Mastering Volatile Variables in Java Multithreading
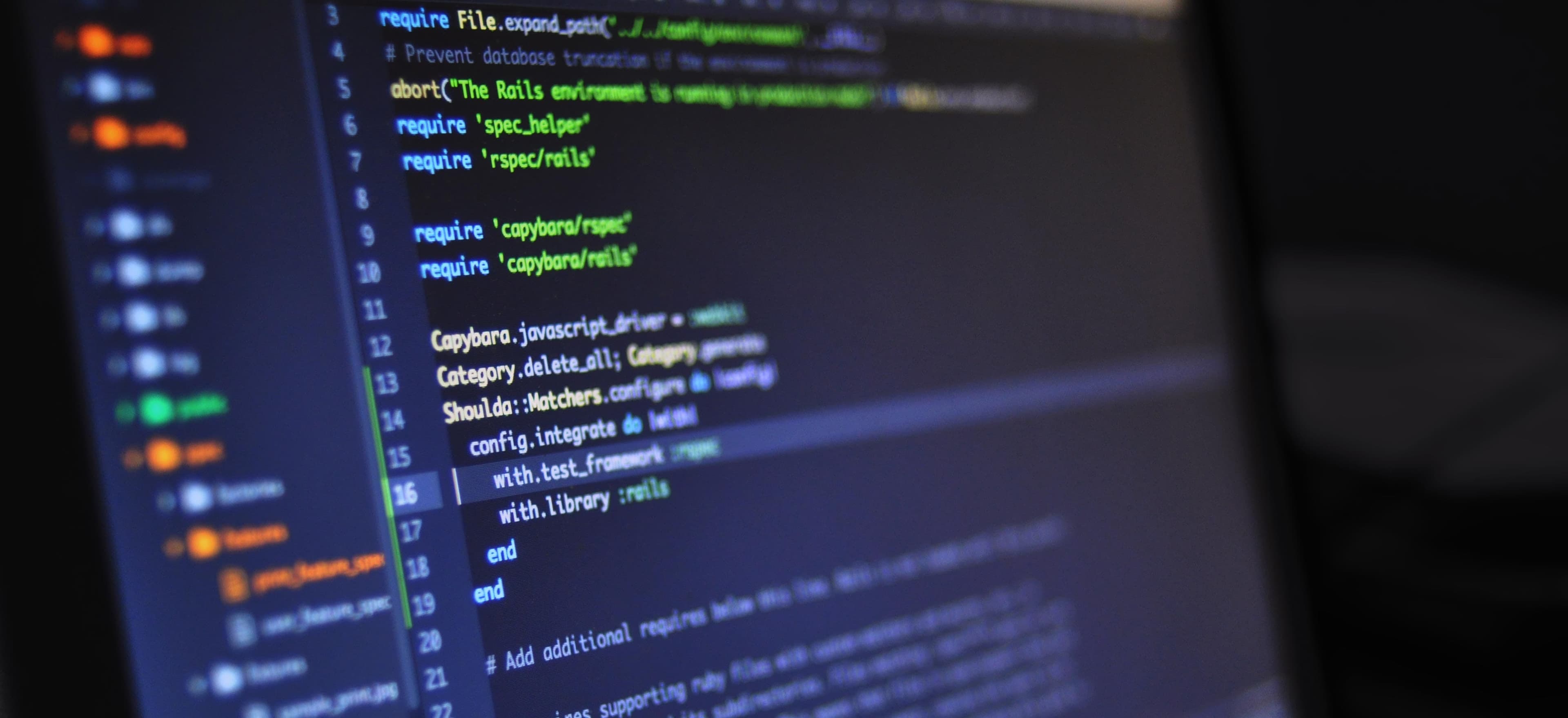
- Published on
Mastering Volatile Variables in Java Multithreading
In the world of Java programming, particularly in the realm of multithreading, ensuring correctness and efficiency is critical. When threads interact with shared data, you may encounter issues like visibility and atomicity. Luckily, Java provides several tools, one of which is the volatile
keyword. This post will delve deep into understanding the volatile
keyword and how to master its use in your multithreaded applications.
Understanding Multithreading
Java allows multiple threads to run concurrently, helping improve application performance. However, with this concurrency comes challenges such as race conditions, thread safety, and visibility of shared variables. Race conditions occur when two or more threads attempt to modify a variable simultaneously, causing unpredictable behavior.
Here’s a straightforward example of a thread that increments a counter:
class Counter {
private int counter = 0;
public void increment() {
counter++;
}
public int getValue() {
return counter;
}
}
In a multithreading environment, invoking increment()
from multiple threads might lead to incorrect results. This problem illustrates the need for proper handling of shared variables.
What is volatile
?
The volatile
keyword is a special modifier that can be applied to a variable. Declaring a variable as volatile
ensures that any thread reading that variable sees the most recent write made by any other thread. This is particularly important when dealing with situations where one or more threads may modify the variable.
Key Characteristics of volatile
-
Visibility: Changes made to a
volatile
variable by one thread are immediately visible to other threads. This prevents the caching of variables in thread local memory. -
Atomicity: While
volatile
guarantees visibility, it does not guarantee atomicity. This means that operations that are not atomic (like incrementing a variable) still require additional synchronization. -
Ordering: The
volatile
keyword enforces a happens-before relationship, which ensures that code preceding a write to avolatile
variable is visible to any subsequent read of that variable by another thread.
Example of volatile
Usage
Here is a simple example showcasing how the volatile
modifier works:
class VolatileExample {
private volatile boolean running = true;
public void run() {
while (running) {
// Perform some operation
}
System.out.println("Stopped running.");
}
public void stop() {
running = false;
}
}
In this example, the running
variable is declared as volatile
. The run()
method continuously executes while running
is true
. When we call the stop()
method, the change to running
is immediately visible to the thread executing run()
.
Why Use volatile
?
-
Performance: Using
volatile
can be more efficient than synchronization blocks, as it avoids the overhead of acquiring and releasing locks. -
Simplicity: In cases where you only need visibility (not atomic operations),
volatile
offers a simpler alternative to more complex synchronization methods.
When to Use volatile
- When you have a flag or state variable that is accessed by multiple threads and only needs to be read and written.
- When you want to ensure a variable’s visibility without requiring a full lock or synchronization overhead.
Keep in mind that volatile
should not be used as a replacement for comprehensive synchronization and locking mechanisms such as synchronized
methods or blocks when atomic operations are involved.
Volatile Variables in Practice
Let’s explore a more comprehensive example to demonstrate the use of volatile
.
A Flip-Flop Example
Consider an application where threads toggle between two states based on a boolean flag.
class FlipFlop {
private volatile boolean flag = false;
public void flip() {
for (int i = 0; i < 5; i++) {
flag = !flag; // Change the state
System.out.println("Flag is now: " + flag);
try {
Thread.sleep(500); // Simulate work
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
public void printState() {
while (true) {
System.out.println("Current Flag State: " + flag);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
break;
}
}
}
}
Setting Up Threads
You can utilize this class by running two threads in parallel: one for flipping the flag and another for printing the current state.
public class VolatileDemo {
public static void main(String[] args) {
FlipFlop flipFlop = new FlipFlop();
Thread flipper = new Thread(flipFlop::flip);
Thread printer = new Thread(flipFlop::printState);
flipper.start();
printer.start();
}
}
Explanation
In the flip()
method, we toggle the flag
every half second, simulating some workload. The printState()
method continuously prints the current state of the flag
. Because flag
is declared volatile
, any updates made to flag
in flip()
will be immediately visible to printState()
.
Limitations of volatile
It is crucial to recognize that while volatile
is powerful, it does have limitations. Here are a few:
- Cannot replace all synchronization: For complex operations involving multiple variables,
volatile
will not suffice. - No atomic operations: If your operations require multiple steps (like reading and then writing), consider using locks.
Best Practices
-
Use with care: Reserve
volatile
for scenarios that truly require it—mainly for flags and state indicators. -
Complement with other mechanisms: If you require atomicity, don’t hesitate to use locks in conjunction with
volatile
. -
Read and Write Considerations: Always consider the read and write frequency. If writes are more frequent,
volatile
may introduce performance overhead. -
Test Thoroughly: Multithreaded applications can be notoriously tricky. Make sure to test your code thoroughly to catch potential issues early.
In Conclusion, Here is What Matters
The volatile
keyword is a powerful tool in Java that can alleviate some of the complexities of multithreaded programming. By ensuring visibility of shared variables across threads, you can prevent certain concurrency issues. However, remember its limitations; volatile
is not a one-size-fits-all solution.
For more on Java multithreading, consider exploring the Java concurrency tutorial from Oracle.
Master volatile
, and you'll improve your Java programming skills, particularly in multithreading contexts. Happy coding!