Understanding HashMap Collisions: Boosting Java Performance
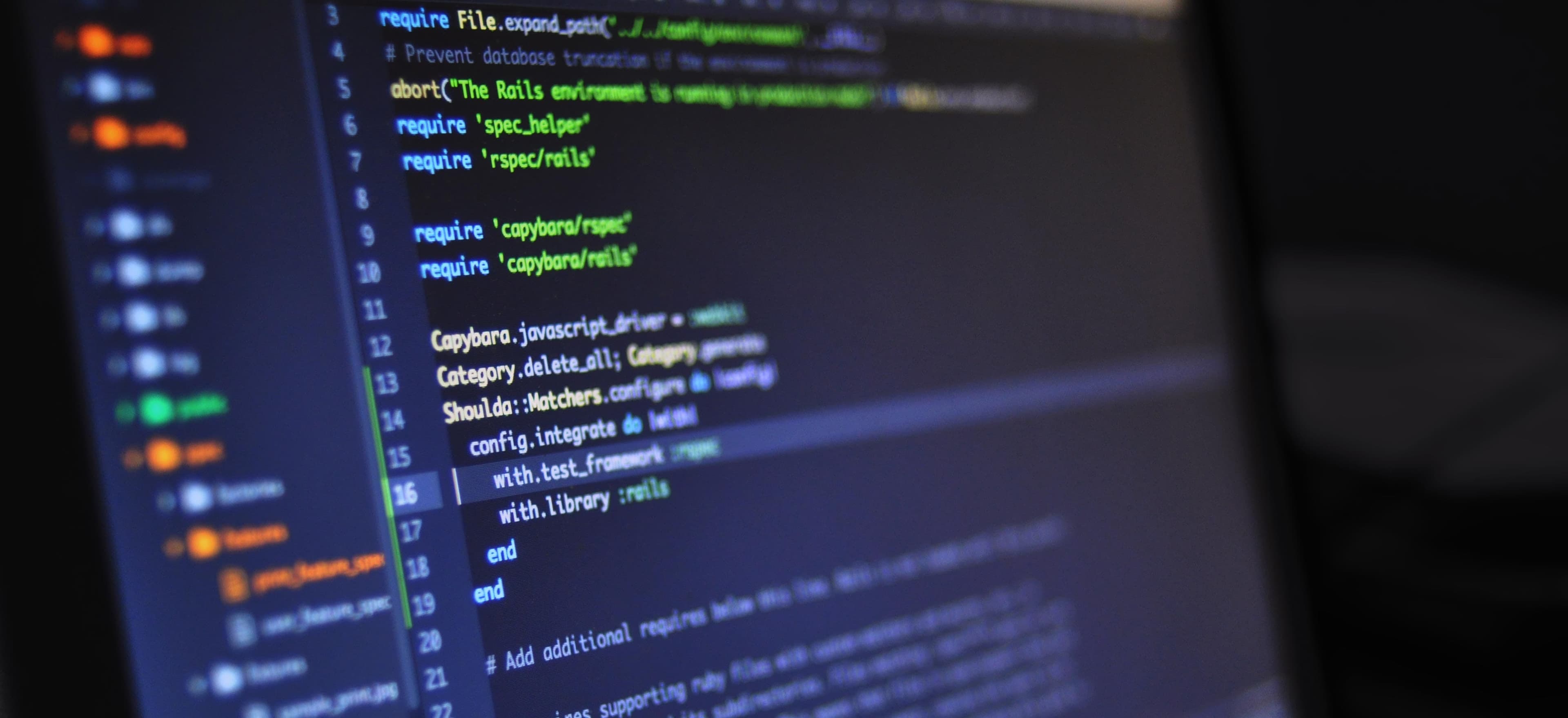
- Published on
Understanding HashMap Collisions: Boosting Java Performance
When you’re building efficient Java applications, the performance of your data structures plays a pivotal role. Among them, HashMap
stands out as a popular choice due to its average-case time complexity of O(1) for basic operations such as add, remove, and contains. However, there is an underlying complexity: collisions. This blog post aims to explore HashMap collisions and how they impact performance, providing practical code examples to illustrate key concepts.
What is a HashMap?
Before diving into collisions, let's quickly recap what a HashMap is. A HashMap is a part of Java’s Collection Framework, and it stores key-value pairs. The key is hashed to determine its storage location (bucket) in the map, which enables quick retrieval.
Basic Structure
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
System.out.println(map.get("Two")); // Output: 2
}
}
In this simple scenario, we created a HashMap, added three key-value pairs, and retrieved one value. Now, let's discuss what happens when two different keys hash to the same bucket.
What are HashMap Collisions?
A collision occurs when two keys produce the same hash code and, as a result, are stored in the same bucket. When collisions happen, the HashMap uses a strategy to store multiple entries.
How Does Java Handle HashMap Collisions?
Historically, Java implemented collision resolution via linked lists. Under this approach, each bucket might contain multiple entries, where collisions are handled by chaining.
Example of Collision Handling with Linked List
import java.util.HashMap;
class User {
private String name;
public User(String name) {
this.name = name;
}
@Override
public int hashCode() {
return name.length(); // Intentionally simplistic for demonstration
}
@Override
public String toString() {
return name;
}
}
public class CollisionExample {
public static void main(String[] args) {
HashMap<User, String> userMap = new HashMap<>();
userMap.put(new User("Alice"), "Admin");
userMap.put(new User("Bob"), "User");
userMap.put(new User("Carol"), "User"); // Let's assume Carol also hashes to the same value
System.out.println(userMap.get(new User("Alice"))); // Output: Admin
System.out.println(userMap.get(new User("Carol"))); // Output might fetch Carol's entry
}
}
Why Linked Lists?
Using linked lists allows the HashMap to handle multiple entries in the same bucket. However, this comes with performance costs. If many keys hash to the same bucket, the average complexity for adding or retrieving elements degrades to O(n), where n is the number of elements in the bucket.
Evolution to Balanced Trees
Starting from Java 8, the handling of collisions has improved significantly. When the number of entries in a bucket exceeds a certain threshold (typically 8), the linked list is converted into a balanced tree (specifically a red-black tree). This transformation enhances retrieval operations to an average of O(log n).
Example of Trees in HashMap
import java.util.HashMap;
public class TreeCollisionExample {
public static void main(String[] args) {
HashMap<String, String> userRoles = new HashMap<>();
// Simulating hash collisions
for (int i = 0; i < 10; i++) {
userRoles.put("user" + i, "role" + (i % 5)); // Potential for collisions
}
System.out.println(userRoles);
}
}
Here we add multiple keys that will most likely hash to common buckets. If the number of keys in those buckets exceeds the threshold, Java 8 converts the structure to a balanced tree, thereby maintaining performance.
Why Optimize HashMap Usage?
Understanding how collisions affect performance is crucial. Poorly designed hash functions or high degrees of collisions can lead to inefficient access times:
- Load Factor: The default load factor of 0.75 strikes a balance between space and time complexity. However, if you know you're going to store many entries, consider adjusting the initial capacity and load factor when you instantiate your HashMap to reduce collisions.
Example with load factor:
int initialCapacity = 16;
float loadFactor = 0.75f;
HashMap<String, String> optimizedMap = new HashMap<>(initialCapacity, loadFactor);
- Hash Function: A bad hash function leads to a higher likelihood of collisions. It’s advisable to override the
hashCode()
method thoughtfully if you’re using custom objects as keys.
Understanding Performance Trade-offs
Despite the optimizations Java has introduced to handle collisions, there are still trade-offs when using HashMap
:
- Memory Overhead: Implementing balanced trees incurs additional memory usage.
- Complexity of Implementation: A balance between time and space efficiency can complicate implementation.
For more details on HashMap and its best practices, check out Oracle’s documentation on HashMap.
The Last Word
HashMap collisions are an essential topic for any Java developer aiming to write performant code. By understanding how collisions can impact performance, you can make smarter decisions when choosing data structures for your applications. Being aware of default behavior and tuning them according to your use case can significantly enhance performance.
Further Reading
- Java HashMap: How It Works, Best Practices, and Pitfalls
- Java Collections Framework: A Comprehensive Guide
Your performance will greatly benefit from this foundational knowledge concerning HashMap collisions. Equip yourself with it, and you’ll be better prepared to build efficient Java applications!
Checkout our other articles