Mastering Java Garbage Collector Tuning: Common Pitfalls
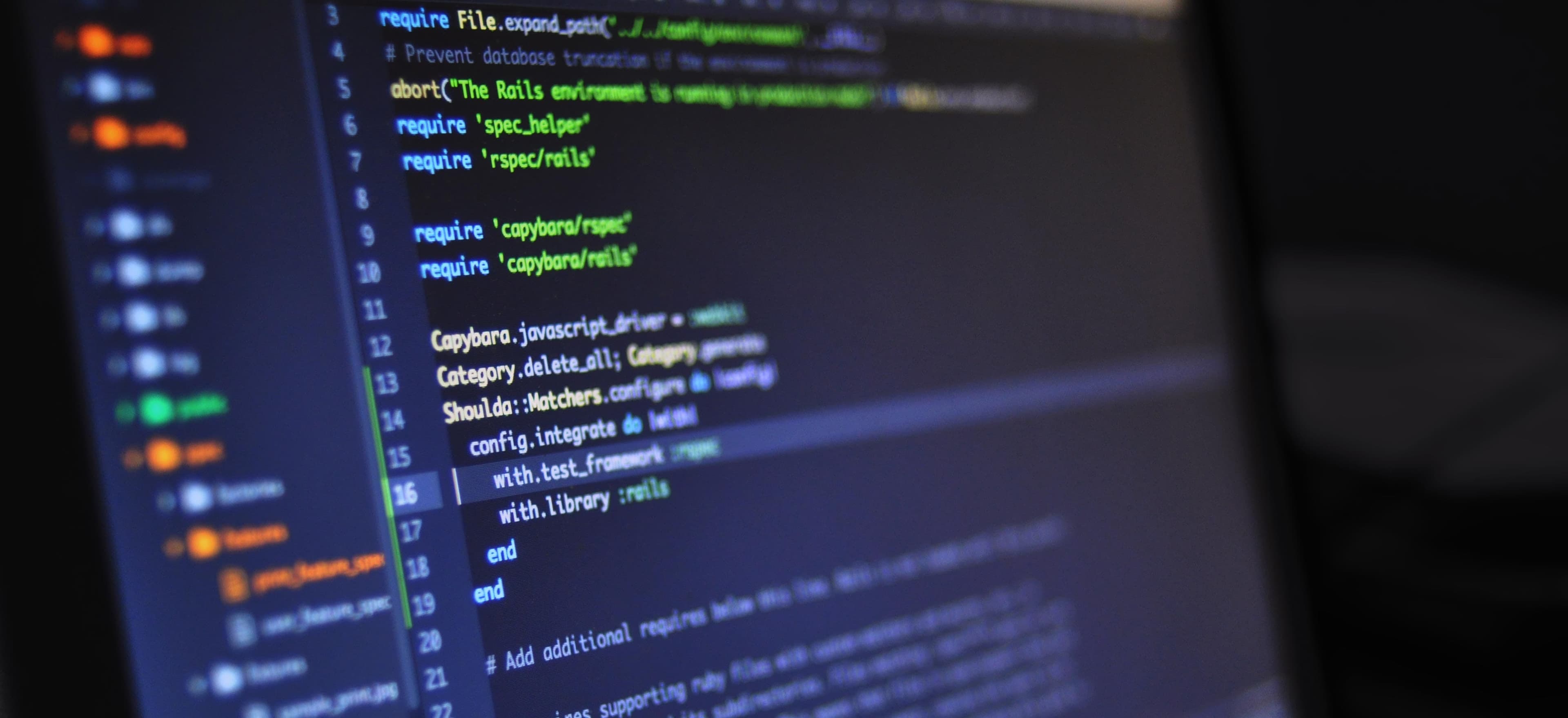
- Published on
Mastering Java Garbage Collector Tuning: Common Pitfalls
Garbage collection (GC) is a pivotal aspect of Java that impacts application performance directly. While the Java Runtime Environment (JRE) provides automatic memory management, tuning the garbage collector can be a nuanced endeavor. This post explores the essentials of Java garbage collector tuning, common pitfalls, and techniques to mitigate issues.
Understanding the Basics of Java Garbage Collection
Before diving into tuning, let's clarify what garbage collection entails. In Java, memory allocated for objects that are no longer needed must be reclaimed. The garbage collector automatically identifies these objects and removes them to free memory. While this process is largely automatic, developers can optimize the performance by tuning the garbage collector.
Key Concepts of Garbage Collection
-
Generational Collection: Java uses a generational garbage collection approach. Objects are separated into generations: Young, Old (or Tenured), and Permanent (or Metaspace). Objects in the Young generation are collected more frequently than those in the Old generation.
-
Minor vs. Major GC: A minor GC occurs in the Young generation, while a major GC collects objects in the Old generation. Tuning can help minimize the frequency and duration of these collections.
Common Pitfalls in Garbage Collector Tuning
As you embark on the tuning journey, be aware of the common pitfalls:
1. Ignoring Application Behavior
Every application has distinct memory usage patterns. Ignoring its behavior can lead to inappropriate GC settings.
// Example of monitoring memory usage
import java.lang.management.ManagementFactory;
import java.lang.management.MemoryMXBean;
public class MemoryMonitor {
public static void main(String[] args) {
MemoryMXBean memoryMXBean = ManagementFactory.getMemoryMXBean();
System.out.println("Heap Memory Usage: " + memoryMXBean.getHeapMemoryUsage());
}
}
This code snippet demonstrates fetching heap memory usage, a critical aspect of understanding your application. This data will inform your tuning decisions effectively.
2. Over-Tuning the Heap Size
Setting heap sizes too low can lead to frequent GC, while setting them too high can cause excessive pause times.
Consider the following parameters when tuning the heap size:
-Xms
(initial heap size)-Xmx
(maximum heap size)
A common pitfall is arbitrary scaling. Instead, size your heap following actual usage trends and system capabilities.
3. Neglecting GC Algorithms
Java offers different garbage collection algorithms—each suited for varying application needs. The most common ones include:
- Serial GC: Best for single-threaded applications.
- Parallel GC: Suitable for multiprocessor machines, allowing for short pause times.
- Concurrent Mark-Sweep (CMS): Reduces pause times by performing most of its work concurrently.
Utilizing the wrong algorithm can directly impact performance.
Example of setting the GC algorithm:
java -XX:+UseG1GC -Xms512m -Xmx2048m -jar your-application.jar
Here, we are using the G1 garbage collector, which is generally a good choice for large heap applications.
4. Not Enabling GC Logging
Lack of GC logging prevents you from understanding how garbage collection affects application performance. Tracking GC events helps you learn about their frequency and impact.
You can enable GC logging with:
java -Xlog:gc*:file=gc.log:time,uptime:filecount=10,filesize=10240 -jar your-application.jar
This logging will generate GC logs, helping you analyze collection times and impact on overall application performance.
5. Failing to Adjust Tuning Parameters
After initially tuning the application, it's easy to leave the settings unchanged. As your application evolves, its memory requirements and behavior do too. Regularly revisit your tuning parameters.
6. Misunderstanding Application Throughput vs. Latency
Throughput is the amount of work done in a given time, while latency refers to response time. Balancing these can be tricky.
For online applications, low latency must be prioritized. Conversely, in batch applications, throughput might be the primary focus. Make sure to understand your application's requirements.
Effective Techniques for Garbage Collector Tuning
With an awareness of these pitfalls, let's discuss effective techniques for optimizing GC:
1. Use the TLab (Thread-Local Allocation Buffers)
The TLab minimizes allocation contention among threads. By using TLab, each thread allocates memory from its buffer rather than a shared area, enhancing performance.
2. Optimize Object Creation
Reducing unnecessary object creation can lighten the load on the garbage collector.
- Reuse objects whenever possible.
- Use primitives instead of wrapper objects to decrease memory overhead.
3. Profiling and Monitoring
Make performance profiling a daily task. Use profiling tools like VisualVM or JProfiler to analyze memory usage and GC behavior. Continuous monitoring empowers you to tune proactively.
4. Analyze Thread Behavior
High thread contention can lead to GC issues. Analyze and optimize your thread usage:
// Example of optimizing threads
ExecutorService executorService = Executors.newFixedThreadPool(10);
executorService.submit(() -> {
// Task implementation
});
executorService.shutdown();
By controlling the number of concurrent threads, you can reduce contention and improve performance.
5. Use Newer Garbage Collection Algorithms
The JVM has evolved; newer algorithms like ZGC or Shenandoah provide low-latency GC at scale. These options are especially useful for large-scale applications.
java -XX:+UseZGC -Xms2g -Xmx4g -jar your-application.jar
Switching to ZGC can significantly reduce pause times for applications requiring high responsiveness.
Closing Remarks
Garbage collector tuning is a complex but essential aspect of Java application optimization. Avoiding common pitfalls, like ignoring application behavior or overlooking heap size settings, is crucial for achieving optimal performance. Regularly profile your application and remain adaptable to changing application needs.
By leveraging the techniques discussed—such as enabling logging, understanding different GC types, and minimizing object creation—you can substantially improve your application's garbage collection performance.
For those seeking a deeper dive into Java performance tuning, consider visiting these resources:
- Java Garbage Collection Basics
- Profiling Java Applications Effectively
Mastering garbage collection tuning can empower developers to build robust, efficient applications that make the most out of Java's automated memory management capabilities. Happy coding!
Checkout our other articles