Understanding Type Erasure: Pitfalls in Java Generics
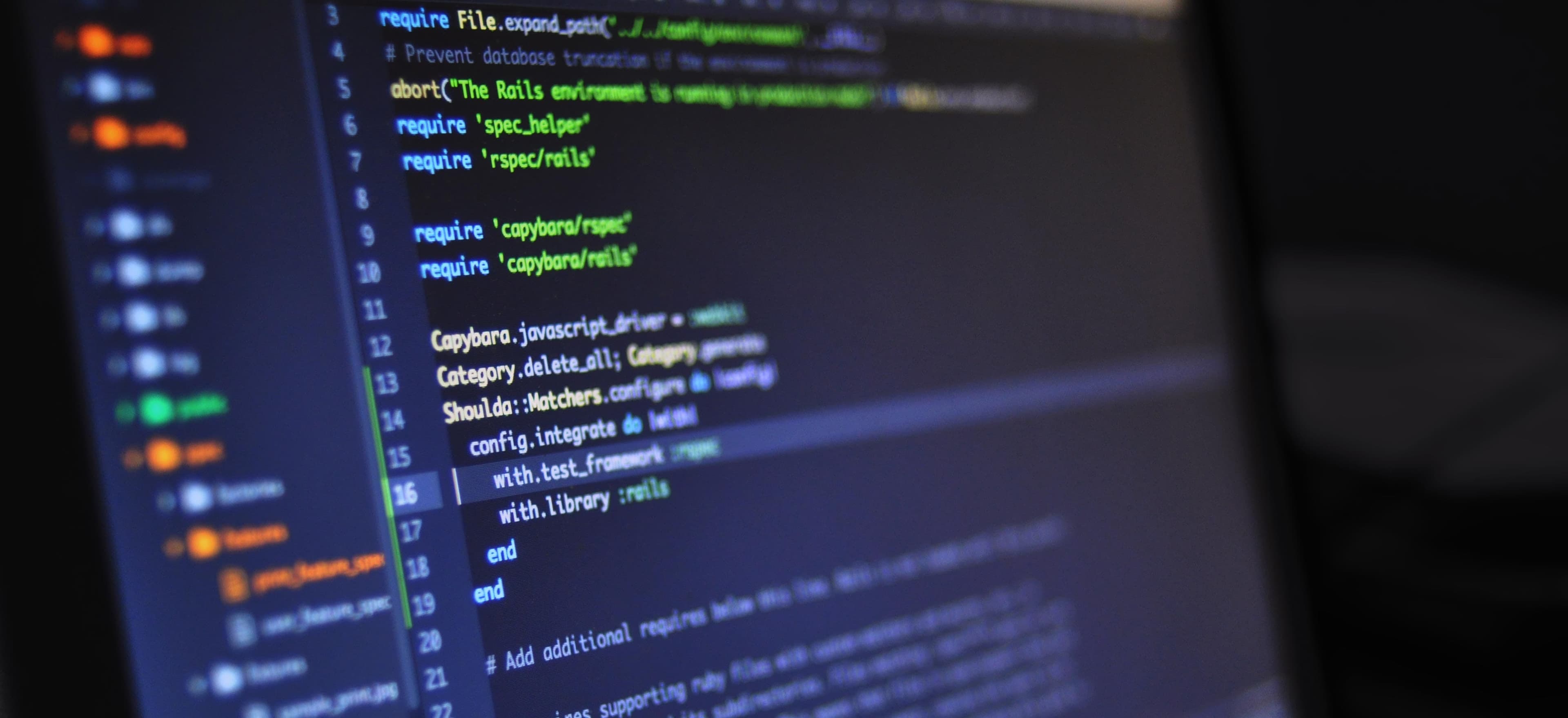
- Published on
Understanding Type Erasure: Pitfalls in Java Generics
Java's generics, introduced in Java 5, provide a powerful way to ensure type safety at compile time. However, they come with a significant underlying implementation detail known as type erasure. This process can lead to pitfalls that developers must navigate carefully to avoid unexpected behaviors and bugs in their applications.
In this comprehensive discussion, we will explore the concept of type erasure, its implications for Java generics, and pitfalls that developers might encounter. We will also include practical examples that highlight the 'why' behind each concept.
What is Type Erasure?
Type erasure is the process by which the compiler removes all generic type information during compilation. This means that the Java Virtual Machine (JVM) does not have any knowledge of the specific types used in generics at runtime.
Why Use Generics?
Generics help improve code reusability and type safety. By allowing classes, interfaces, and methods to operate on objects of various types while providing compile-time type checking, developers can avoid ClassCastException at runtime.
How Type Erasure Works
During compilation, the Java compiler translates generics into a non-generic form. For example, List<T>
becomes List
. This means that all the generic type parameters are replaced with their bounds or Object
if there are no bounds.
Example:
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
After type erasure, the above class is transformed roughly into:
public class Box {
private Object item;
public void setItem(Object item) {
this.item = item;
}
public Object getItem() {
return item;
}
}
In this example, you can see how the generic type T
has been replaced with Object
.
Benefits and Drawbacks of Type Erasure
Benefits:
- Backward Compatibility: Type erasure allows for the inclusion of generics without breaking existing codebases that do not use them.
- Reduced Complexity: By simplifying the type system at runtime, the JVM remains efficient in managing memory and performance.
Drawbacks:
- No Runtime Type Information: Generics do not retain information about their specific type during execution.
- Type Casting Required: To retrieve the object as its actual type, developers need to perform casting, which introduces the possibility of ClassCastException.
- Cannot use Primitive Types: Generics cannot work directly with primitives; they require their wrapper classes.
These drawbacks begin to illustrate the pitfalls developers should be wary of.
Common Pitfalls of Type Erasure
- ClassCastException Risk
Consider the scenario where you have a method that returns a generic list. While the type information might seem clear, type erasure can lead to unexpected issues.
public class GenericListExample {
public static void main(String[] args) {
List<String> stringList = new ArrayList<String>();
List rawList = stringList;
rawList.add(1); // Adding an integer to a list of strings
String item = stringList.get(0); // This will throw ClassCastException at runtime
}
}
Explanation
In this code snippet, although stringList
was defined to hold String elements, rawList
allows adding any object due to type erasure. When you attempt to retrieve the item, the program will throw a ClassCastException
.
- Inability to Create Generic Arrays
Java does not allow the creation of generic arrays, mainly because of the ambiguity of the array type and potential for type safety issues.
public class ArrayGenericExample {
public static void main(String[] args) {
List<String>[] stringLists = new List<String>[10]; // Compile-time error
}
}
Explanation
This declaration will not compile because you cannot create an array of a parameterized type. One common workaround is to create an array of raw type and cast it, but this is unsafe and may lead to runtime errors later.
// Unsafe workaround
List<String>[] unsafeLists = (List<String>[]) new List[10];
- Type Parameters in Static Context
Static fields and methods cannot use type parameters from an enclosing class. Here's an example that demonstrates this.
public class Example<T> {
private static T value; // Compile-time error: static context
public static void setValue(T val) {
value = val;
}
}
Explanation
In this example, trying to declare a static variable or method with a type parameter fails because static does not associate with any instance of a class.
- Wildcard Limitations
While wildcards in generics are useful, they can introduce confusion and limitations.
public class WildcardExample {
public static void addNumbers(List<? extends Number> list) {
// list.add(new Integer(10)); // Compile-time error
}
}
Explanation
The use of ? extends Number
signifies that the method can accept a list of any subtype of Number. However, the method cannot add items to the list because the compiler cannot ensure type safety.
Best Practices to Avoid Pitfalls
To navigate the pitfalls associated with type erasure, consider the following best practices:
-
Use Generics Wisely: When possible, prefer generic collections over raw types. They provide safety and clarify intention.
-
Keep Array Usage Separate: Avoid creating arrays of generic types. Consider using collections instead.
-
Limit the Use of Wildcards: Understand the implications of using
? super T
and? extends T
. Ensure that your usage aligns with the intended behavior. -
Avoid Static Context for Generics: If you need to utilize generics, do so in instance methods or fields within the class.
-
Leverage Annotations: Use
@SuppressWarnings("unchecked")
carefully when performing unchecked casts, and document the reasoning to avoid future confusion.
The Closing Argument
Type erasure in Java is a key implementation detail behind the generics that we use to ensure type safety. While it brings significant benefits, it also introduces several pitfalls that developers should remain vigilant against. By understanding how type erasure works and utilizing best practices, you can avoid common mistakes and write more robust Java applications.
For further reading on Java generics and type safety, check out the official Java Documentation on Generics and the insightful article on Type Erasure.
Understanding these concepts not only improves your coding skills but also enhances your ability to design systems that are efficient, safe, and maintainable.
References
- Effective Java by Joshua Bloch
- Java Generics and Collections by Maurice Naftalin
By keeping these discussions in mind, you can harness the power of Java generics while steering clear of their inherent complexities.