How to Safely Handle Empty Collections in Java Streams
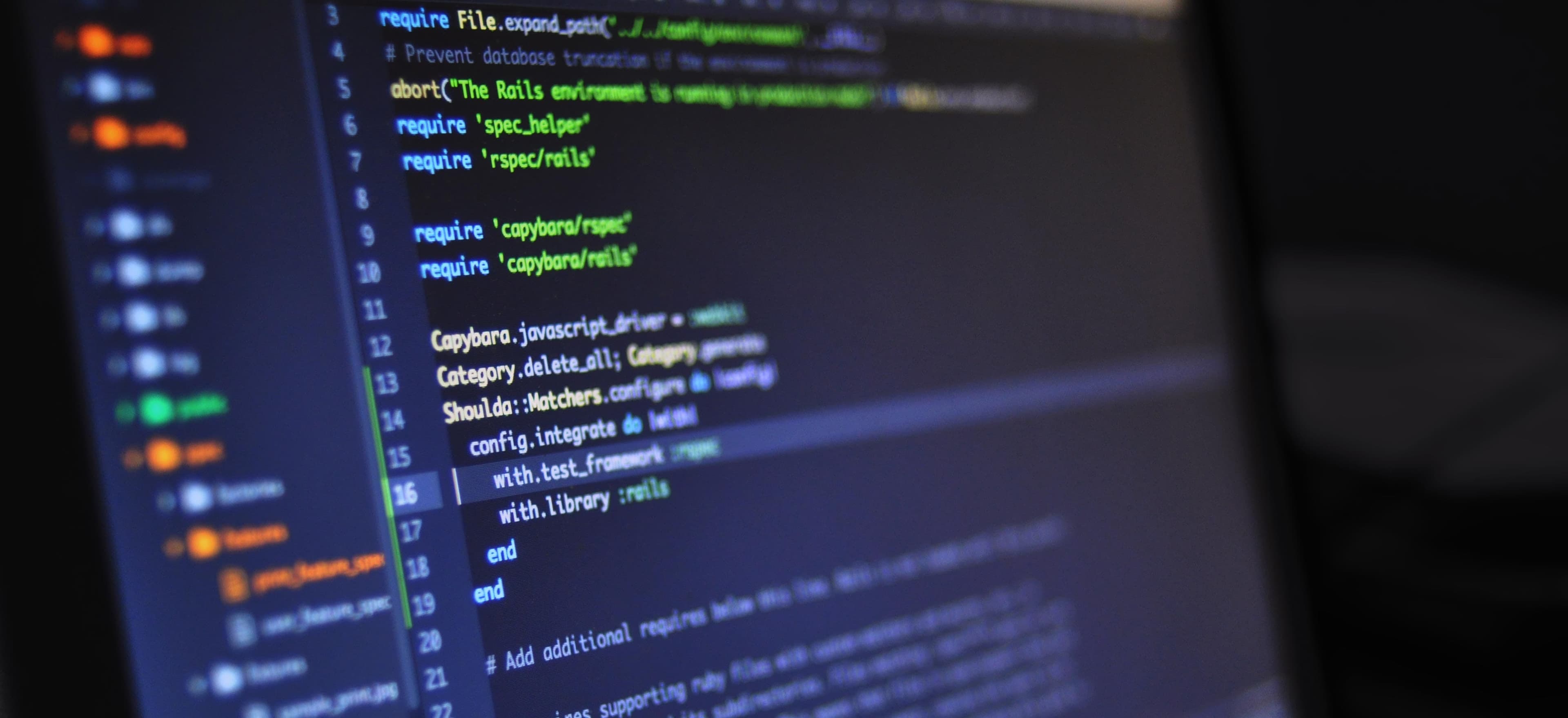
- Published on
How to Safely Handle Empty Collections in Java Streams
Java Streams provide a powerful and flexible way to process sequences of elements. However, one common challenge developers face is how to handle empty collections safely. In this blog post, we will delve into various techniques for handling empty collections, ensuring your code remains robust, readable, and efficient.
Understanding the Issue: Why Handle Empty Collections?
Empty collections are common in programming. They can occur for several reasons, such as:
- User input that returns no results.
- Data sources that may not contain any data.
- Filtering processes that result in no items.
If not handled properly, operations on empty collections can lead to unexpected behaviors or runtime exceptions. For instance, trying to access an element from an empty collection results in an IndexOutOfBoundsException
.
The Basics of Java Streams
Before diving into handling empty collections, let's quickly review how Java Streams work.
A Stream in Java is a sequence of elements that can be processed in parallel or sequentially. The key benefits of using Streams include:
- Conciseness: Code can be compactly expressed.
- Lazy Evaluation: Operations on Streams are evaluated only when necessary.
- Interoperability: Streams can be easily connected through a pipeline of operations.
Here's a simple example of creating a Stream from a List:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
}
}
This code filters names starting with 'A' and prints them. If the list is empty, no output will occur, but no exceptions will be thrown—demonstrating how Streams can gracefully handle emptiness.
Safe Handling of Empty Collections in Java Streams
1. Use Optional
to Wrap Results
One of the simplest ways to handle empty collections is by using Optional
. This approach is particularly effective when you want to retrieve a single element from a Stream. Here’s how:
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
List<String> names = Arrays.asList();
// Use findFirst() to retrieve the first element as an Optional
Optional<String> firstName = names.stream().findFirst();
// Safely handle potential absence of value
firstName.ifPresent(System.out::println);
}
}
In this example, if names
is empty, findFirst()
will return an empty Optional
, and ifPresent
will safely check the presence before printing.
2. Default Values Using orElse
Sometimes you may want to provide a default value when your collection doesn't have any elements. You can achieve this using the orElse
method.
import java.util.Arrays;
import java.util.List;
public class DefaultValueExample {
public static void main(String[] args) {
List<String> names = Arrays.asList();
String firstName = names.stream()
.findFirst()
.orElse("No names available");
System.out.println(firstName);
}
}
By providing a default value with orElse
, the output will always be meaningful, preventing potential null pointer issues when working with empty Streams.
3. Stream Operations that Handle Empties Gracefully
Several Stream operations inherently handle empty collections without throwing exceptions. For instance, methods like count()
, collect()
, and reduce()
gracefully return zero, an empty collection, or an empty Optional
, respectively.
Example: Using collect
Here’s how you can safely collect results—transforming your Stream back into a collection:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class CollectExample {
public static void main(String[] args) {
List<String> names = Arrays.asList();
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
System.out.println("Filtered List: " + filteredNames);
}
}
Even if names
is empty, filteredNames
will still be a valid empty list, devoid of exceptions.
4. Leveraging Default Stream Behavior
Java's Stream API provides a convenient method, Stream.of()
which allows creating a stream that can handle empty or non-empty entries conveniently.
import java.util.stream.Stream;
public class DefaultStreamExample {
public static void main(String[] args) {
Stream<String> emptyStream = Stream.of();
long count = emptyStream.count(); // Returns 0
System.out.println("Count of items in stream: " + count);
}
}
5. Optional Chaining for Complex Scenarios
For complex scenarios where methods may return Optional, you can chain them using flatMap
. This allows for more sophisticated handling without cluttering your code.
import java.util.Optional;
public class ChainingExample {
public static void main(String[] args) {
Optional<List<String>> optionalNames = Optional.of(Arrays.asList());
long nameCount = optionalNames
.flatMap(names -> names.stream().findFirst())
.map(name -> name.length())
.orElse(0); // Default to 0 if the list is empty.
System.out.println("Length of first name: " + nameCount);
}
}
The Last Word
Effective handling of empty collections in Java Streams is crucial for creating robust applications. By leveraging Optional
, default values, inherent Stream behaviors, and chaining methods, you can write safe, clean, and maintainable code.
If you find this topic intriguing, you may explore more about the Java Stream API in the official Java Documentation to deepen your understanding.
Final Thoughts
Handling empty collections is just one aspect of efficient data processing with Java Streams. Embrace these techniques to build more resilient applications, and remember that the use of Optional
and safe defaults can significantly reduce the risk of runtime exceptions. Always code with the potential for emptiness in mind as this approach is not just best practice — it’s essential for quality software development.
Checkout our other articles