Navigating Java DateTime Challenges Across Time Zones
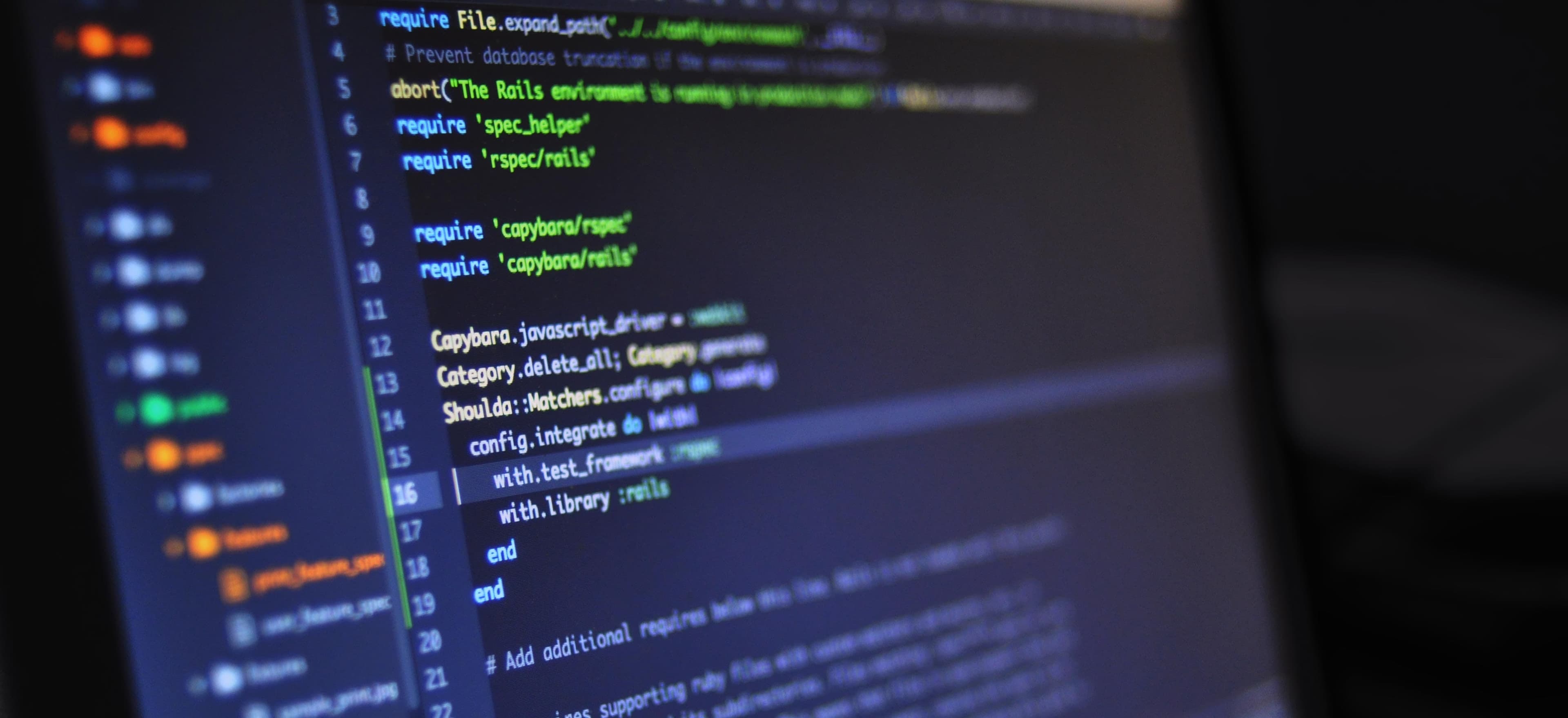
- Published on
Navigating Java DateTime Challenges Across Time Zones
Managing dates and times in software applications can be an intricate matter, especially when dealing with multiple time zones. Java, as one of the most widely used programming languages, has its own set of tools and libraries to help developers efficiently handle date and time operations. In this blog post, we will explore the challenges of managing time zones in Java, and how to best implement solutions using modern APIs.
Understanding Time Zones
A time zone is a region of the Earth that has the same standard time. While this concept seems straightforward, complexities arise when dealing with Daylight Saving Time (DST), historical changes in time zones, and global interactions.
Consider the following scenario: You have an application that deals with users from various time zones, and you want to show them the same event in their local time. Without a proper understanding of how to navigate these time zones, you could easily confuse your users.
Java Date and Time API
In Java, dates and times are not managed effectively until Java 8, when the new java.time package was introduced. This API is built around the ISO calendar system and takes into consideration time zone complexities.
Key Classes
- LocalDate: Represents a date without time zone information.
- LocalTime: Represents time without date and time zone information.
- ZonedDateTime: Represents a date-time with a time zone.
These enhancements make managing time simpler. Let's take a closer look at some code examples.
Example 1: Getting Current Time in Different Time Zones
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
import java.time.ZoneId;
public class TimeZoneExample {
public static void main(String[] args) {
// Getting current time in specified time zones
ZonedDateTime newYorkTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
ZonedDateTime londonTime = ZonedDateTime.now(ZoneId.of("Europe/London"));
// Formatting the output
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss Z");
System.out.println("Current time in New York: " + newYorkTime.format(formatter));
System.out.println("Current time in London: " + londonTime.format(formatter));
}
}
Why This Code Works
In the above code, we first import the necessary classes from java.time
. The ZoneId.of()
method allows us to specify which time zone we want to work with. Using ZonedDateTime.now()
, we fetch the current date and time in that specific zone. Finally, we use a date-time formatter to present the output neatly.
Example 2: Converting Between Time Zones
One of the common operations is to convert a date-time in one time zone to another. Let's see how we can achieve this:
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class TimeZoneConversion {
public static void main(String[] args) {
// Original time in New York
ZonedDateTime newYorkTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
System.out.println("New York time: " + newYorkTime);
// Convert to Tokyo time
ZonedDateTime tokyoTime = newYorkTime.withZoneSameInstant(ZoneId.of("Asia/Tokyo"));
System.out.println("Converted to Tokyo time: " + tokyoTime);
}
}
Why This Code Works
In this example, withZoneSameInstant()
is crucial. It converts the time to Tokyo's time zone while ensuring that the instant (the actual moment in time) remains unchanged. This avoids errors that may occur from simply changing the zone without consideration of the actual time offset.
Handling Daylight Saving Time
One major issue with time zones is how Daylight Saving Time (DST) impacts time shifts. In some regions, the clocks move forward one hour in the spring and back in the fall. This can create confusion when scheduling events.
Here’s how to account for DST:
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class DaylightSavingExample {
public static void main(String[] args) {
ZonedDateTime summerTime = ZonedDateTime.of(2023, 6, 15, 12, 0, 0, 0, ZoneId.of("America/New_York"));
ZonedDateTime winterTime = ZonedDateTime.of(2023, 12, 15, 12, 0, 0, ZoneId.of("America/New_York"));
System.out.println("Summer time: " + summerTime);
System.out.println("Winter time: " + winterTime);
}
}
Why This Code Works
By creating two ZonedDateTime
instances for summer and winter months, we can clearly see the impact DST has on time representation. This careful consideration is essential when displaying or processing time-sensitive information.
Best Practices for Time Zone Management
-
Always Use UTC for Storage: When storing timestamps in databases, always convert them to Coordinated Universal Time (UTC). This ensures consistency, regardless of the time zone of users.
-
Convert to User's Local Time: When displaying times to users, always convert from UTC to the user's local time zone. This enhances the user experience as they see the times relevant to their location.
-
Test Across Time Zones: It's vital to test your application across different time zones, especially if it’s time-sensitive (like scheduling).
-
Stay Updated: Keep your time zone data up to date, as changes occur frequently (regions may change their DST observance).
-
Utilize Libraries: Consider using libraries like Joda-Time if you're working on Java versions before 8. Joda-Time provides comprehensive time zone management features.
A Final Look
Navigating the intricacies of date and time management across time zones in Java may initially seem daunting. However, armed with the modern capabilities of the Java Date and Time API introduced in Java 8, developers can handle this complexity with ease. Understanding the various classes and methods available, along with best practices, will empower firms to create applications that serve users accurately, regardless of their geographical locations.
For further reading on handling date-time complexities, refer to the official Java Time API documentation and consider exploring community resources like Baeldung for more nuanced articles and examples.
With this knowledge, you can navigate the time zones in Java smoothly, providing a better user experience and strengthening your application’s reliability.
Checkout our other articles