Mastering Edge Cases in Java String Comparisons
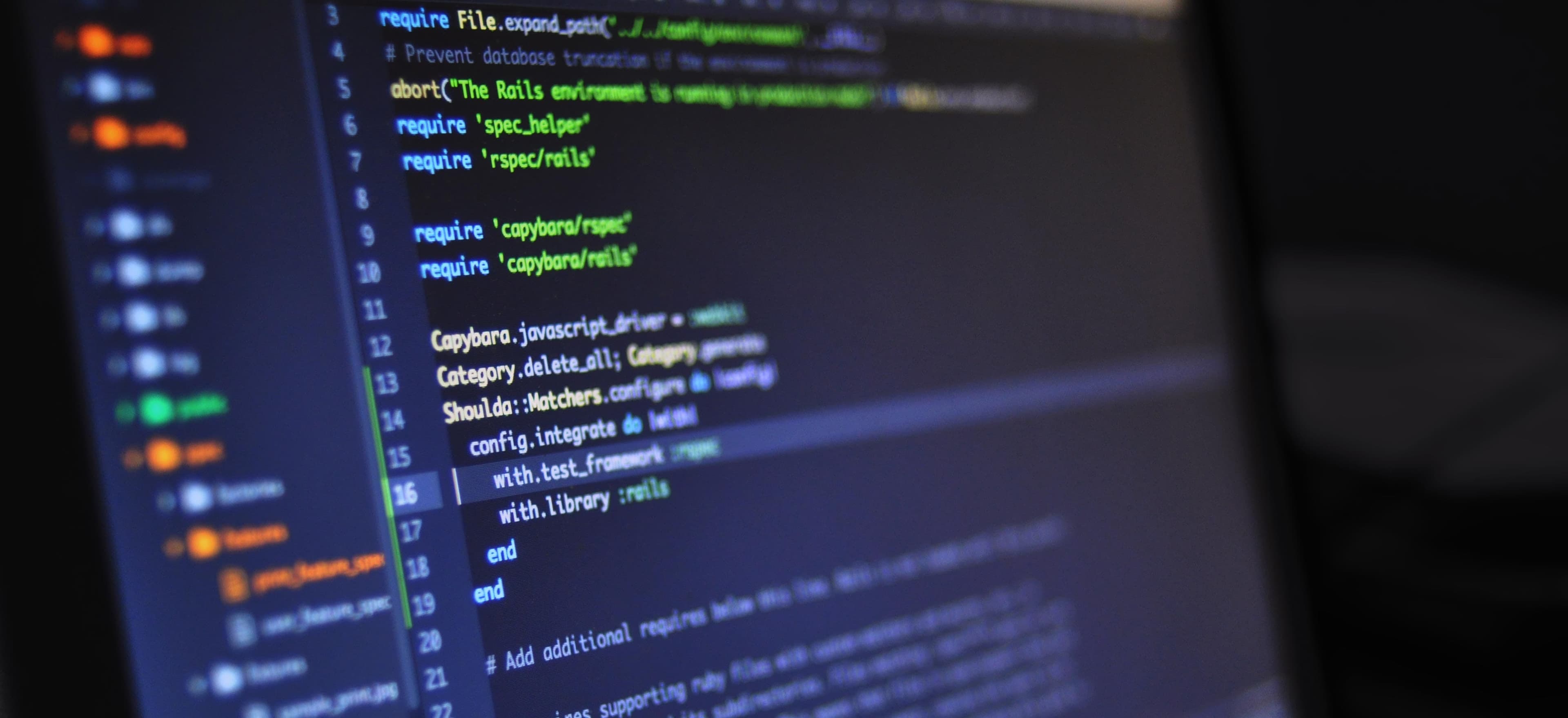
- Published on
Mastering Edge Cases in Java String Comparisons
In programming, comparing strings may seem straightforward at first glance. However, as anyone who has delved deeper into Java can attest, it can quickly become a tangled web of nuances, especially when edge cases come into play. In this post, we will explore how to handle string comparison in Java, delve into common edge cases, and provide illustrative code snippets to help you master this essential skill.
Understanding String Comparison in Java
Equality vs Identity
In Java, it's critical to distinguish between the equality operator (==) and the .equals()
method. The operator checks whether two references point to the same object in memory, while .equals()
checks for logical equality—the actual content of the strings.
Let's look at a simple code example to illustrate this:
String str1 = new String("hello");
String str2 = new String("hello");
if (str1 == str2) {
System.out.println("str1 and str2 reference the same object."); // This will not execute
} else {
System.out.println("str1 and str2 are different objects."); // This will execute
}
if (str1.equals(str2)) {
System.out.println("str1 and str2 have the same content."); // This will execute
}
The Importance of .equalsIgnoreCase()
When comparing strings that may differ by case, using .equalsIgnoreCase()
can be immensely beneficial. This method allows you to compare strings in a case-insensitive manner, which could be crucial in scenarios like user authentication or data normalization.
Consider the following code:
String userInput = "JAVA";
String target = "java";
if (userInput.equalsIgnoreCase(target)) {
System.out.println("User input matches the target string (case insensitive).");
} else {
System.out.println("User input does not match the target string.");
}
Common Edge Cases in String Comparison
1. Null Check Before Comparison
One of the most common pitfalls in string comparison is neglecting null checks. Attempting to call methods on a null reference will result in a NullPointerException
.
String a = null;
String b = "hello";
if (b != null && b.equals(a)) {
System.out.println("b matches a.");
} else {
System.out.println("Either b is not equal to a or a is null."); // This will execute
}
In the above example, we first check if b
is not null before making the comparison, thereby avoiding a potential error.
2. Whitespace Sensitivity
Another subtlety in string comparison is the sensitivity to whitespace. Two strings that look identical may differ if one has extra spaces.
String str1 = "hello";
String str2 = " hello ";
if (str1.trim().equals(str2.trim())) {
System.out.println("The strings are equal after trimming whitespace.");
} else {
System.out.println("The strings are not equal."); // This will execute
}
Using .trim()
can help standardize strings by removing leading and trailing whitespace, making comparisons more reliable.
3. Unicode and Locale Considerations
Strings in Java are Unicode-compliant, which introduces additional complexity for certain languages and symbols. For instance, the English letter "a" is not the same as the accented "à". You must carefully consider locale when performing string comparisons in internationalized applications.
Utilizing Collator
can help ensure proper comparison based on linguistic rules:
import java.text.Collator;
import java.util.Locale;
Collator collator = Collator.getInstance(Locale.FRENCH);
String str1 = "école";
String str2 = "ecole";
if (collator.compare(str1, str2) == 0) {
System.out.println("Strings are considered equal in French locale.");
} else {
System.out.println("Strings are not equal in French locale."); // This will execute
}
By taking locale into account, you can develop applications that are more user-friendly across different cultures.
Advanced Techniques for String Comparison
String Pool and Interning
Java maintains a string pool to optimize memory usage. Strings literals such as "hello"
are stored in this pool. When you create a string using new String("hello")
, Java creates a new object instead of referencing the pool. Interning can help mitigate this:
String str1 = "hello";
String str2 = new String("hello").intern(); // Retrieves existing value from the pool
if (str1 == str2) {
System.out.println("Both strings point to the same memory address in the pool."); // This will execute
}
Using .intern()
ensures that the string created with new
will reference the pooled instance.
Using StringBuilder for Complex Comparisons
When dealing with complex manipulations before comparison, it's often better to use a StringBuilder
due to its performance benefits in mutable string operations.
StringBuilder strBuilder1 = new StringBuilder("hello");
StringBuilder strBuilder2 = new StringBuilder("hello");
if (strBuilder1.toString().equals(strBuilder2.toString())) {
System.out.println("Both StringBuilders contain the same content.");
} else {
System.out.println("The contents are different.");
}
Although the comparison uses .toString()
, StringBuilder
allows you to append or modify strings efficiently.
Final Considerations
Mastering edge cases in Java string comparisons is not just about understanding the technical aspects—it's also about recognizing common pitfalls and applying best practices to minimize errors in your code. By becoming familiar with string equality checks, null handling, whitespace sensitivity, locale considerations, and advanced techniques like string interning and StringBuilder
, you can write more robust Java applications.
For further reading, consider checking out Java String Documentation and String Comparison Techniques to keep abreast of the latest best practices and methods.
Happy coding, and may your string comparisons be swift and error-free!
Checkout our other articles