Mastering Precision: Fixing Float and Double Errors in Java
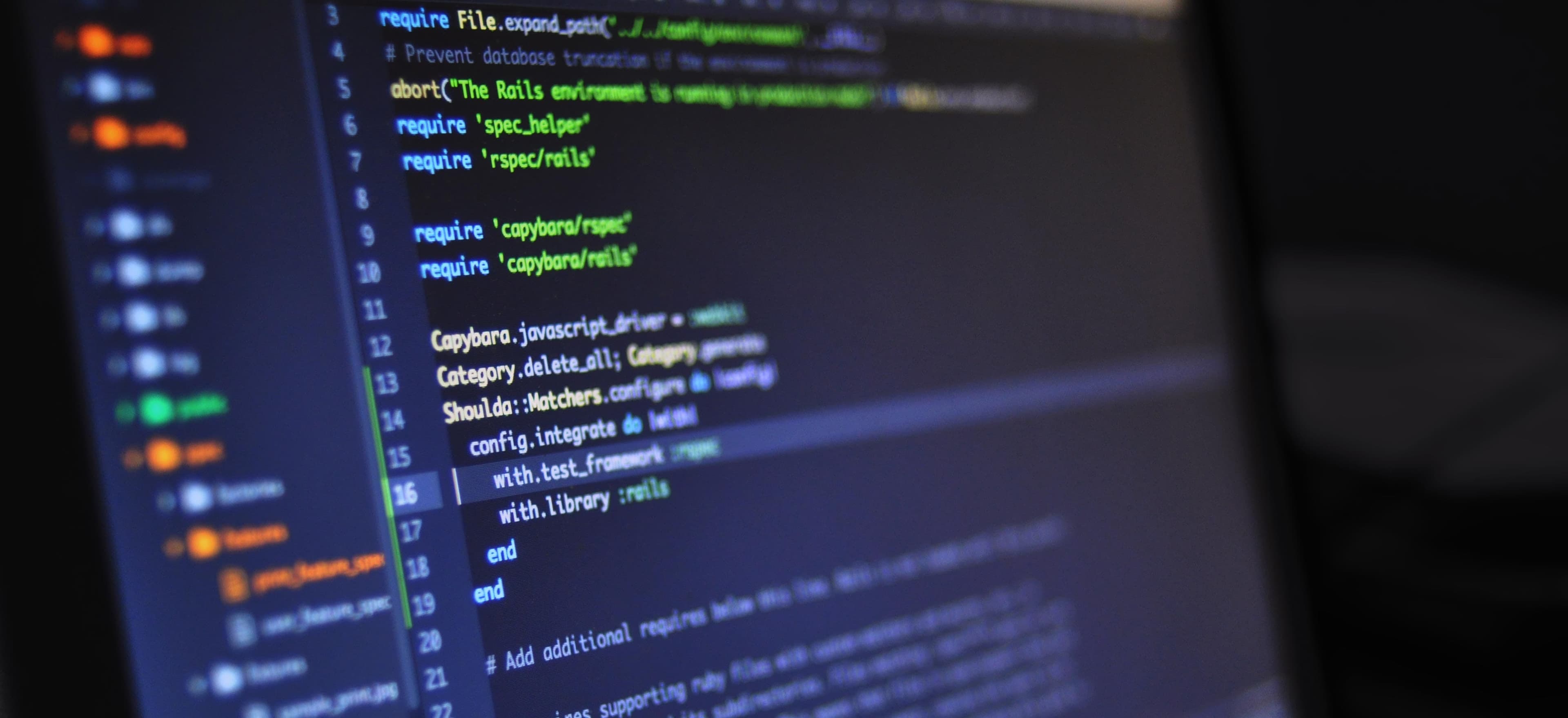
- Published on
Mastering Precision: Fixing Float and Double Errors in Java
Java is a powerful programming language that provides developers with various data types. Among these, floating-point numbers (float and double) are commonly used for representing non-integer values. However, a frequent issue many developers encounter is precision errors associated with these data types. In this blog post, we will explore the underlying reasons for these errors and provide solutions to fix them. Whether you are a seasoned Java developer or just starting, this guide will help you understand how to handle floating-point precision more effectively.
Understanding Floating-Point Numbers
In Java, floating-point numbers are represented using the IEEE 754 standard. This standard defines the format for representing real numbers in binary, which allows for an efficient use of memory and computational speed. However, the binary representation can lead to precision errors, especially when performing arithmetic operations.
Float vs. Double
Java provides two types of floating-point numbers:
float
: A single-precision 32-bit IEEE 754 floating point.double
: A double-precision 64-bit IEEE 754 floating point.
Here's a quick comparison:
| Type | Memory Size | Precision | |--------|-------------|-------------------------| | float | 4 bytes | 7 decimal digits | | double | 8 bytes | 15 decimal digits |
While float
consumes less memory, double
is often preferred due to its higher precision, which reduces the likelihood of significant rounding errors.
float floatValue = 0.1f; // density
double doubleValue = 0.1; // velocity
Note the use of the suffix f
for float values, as Java defaults to double for decimal literals.
The Precision Problem
The Issue
Floating-point precision issues arise due to their representation in binary. For example, the decimal fraction 0.1 cannot be represented exactly as a binary fraction. This leads to unexpected results when performing arithmetic.
public class PrecisionProblem {
public static void main(String[] args) {
float floatSum = 0.1f + 0.2f;
double doubleSum = 0.1 + 0.2;
System.out.println("Float Sum: " + floatSum); // Outputs: 0.30000001
System.out.println("Double Sum: " + doubleSum); // Outputs: 0.30000000000000004
}
}
The Result
You might expect the output to be 0.3
, but instead, you see small discrepancies. This is the essence of floating-point arithmetic: imprecision due to binary representation.
Solutions to Fix Float and Double Errors
1. Using BigDecimal
One of the most reliable ways to avoid floating-point precision errors is to use the BigDecimal
class. This class provides a way to perform high-precision arithmetic. However, it is critical to note that the constructor expects a String
representation of the number to maintain precision.
import java.math.BigDecimal;
public class BigDecimalExample {
public static void main(String[] args) {
BigDecimal firstValue = new BigDecimal("0.1");
BigDecimal secondValue = new BigDecimal("0.2");
BigDecimal sum = firstValue.add(secondValue);
System.out.println("BigDecimal Sum: " + sum); // Outputs: 0.3
}
}
Why BigDecimal?
Using BigDecimal
avoids the pitfalls of binary floating-point arithmetic. Its benefits are particularly pronounced in financial calculations, where precision is paramount.
2. Rounding Modes
When working with BigDecimal
, you can also specify rounding modes to control how calculations behave when they exceed precision.
import java.math.BigDecimal;
import java.math.RoundingMode;
public class RoundingExample {
public static void main(String[] args) {
BigDecimal value = new BigDecimal("0.123456789");
BigDecimal roundedValue = value.setScale(2, RoundingMode.HALF_UP); // 0.12
System.out.println("Rounded Value: " + roundedValue);
}
}
3. Simple Alternative Strategies
If you are looking for simpler alternatives and can tolerate some imprecision, you can scale your numbers up and use integers for calculations.
public class ScaledExample {
public static void main(String[] args) {
int firstValue = 1; // Represents 0.1
int secondValue = 2; // Represents 0.2
int scaledSum = firstValue + secondValue;
// Dividing by 10, the scale factor
double result = scaledSum / 10.0;
System.out.println("Scaled Sum: " + result); // Outputs: 0.3
}
}
4. Utilizing Libraries
Several libraries can help manage floating-point arithmetic effectively. Notably, Apache Commons Math provides utilities that can handle mathematical operations more reliably than using the standard floating-point types.
Explore the library here.
Practical Considerations
When deciding which approach to take, remember to consider the implications of each method. Here are a few points to ponder:
- Performance:
BigDecimal
is generally slower than float/double operations. Use it when precision is necessary. - Simplicity: Scaling can simplify operations but be aware that it may introduce complexity in tracking scales.
- Domain Context: In certain applications like scientific computations, floats or doubles may be more acceptable than in financial systems where precision is critical.
Key Takeaways
In conclusion, floating-point precision issues in Java can complicate the lives of developers. By using data types like BigDecimal
, understanding rounding, scaling, and potentially utilizing libraries, you can effectively manage and rectify these precision errors.
As you delve deeper into Java, keep in mind the importance of choosing the correct data type for your needs; your choice will have significant repercussions on your application's performance and accuracy.
For further reading on floating-point arithmetic and its underlying principles, check out the IEEE 754 standard documentation here.
With this knowledge, you are now better equipped to handle floating-point numbers in Java confidently. Happy coding!
Checkout our other articles