Mastering Garbage Collection Tuning for Low-Latency Java Apps
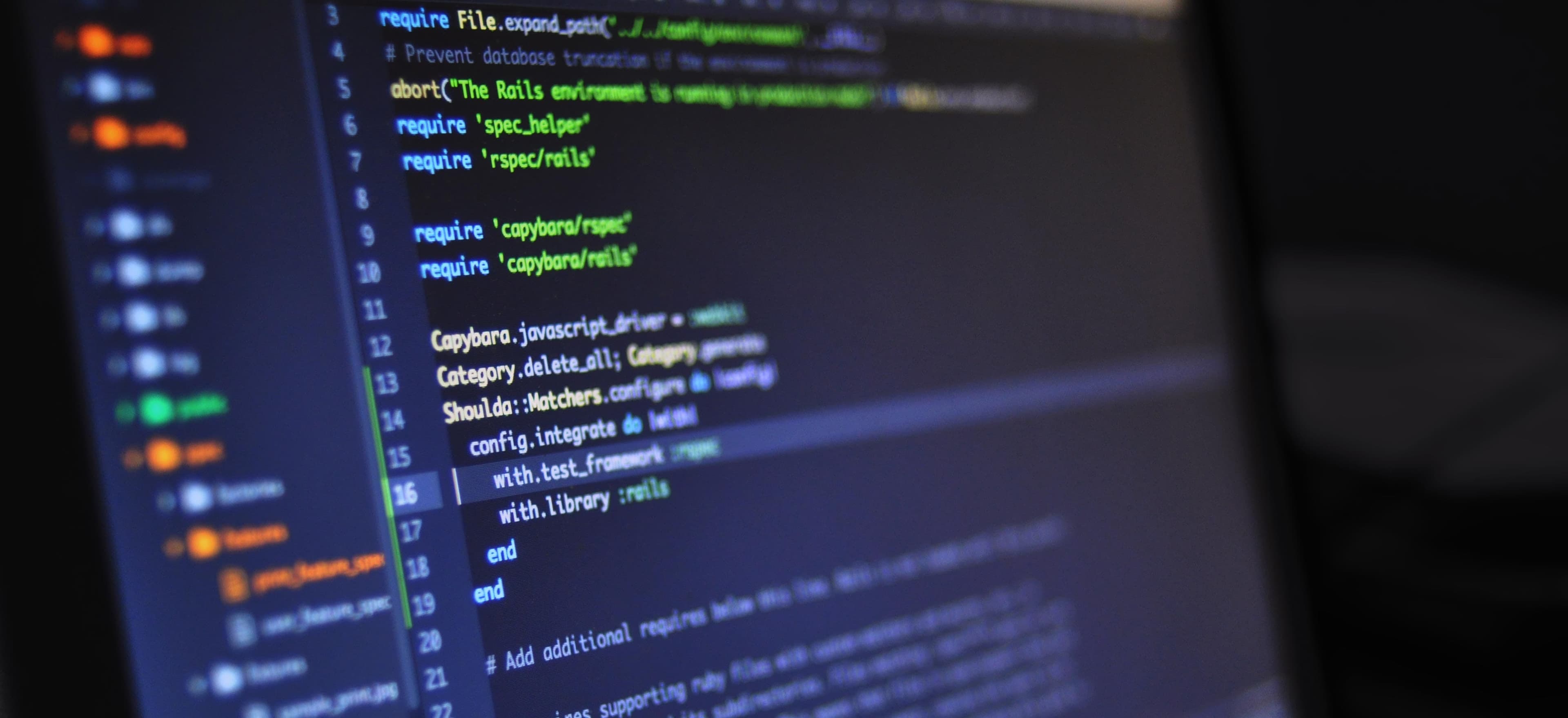
- Published on
Mastering Garbage Collection Tuning for Low-Latency Java Apps
Java's garbage collection (GC) plays a crucial role in memory management, automatically reclaiming memory used by objects that are no longer needed. While the automatic nature of garbage collection is beneficial for many applications, it can introduce latency issues that are particularly problematic for low-latency applications, such as financial trading systems or real-time data processing.
In this blog post, we will explore ways to optimize garbage collection for low-latency Java applications. We will discuss the various garbage collectors offered by the Java Virtual Machine (JVM), tuning techniques, and clear code examples demonstrating these concepts in action.
Understanding Garbage Collection
Before diving into tuning, we need to understand the basic workings of garbage collection in Java. The JVM employs several garbage collectors, each with its own algorithm for memory management.
Major Garbage Collection Algorithms
- Serial GC: A single-threaded collector best suited for small applications with low memory requirements.
- Parallel GC: Utilizes multiple threads for garbage collection, making it more suitable for application with medium to large heap sizes.
- Concurrent Mark-Sweep (CMS) GC: Designed for low-latency trades, minimizes pause times but can run into fragmentation issues.
- G1 (Garbage-First) GC: A low-pause collector that divides the heap into regions, which helps in claiming object space more efficiently.
- ZGC and Shenandoah: Low-latency garbage collectors released in Java 11 and Java 13, respectively, designed to manage memory with minimal pauses.
Choosing the Right Collector
Choosing the right garbage collector is crucial for application performance. For low-latency applications, G1, CMS, or the latest low-latency options (ZGC, Shenandoah) are preferred due to their reduced pause times.
Analyzing Your Application’s GC Behavior
You can gather valuable statistics on garbage collection behavior using JVM options. For instance, the following command can be added when you run your application:
java -Xlog:gc*:file=gc.log:time -XX:+PrintGCDetails -XX:+PrintGCTimeStamps -jar your-java-app.jar
This command logs detailed information about garbage collection events into gc.log
, allowing you to monitor and analyze application performance.
Tuning Strategies
Once you have chosen the appropriate garbage collector, the next step is to optimize it for your specific application. Here are several strategies for tuning garbage collection:
1. Heap Size Configuration
Setting the right heap size is essential. A too-small heap may cause excessive GC activity, while a too-large heap may increase the pause time due to the longer garbage collection cycles.
java -Xms512M -Xmx2G -jar your-java-app.jar
In this example, we set the initial heap size (-Xms) to 512 MB and the maximum heap size (-Xmx) to 2 GB. This configuration provides a balance that can help minimize GCs.
2. Garbage Collector Flags
Once you have selected the garbage collector, you can further adjust its behavior using specific flags.
For G1 GC, you might use:
java -XX:+UseG1GC -XX:MaxGCPauseMillis=100 -XX:InitiatingHeapOccupancyPercent=45 -jar your-java-app.jar
These flags do the following:
- MaxGCPauseMillis: Aims to keep the max pause time under 100 milliseconds.
- InitiatingHeapOccupancyPercent: Starts concurrent marking when the heap is 45% occupied.
3. Tuning the Young Generation
In a typical garbage collector, memory is divided into three generations: young, old, and permanent. Tuning the young generation size can have a significant impact on application performance, especially in low-latency systems.
java -XX:NewRatio=3 -jar your-java-app.jar
Here, we set the new ratio, which means the young generation will occupy 25% of the total heap space, providing a good balance for applications with a lot of short-lived objects. Adjust this according to your application needs.
4. Logging and Monitoring
You can gain insights into the effectiveness of your garbage collection tuning using the GC log files. You may want to look for
- Frequency of Full GCs
- Total pause time caused by GC
- Memory trends
Using tools like VisualVM or Java Mission Control can help visualize GC performance and behavior over time.
Example Code for Monitoring
To demonstrate a simple application that can help monitor GC behavior, consider the following code snippet:
public class GCDemo {
public static void main(String[] args) {
for (int i = 0; i < 100000; i++) {
String str = new String("String " + i);
// Simulating workload
if (i % 1000 == 0) {
System.gc(); // Trigger garbage collection manually for demonstration
}
}
}
}
This code snippet creates a considerable number of string objects. The call to System.gc()
is usually not recommended for production but is shown here for demonstration purposes.
Closing Remarks
Tuning garbage collection for low-latency Java applications can significantly improve performance and responsiveness. By carefully selecting the right garbage collector, configuring heap sizes, and utilizing various flags, developers can achieve optimal memory management in demanding environments.
The topic of garbage collection is complex and often requires iterative profiling and tuning to get truly ideal performance results. Consider integrating monitoring tools into your development process and analyze GC logs for better insights.
For more in-depth insights into Java performance, check out Java Performance Tuning for a comprehensive guide.
As always, testing and profiling in your specific context are key to achieving the desired latency and responsiveness. Happy tuning!