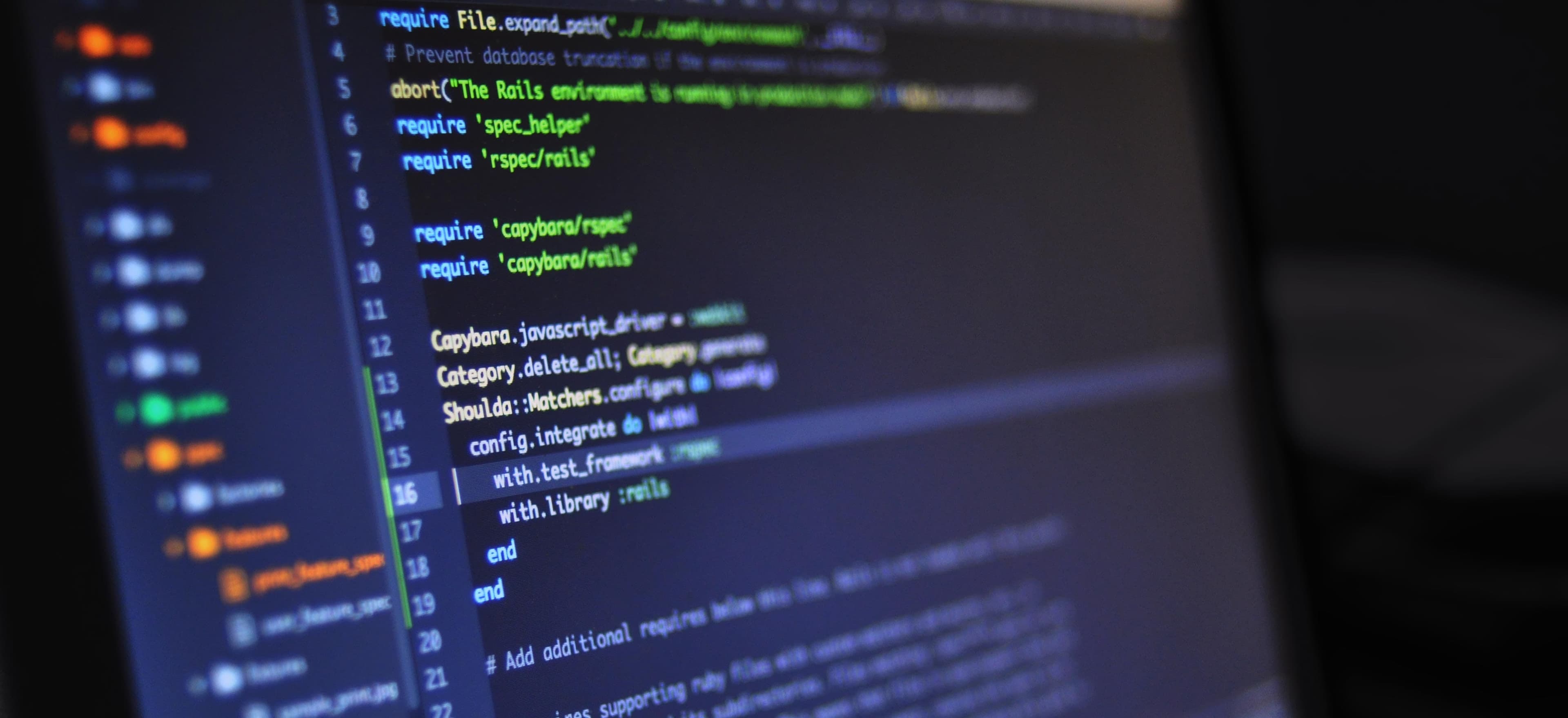
- Published on
Avoiding Deadlocks in Java ProcessBuilder: Key Strategies
Deadlocks can occur when processes or threads are waiting indefinitely for resources that other processes hold. This becomes especially pesky when working with external processes through the ProcessBuilder
class in Java, where improper management can lead to system-wide deadlocks. In this post, we will explore key strategies to avoid deadlocks when using Java's ProcessBuilder
, break down the concepts in an engaging manner, and provide well-commented code examples to clarify the why behind our strategies.
Understanding ProcessBuilder
The ProcessBuilder
class in Java is a powerful utility that allows developers to create operating system processes. This utility can start external applications, pass input, and read output. However, it also introduces complexities, especially in how these processes interact with the Java process. Let's see how it works with a simple example.
Example Code Snippet
import java.io.IOException;
public class ProcessBuilderExample {
public static void main(String[] args) {
ProcessBuilder processBuilder = new ProcessBuilder("echo", "Hello, World!");
processBuilder.redirectErrorStream(true); // Merges error and output streams
try {
Process process = processBuilder.start();
process.waitFor(); // Wait for the process to complete
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
In this code snippet, we create a simple process that echoes "Hello, World!". By merging the error and output streams with redirectErrorStream(true)
, we are already taking a step to simplify our process's output handling, which reduces the risk of deadlocks.
What Causes Deadlocks in ProcessBuilder?
Deadlocks often occur due to unconsumed input or output streams. When a Java process generates output, and this output cannot be consumed quickly enough, the underlying operating system may block the process until it can write this data to a stream. If the stream buffer fills up, the process effectively halts, leading to a situation where both the Java process and the external process are waiting for each other infinitely.
Deadlock Scenario Example
Consider an example where we instantiate a ProcessBuilder
without managing its streams carefully:
import java.io.InputStream;
public class DeadlockExample {
public static void main(String[] args) {
ProcessBuilder processBuilder = new ProcessBuilder("someCommandThatGeneratesLotsOfOutput");
try {
Process process = processBuilder.start();
InputStream inputStream = process.getInputStream();
// Failing to read the output stream here creates a deadlock since it fills up.
} catch (IOException e) {
e.printStackTrace();
}
}
}
In the above scenario, if the command generates a large amount of output, the input stream fills up, causing the process to halt and wait for the input stream to be consumed. This is a classic deadlock.
Strategies to Avoid Deadlocks
Now that we understand the causes of deadlocks, let's discuss four effective strategies to avoid them while using ProcessBuilder
.
1. Always Consume Output Streams
The most effective way to prevent deadlocks is by ensuring that all output streams (standard output and standard error) are actively consumed. This means reading the output and error streams immediately after starting the process.
Code Example
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class ProcessWithoutDeadlock {
public static void main(String[] args) {
ProcessBuilder processBuilder = new ProcessBuilder("someCommand");
try {
Process process = processBuilder.start();
// Create threads to consume output and error streams
StreamGobbler outputGobbler = new StreamGobbler(process.getInputStream());
StreamGobbler errorGobbler = new StreamGobbler(process.getErrorStream());
outputGobbler.start();
errorGobbler.start();
process.waitFor(); // Wait for the process to complete
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
class StreamGobbler extends Thread {
private final InputStream inputStream;
StreamGobbler(InputStream inputStream) {
this.inputStream = inputStream;
}
public void run() {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line); // Consume the output
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why This Works
In this code snippet, we spawn separate threads to read from both the input and error streams. This approach prevents the streams from filling up and leading to a deadlock situation.
2. Use redirectErrorStream()
As mentioned earlier, merging standard error and output streams can help in keeping track of them more efficiently. This can simplify your code and reduce the risk of deadlocks, especially with simpler commands.
Example Code
processBuilder.redirectErrorStream(true); // Handle both output and errors
3. Set Timeouts
An additional layer of safety can involve setting timeouts on reading the stream. Java’s Process
class does not support timeouts explicitly, but you can handle your implementation logic accordingly.
Example Code Snippet
class SafeStreamReader extends Thread {
private final InputStream inputStream;
SafeStreamReader(InputStream inputStream) {
this.inputStream = inputStream;
}
public void run() {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
reader.lines().forEach(System.out::println); // Print lines regularly
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why Timeouts?
Using timeouts can help terminate threads that block on reading streams. This helps prevent your application from stalling indefinitely due to unexpected output.
4. Monitor Process Lifecycle
Monitoring the lifecycle of your external processes will also let you manage resources effectively. Use .isAlive()
method to check if a process is still running and handle it appropriately.
Code Example
public class MonitorProcess {
public static void main(String[] args) {
try {
ProcessBuilder processBuilder = new ProcessBuilder("someProcess");
Process process = processBuilder.start();
while (process.isAlive()) {
// Monitor process status
System.out.println("Process is still running...");
Thread.sleep(1000); // Polling interval
}
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
Why Monitoring Works
By monitoring, you can proactively handle issues that arise from the process's output. If the process hangs or runs longer than expected, you can choose to terminate it or take necessary action.
Lessons Learned
Deadlocks in Java's ProcessBuilder
can stop your application in its tracks, leading to time inefficiencies and frustration. However, the strategies we've discussed not only help eliminate the potential for deadlocks but also promote better resource management when dealing with external processes. By always consuming output streams, merging error streams, implementing timeouts, and monitoring process lifecycles, you can avoid many common pitfalls associated with ProcessBuilder
.
The power of ProcessBuilder
is immense, and with careful handling, you can leverage its capabilities without falling prey to the pitfalls of deadlocks.
For further reading on Java's concurrency model, you can check out Java Concurrency in Practice by Brian Goetz. This book provides deeper insights into managing threads effectively in Java applications.
Checkout our other articles