Stop Memory Leaks: Mastering Anonymous Classes in Java
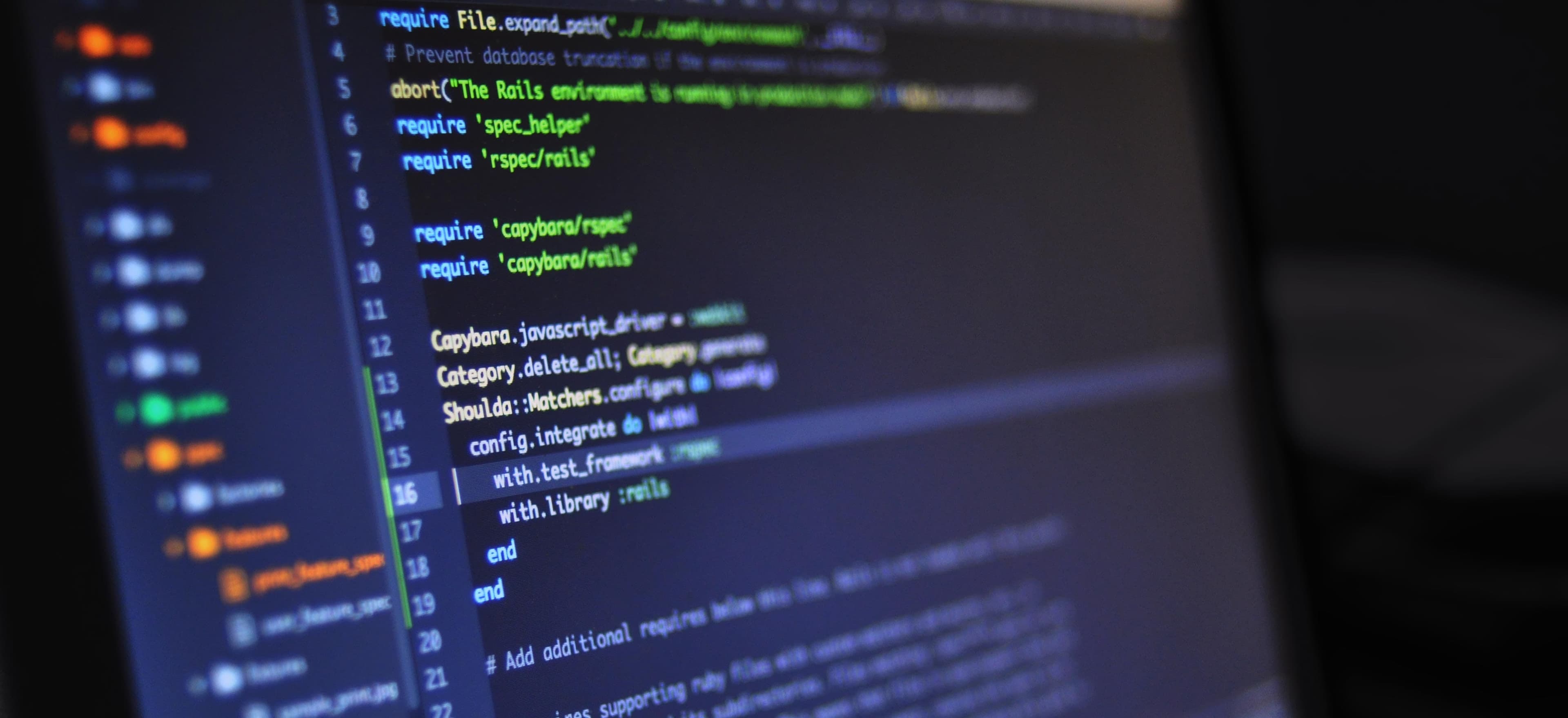
- Published on
Stop Memory Leaks: Mastering Anonymous Classes in Java
Memory management is a crucial aspect of programming in Java. It not only optimizes the application's performance but also prevents potential crashes. Among the various features Java provides, anonymous classes are both powerful and perilous. When mismanaged, they can lead to memory leaks that can cripple your application. In this blog post, we will explore anonymous classes in Java, how they function, their best practices, and strategies to prevent memory leaks.
What is an Anonymous Class?
An anonymous class in Java is a kind of inner class without a name. It enables you to instantiate a class and at the same time override some of its methods. Anonymous classes are often used to implement interfaces or extend class behavior in a concise manner. Consider the following code snippet:
interface Greeting {
void sayHello();
}
public class Main {
public static void main(String[] args) {
Greeting greeting = new Greeting() {
public void sayHello() {
System.out.println("Hello, World!");
}
};
greeting.sayHello();
}
}
Why Use Anonymous Classes?
- Conciseness: They allow for more compact code.
- Immediate implementation: Ideal for quick and simple event listeners.
- Encapsulation: They keep implementation details close to their usage.
While these attributes are advantageous, they come with risks that can lead to memory-related problems.
Memory Leaks Explained
A memory leak occurs when objects are no longer accessible but still occupy memory. In Java, this can lead to exhausting the heap space, causing the application to slow down or crash.
In the context of anonymous classes, memory leaks often arise due to unintended references to the outer classes. Here's an example to illustrate this concept:
public class OuterClass {
private String outerField = "Outer Field";
public void createAnonymousClass() {
// Anonymous class referencing outer class variable
new Thread(new Runnable() {
public void run() {
System.out.println(outerField);
}
}).start();
}
}
Explanation of the Memory Leak
In the example above, the anonymous class keeps a reference to its containing class instance (OuterClass
). If you create multiple instances of OuterClass
, those outer instances remain in memory until the threads are terminated, leading to a memory leak. Now, let’s explore how to prevent this.
Strategies to Prevent Memory Leaks with Anonymous Classes
1. Avoid Unnecessary References
Where possible, avoid using member variables from the outer class in the anonymous class. If you need to pass data, consider using method parameters or local variables.
Example:
public void createAnonymousClass() {
final String localVar = "Local Variable";
new Thread(new Runnable() {
public void run() {
System.out.println(localVar);
}
}).start();
}
In this example, we use a final local variable instead of an outer class member, which limits the scope of the reference.
2. Use Weak References
For scenarios where you must keep a reference to the outer class, consider using WeakReference
. This allows garbage collection to clean up the reference when needed.
Example:
import java.lang.ref.WeakReference;
public class OuterClass {
private String outerField = "Outer Field";
public void createAnonymousClass() {
final WeakReference<OuterClass> weakRef = new WeakReference<>(this);
new Thread(new Runnable() {
public void run() {
OuterClass outer = weakRef.get();
if (outer != null) {
System.out.println(outer.outerField);
}
}
}).start();
}
}
This way, if OuterClass
isn’t strongly referenced anywhere else, it can be garbage collected, preventing a memory leak.
3. Use static
Nested Classes
If you do not need to access the outer class instance, consider using a static
nested class instead of an anonymous class. Static nested classes do not hold an implicit reference to the outer class.
Example:
public class OuterClass {
private static String outerField = "Static Outer Field";
static class NestedClass implements Runnable {
public void run() {
System.out.println(outerField);
}
}
public void createNestedClass() {
new Thread(new NestedClass()).start();
}
}
By employing a static nested class, you create a clear demarcation from the outer class, lowering memory pressure.
Summary
Anonymous classes in Java provide a convenient way to define classes on-the-fly. However, their use can complicate memory management if not handled with care. Memory leaks often result from unintended references to outer classes, leading to poor performance or application crashes.
To recap, here are three main strategies to combat memory leaks associated with anonymous classes:
- Avoid unnecessary outer class references: Use local or final variables instead.
- Use WeakReferences: This allows the garbage collector to reclaim memory if necessary.
- Opt for static nested classes: Use them when you don't need direct access to the outer instance.
By understanding and implementing these strategies, you can harness the power of anonymous classes in Java without the fear of memory-related issues. If you're interested in further reading on memory management in Java, check out Java Memory Management and Understanding Memory Leaks in Java.
Embrace these techniques, and you'll be well on your way to writing efficient, leak-free Java applications!
Checkout our other articles