Ensuring Thread-Safety in Java Singleton Patterns
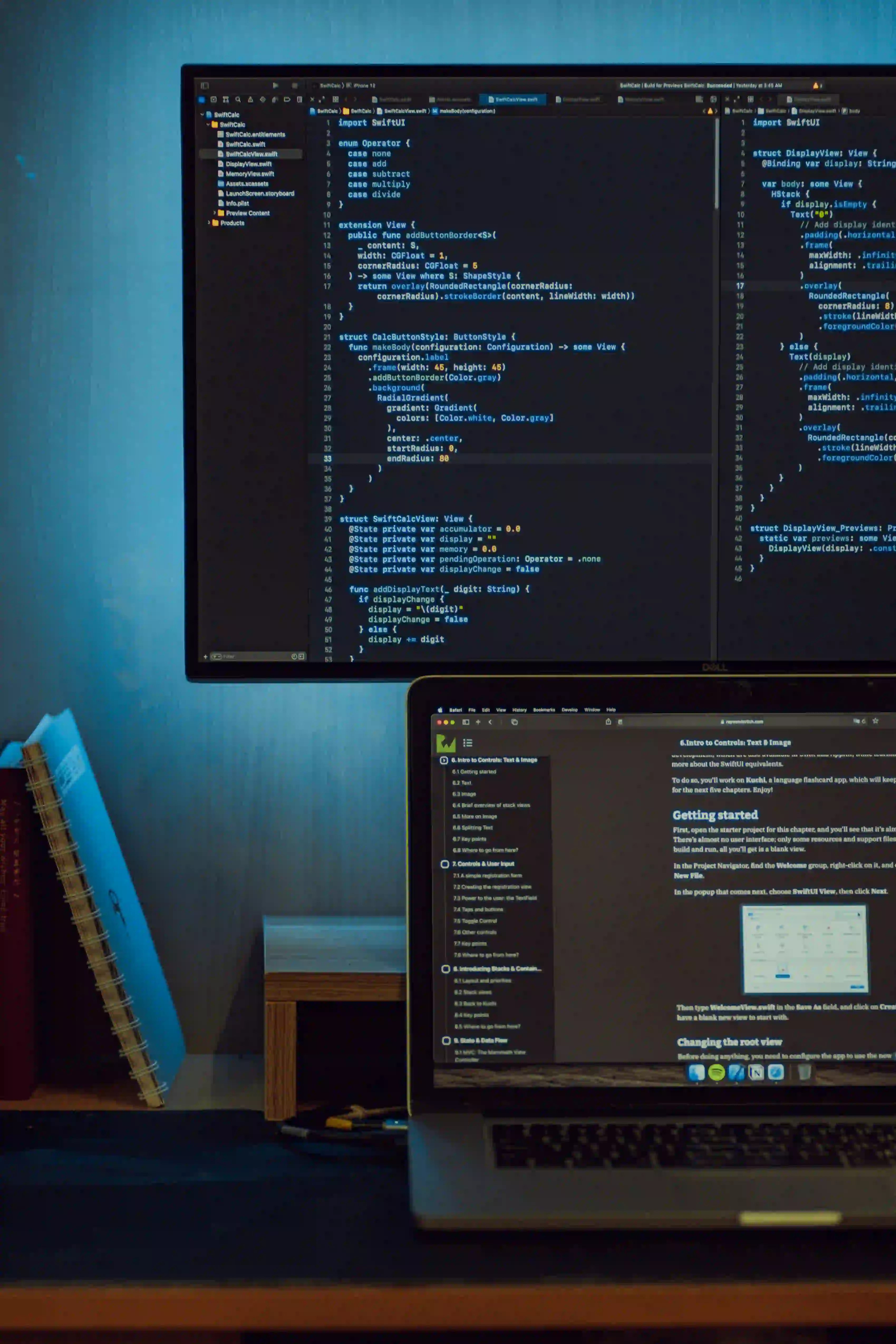
Ensuring Thread-Safety in Java Singleton Patterns
Singleton design pattern is a fundamental concept in software development, especially in Java, where it ensures that a class has only one instance and provides a global point of access to that instance. Thread-safety becomes crucial when multiple threads access the singleton instance simultaneously. In this blog post, we will explore various ways to implement thread-safe Singleton patterns in Java, elaborate on their pros and cons, and provide practical code examples.
What is a Singleton Pattern?
Before delving into thread-safety, let's clarify what a Singleton pattern is. The Singleton pattern restricts the instantiation of a class to a single object. This is particularly beneficial for managing shared resources, such as configurations or logs, where maintaining a single point of access is paramount.
Why Use a Singleton?
- Controlled access to resources: Ensures that one object controls the entire resource management process.
- Reduced namespace: Avoids polluting the global namespace with numerous instance variables.
- Enhanced performance: Avoids the overhead of object creation, leading to improved performance in certain scenarios.
To understand how to make a Singleton pattern thread-safe, we need to look at different approaches.
Common Methods for Implementing a Singleton Pattern
1. Eager Initialization
In this approach, the singleton instance is created at the time of class loading. This method is simple and thread-safe by nature.
public class EagerSingleton {
private static final EagerSingleton INSTANCE = new EagerSingleton();
private EagerSingleton() {
// private constructor to prevent instantiation
}
public static EagerSingleton getInstance() {
return INSTANCE;
}
}
Why Use It?
The instance is created once when the class is loaded, ensuring thread safety without synchronization overhead. However, the downside is that the instance gets created even if it is never used.
2. Lazy Initialization (Thread-Safe)
The lazy initialization method defers the creation of the Singleton instance until it is requested. However, we need to ensure it's thread-safe.
public class LazySingleton {
private static LazySingleton instance;
private LazySingleton() {
// private constructor
}
public static synchronized LazySingleton getInstance() {
if (instance == null) {
instance = new LazySingleton();
}
return instance;
}
}
Why Use It?
The synchronized keyword ensures that only one thread can access the method at a time, making it safe in a multithreaded environment. However, this can lead to performance issues, as every call to getInstance
must acquire a lock.
3. Double-Checked Locking
To optimize the performance of the lazy initialization pattern, we can use double-checked locking.
public class DoubleCheckedLockingSingleton {
private static volatile DoubleCheckedLockingSingleton instance;
private DoubleCheckedLockingSingleton() {
// private constructor
}
public static DoubleCheckedLockingSingleton getInstance() {
if (instance == null) {
synchronized (DoubleCheckedLockingSingleton.class) {
if (instance == null) {
instance = new DoubleCheckedLockingSingleton();
}
}
}
return instance;
}
}
Why Use It?
This method minimizes the overhead of acquiring a lock by first checking if the instance is null
without synchronization. If it is null, only then does it synchronize. The use of volatile
prevents instruction reordering that could lead to visibility issues.
4. Bill Pugh Singleton Design
This method utilizes an inner static helper class to hold the instance of the Singleton class. This approach is both thread-safe and efficient.
public class BillPughSingleton {
private BillPughSingleton() {
// private constructor
}
private static class SingletonHelper {
private static final BillPughSingleton INSTANCE = new BillPughSingleton();
}
public static BillPughSingleton getInstance() {
return SingletonHelper.INSTANCE;
}
}
Why Use It?
The singleton instance is created only when the getInstance()
method is called, leveraging Java's classloader mechanism to ensure thread-safety. This design avoids the overhead associated with synchronized methods while being lazy in the instantiation.
5. Enum Singleton
The simplest and most robust way to create a Singleton in Java is using an enum.
public enum EnumSingleton {
INSTANCE;
public void someMethod() {
// some logic here
}
}
Why Use It?
Enum singletons are inherently serializable, and since the JVM handles the instance creation, this approach is automatically thread-safe. It also handles the issue of preventing multiple instances during serialization.
Performance Comparison
To sum up the various methods for implementing a Singleton, here’s a quick comparison based on factors like simplicity, performance, and thread safety:
| Method | Simplicity | Performance | Thread-Safety | |-------------------------|------------|-------------|---------------| | Eager Initialization | High | Low | High | | Lazy Initialization | Medium | Low | Medium | | Double-Checked Locking | Medium | High | High | | Bill Pugh | High | High | High | | Enum | High | High | High |
Best Choice: If your Singleton implementation requires lazy loading, Bill Pugh or Enum are both excellent choices.
Bringing It All Together
Creating a Singleton pattern in Java requires careful consideration of thread-safety, performance optimization, and ease of use. Whether you choose to implement an eager initialization or one of the lazy techniques, understanding the underlying mechanisms is essential.
For further reading, consider exploring the following resources:
- Singleton Design Pattern in Java for a comprehensive breakdown.
- Effective Java by Joshua Bloch for best practices in Java programming.
By employing these various options and understanding their implications, you can effectively implement thread-safe Singleton patterns in your Java applications. Happy coding!