Mastering NullPointerExceptions in Java Lambda Expressions
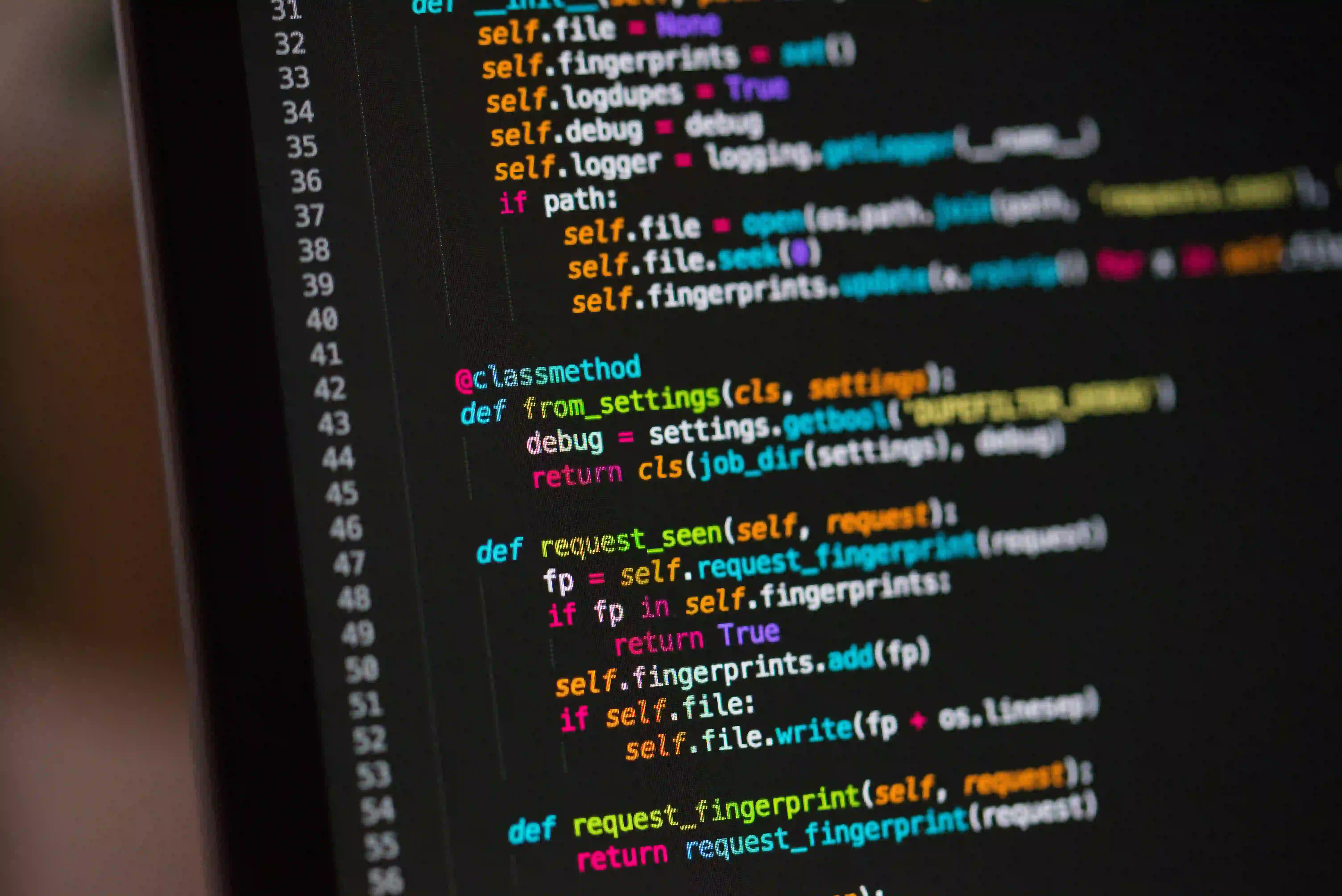
Mastering NullPointerExceptions in Java Lambda Expressions
Java has evolved significantly, particularly with the introduction of lambda expressions in Java 8. They brought about concise syntax and made it easier to work with functional programming concepts. However, one of the notorious pitfalls that developers may encounter when using lambda expressions is encountering NullPointerExceptions.
In this article, we’ll dive deep into understanding what causes NullPointerExceptions
with lambda expressions, how to avoid them, and refactoring strategies to handle similar situations. Our goal is to help you master handling NullPointerExceptions
effectively.
Understanding NullPointerExceptions
A NullPointerException
is thrown when your application attempts to use an object reference that has not been initialized or points to null
. In Java, this exception can arise in several scenarios:
- Dereferencing a
null
object - Accessing methods or fields of
null
objects - Attempting to get the length of an array that is
null
With lambda expressions, this behavior can become more pronounced since they often involve passing methods as arguments where the values might be null
.
Why Lambda Expressions?
Lambda expressions allow you to express instances of single-method interfaces (functional interfaces) in a more concise way. They encourage functional programming approaches in Java, leading to cleaner and more maintainable code.
A Common Lambda Expression Scenario
Let’s look at a typical scenario that can lead to NullPointerExceptions
. Imagine you want to filter a list of names and print them out. Here's how you could implement that using Java's lambda expressions:
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", null, "Alice", "Bob", null);
// Lambda expression to filter and print non-null names
names.stream()
.filter(name -> name != null)
.forEach(System.out::println);
}
}
Why the Above Works
In this code, we create a list of names that includes some null
values. The lambda expression inside filter
checks for null
values before proceeding to forEach
. This is crucial because if we were to directly call toUpperCase()
on a name that could potentially be null
, we would face a NullPointerException
.
Breaking the Code
Now, let’s see what happens if we forget to include the null
check:
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", null, "Alice", "Bob", null);
// Potential NullPointerException here
names.stream()
.map(String::toUpperCase)
.forEach(System.out::println);
}
}
In this version, when Java attempts to execute String::toUpperCase
on a null
value, it throws a NullPointerException
.
Key Takeaway
When dealing with lambda expressions, always consider the potential for null
values in your data. Implement necessary checks to prevent NullPointerExceptions
.
Strategies to Handle Null Values
1. Using Optional
Java 8 introduced the Optional
class, which is a powerful way to handle potential null
values. Here’s how you can refactor the previous example using Optional
:
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
public class Main {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", null, "Alice", "Bob", null);
names.stream()
.map(name -> Optional.ofNullable(name).map(String::toUpperCase).orElse("N/A"))
.forEach(System.out::println);
}
}
Why Use Optional?
The Optional
class helps eliminate null
checks with more semantic clarity. If a value is present, it executes the function; otherwise, it provides an alternative (here, “N/A”).
For more details about how Optional
works in Java, you can refer to the Java Documentation on Optional.
2. Method References & Null Checks
If you're utilizing method references in conjunction with lambda expressions, make sure your method handles null
values effectively. For instance:
public class Main {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", null, "Alice", "Bob", null);
names.stream()
.filter(Objects::nonNull)
.map(String::toUpperCase)
.forEach(System.out::println);
}
}
Why Method References with Null Checks?
By using Objects::nonNull
, we’re ensuring that only non-null values reach the mapping stage, thus significantly reducing the risk of a NullPointerException
.
Lessons Learned
Mastering NullPointerExceptions
when working with lambda expressions in Java is vital for robust application development. Remember, careful checks, using Optional
, and leveraging method references are your best tools against these exceptions.
In summary, the key practices include:
- Always vet data for potential
null
values. - Use
Optional
where applicable to provide safer handling. - Use method references efficiently to avoid complexities.
By mastering these techniques, you can ensure a smoother experience when implementing lambda expressions in your Java applications.
For further reading about functional programming in Java, consider visiting Oracle’s Guide on Functional Programming.
Now, it’s your turn! Start incorporating these techniques and produce cleaner, exception-free Java lambdas. Happy coding!