Preventing Java Primitive Type Overflows: Essential Tips
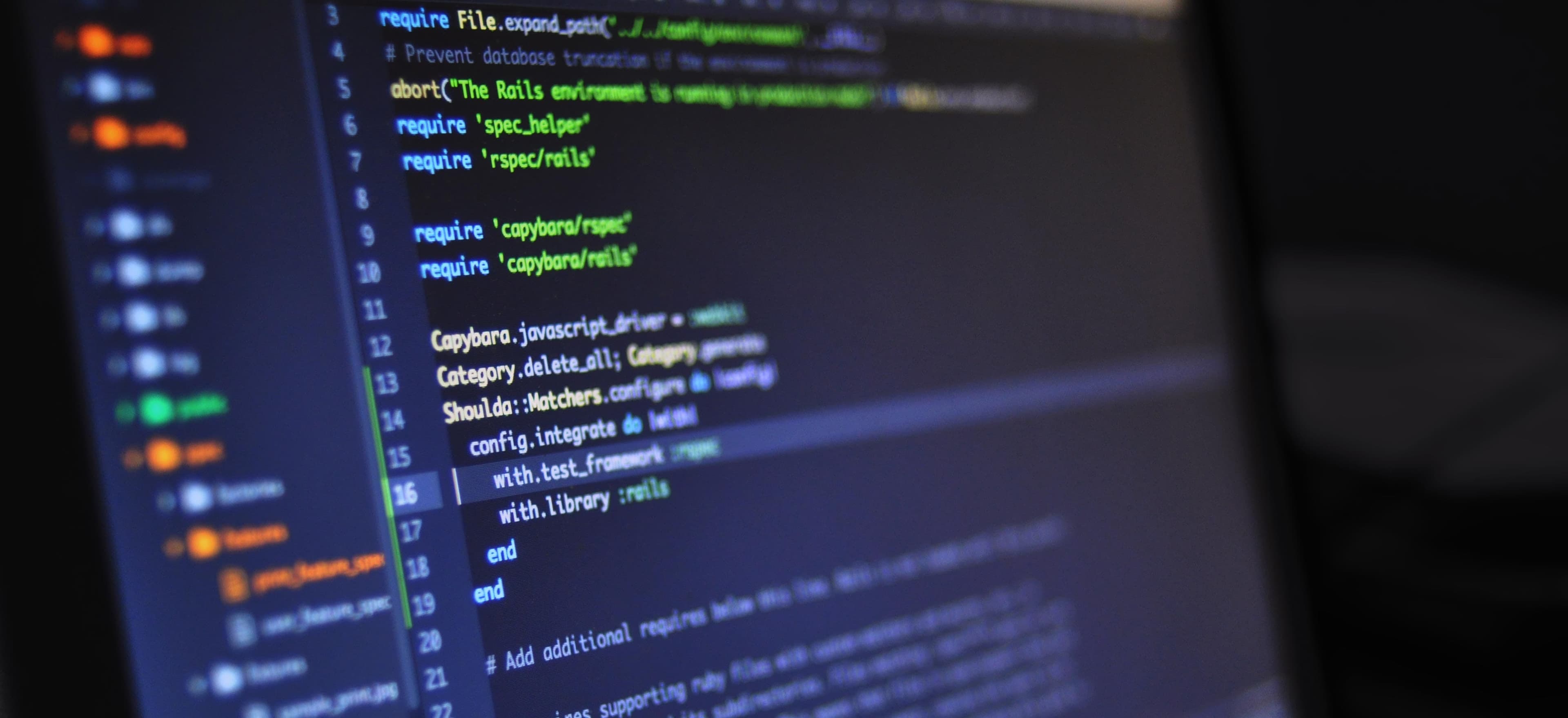
- Published on
Preventing Java Primitive Type Overflows: Essential Tips
Java is a versatile programming language, but it does come with its own set of challenges, notably in managing primitive types. One such challenge is the potential for type overflow. A primitive type overflow occurs when a value exceeds the maximum limit of its designated data type, wrapping around and starting again from the minimum value. This can lead to unexpected errors and security vulnerabilities in your applications.
In this blog post, we will delve into the nuances of primitive type overflows in Java, explore practical strategies to prevent them, and include code snippets that illustrate these concepts with clarity. Let's stay informed and write error-free Java code!
Understanding Primitive Types and Their Limits
Java has several primitive types, each with defined ranges:
- byte: -128 to 127
- short: -32,768 to 32,767
- int: -2,147,483,648 to 2,147,483,647
- long: -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
- float and double: These are for floating-point numbers but can also overflow significantly due to their precision limits.
Failing to account for these limits can lead to issues such as incorrect calculations, application crashes, and vulnerabilities to attacks like denial-of-service.
The Problem with Overflows
When an overflow occurs, Java does not throw an error by default. Instead, it simply wraps the number around. For example, adding one to Integer.MAX_VALUE
results in Integer.MIN_VALUE
, as shown below:
public class OverflowExample {
public static void main(String[] args) {
int maxValue = Integer.MAX_VALUE;
int overflow = maxValue + 1; // Overflow occurs
System.out.println("Max Value: " + maxValue);
System.out.println("Overflow Value: " + overflow); // Outputs -2147483648
}
}
This can be dangerous, especially in financial applications or any software where data integrity is crucial.
Essential Tips to Prevent Primitive Type Overflows
1. Use Java's Built-In Methods
Java provides Math
class methods that can help prevent overflow:
int a = Integer.MAX_VALUE;
int b = 1;
int result = Math.addExact(a, b);
The Math.addExact
method throws an ArithmeticException
if an overflow occurs, offering a safety net for your code.
2. Perform Range Checks Before Calculations
Always check if the expected operations might lead to overflow:
public class RangeCheckExample {
public static void main(String[] args) {
int a = Integer.MAX_VALUE;
int b = 1;
if (a > Integer.MAX_VALUE - b) {
System.out.println("Addition would overflow!");
} else {
System.out.println("Result: " + (a + b));
}
}
}
By implementing range checks, you can make informed decisions about whether to proceed with calculations.
3. Utilize Larger Data Types
Sometimes, the simplest solution is to use a larger data type:
public class TypeAdjustmentExample {
public static void main(String[] args) {
int a = Integer.MAX_VALUE;
int b = 1;
long result = (long) a + (long) b; // Switch to long
if (result > Integer.MAX_VALUE) {
System.out.println("Overflow occurs if treated as int, result is: " + result);
} else {
System.out.println("Result: " + (int) result);
}
}
}
While this may not always be the most memory-efficient approach, it can safeguard against overflow in critical calculations.
4. Leverage Java 8's Optional
and Streams
Using Java 8 features can also help manage overflow:
import java.util.OptionalInt;
public class OptionalExample {
public static void main(String[] args) {
OptionalInt result = OptionalInt.of(Integer.MAX_VALUE).map(a -> a + 1);
result.ifPresentOrElse(
value -> System.out.println("Result: " + value),
() -> System.out.println("Overflow detected!")
);
}
}
This method allows for a functional approach that makes it clear when an overflow occurs.
5. Implement Thread-safe Operations
If your program is multi-threaded, ensure that your calculations are thread-safe to avoid race conditions, which could also lead to overflows.
import java.util.concurrent.atomic.AtomicInteger;
public class ThreadSafeExample {
private static AtomicInteger counter = new AtomicInteger(0);
public static void main(String[] args) {
// Start multiple threads that increment the counter
for (int i = 0; i < 10; i++) {
new Thread(() -> {
System.out.println("Incremented: " + counter.incrementAndGet());
}).start();
}
}
}
Using AtomicInteger
helps maintain integrity in a multi-threaded environment.
6. Educate Your Users
Sometimes, the best way to prevent overflows is through user education. Provide clear documentation on the limits of your application. Help users understand their input constraints to avoid unintentional overflow scenarios.
Bringing It All Together
Primitive type overflows can introduce serious bugs and vulnerabilities into your Java applications. By adopting best practices such as using built-in methods, performing range checks, leveraging larger data types, incorporating Java 8 features, ensuring thread safety, and educating users, you can mitigate the risks associated with primitive type overflows effectively.
Always prioritize data integrity, especially when handling critical calculations or sensitive information. Applying these techniques will not only help you avoid overflow-related issues but also enhance your skills as a proficient Java developer.
For further reading on managing numeric types in Java, consider these resources:
- Java Primitive Types | Oracle Documentation
- Understanding Java Math Functions
- Java Concurrency in Practice
Happy coding! And may your numbers always remain in range!
Checkout our other articles