Mastering Java Enum: Avoiding Fallthrough in Switch Cases
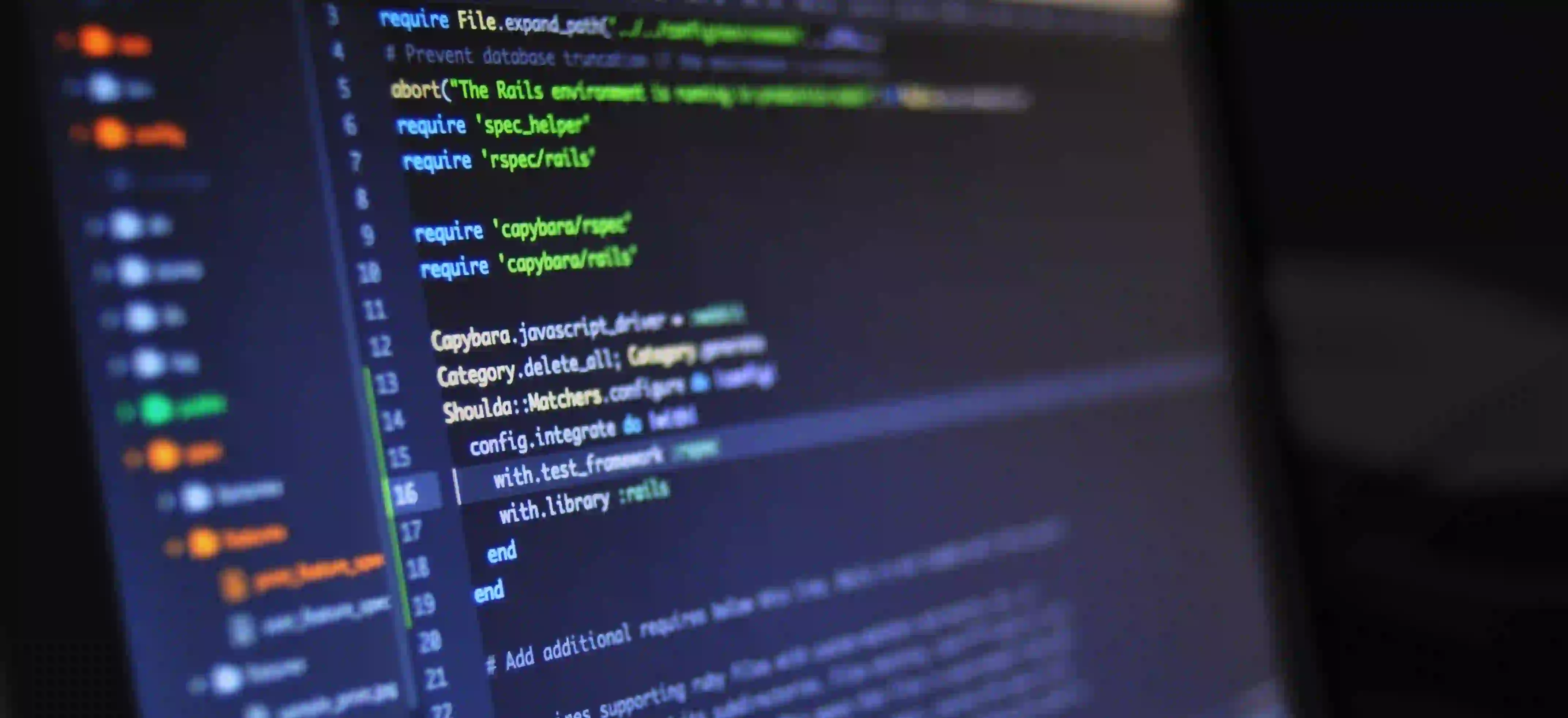
Mastering Java Enum: Avoiding Fallthrough in Switch Cases
Enums in Java are powerful tools that enhance type safety and code readability. They are often used in switch cases, but developers sometimes encounter the problem of fallthrough, especially when multiple enum constants share a code block. In this post, we will explore how to master Java enums while ensuring we avoid fallthrough in switch statements.
What is an Enum in Java?
An enum (short for enumeration) is a special Java type used to define collections of constants. It adds clarity and type safety to code, making it easier for developers to read and maintain. Each enum constant is an instance of its enum type.
Enums help in avoiding hard-coded values, leading to cleaner, more maintainable code. For example, consider the following enum definition:
public enum Day {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY
}
While working with enums, especially inside switch statements, developers need to be careful with fallthrough, which can lead to unexpected behavior.
The Fallthrough Problem in Switch Statements
Fallthrough occurs when the control flows from one case to the next without hitting a break statement. This means that all subsequent case blocks get executed, which might not be the intended behavior.
Example of Fallthrough in Switch Cases
Let’s examine an example involving an enum:
public enum TrafficLight {
RED,
YELLOW,
GREEN
}
public class TrafficControl {
public void getAction(TrafficLight light) {
switch (light) {
case RED:
System.out.println("Stop!");
case YELLOW:
System.out.println("Caution!");
case GREEN:
System.out.println("Go!");
}
}
}
If we call getAction(TrafficLight.RED)
, the output will be:
Stop!
Caution!
Go!
This happens because there are no break statements present. Consequently, the program continues executing all subsequent cases.
How to Prevent Fallthrough
To prevent fallthrough, you should always include a break statement at the end of each case block. This approach ensures that once the execution leaves a case, it does not enter the next case unintentionally.
Revised Example
Here’s a revised version of our previous example that prevents fallthrough:
public class TrafficControl {
public void getAction(TrafficLight light) {
switch (light) {
case RED:
System.out.println("Stop!");
break; // Prevents fallthrough
case YELLOW:
System.out.println("Caution!");
break; // Prevents fallthrough
case GREEN:
System.out.println("Go!");
break; // Prevents fallthrough
}
}
}
With this modification, if you call getAction(TrafficLight.RED)
, the output will be:
Stop!
Understanding Break Statements
The break statement in Java is essential within switch cases. It terminates the switch statement and transfers control to the next line of code following the switch block. Without the break statement, all code in subsequent cases will be executed.
Best Practices for Using Enums in Switch Statements
-
Always Use Break Statements: Include break statements for each case to prevent accidental fallthrough.
-
Organize Cases Logically: Group similar cases together to enhance readability and understanding.
-
Default Case: Always consider implementing a default case. This is a great way to handle unexpected values.
☕snippet.javapublic void getAction(TrafficLight light) { switch (light) { case RED: System.out.println("Stop!"); break; case YELLOW: System.out.println("Caution!"); break; case GREEN: System.out.println("Go!"); break; default: System.out.println("Invalid light!"); } }
-
Use Enum Methods: Enums allow us to define methods and fields. Consider using them for shared behavior or properties.
Enum Methods Example
public enum TrafficLight {
RED("Stop!"),
YELLOW("Caution!"),
GREEN("Go!");
private final String action;
TrafficLight(String action) {
this.action = action;
}
public String getAction() {
return action;
}
}
public class TrafficControl {
public void getAction(TrafficLight light) {
System.out.println(light.getAction());
}
}
In this example, instead of using a switch case, we call the getAction
method directly on the enum, resulting in cleaner and more maintainable code.
Closing the Chapter
Enums in Java can significantly enhance your code’s readability and maintainability. However, when using switch statements with enums, be wary of fallthrough and always implement good practices.
By following the guidelines provided in this post, you can master Java enums and improve your programming efficiency. Always remember to check out the Java documentation for more insights on enums.
In your next Java project, take the opportunity to leverage enums while ensuring you avoid the pitfalls of fallthrough in switch statements. Happy coding!