Preventing Java ClassLoader Memory Leaks in Your Applications
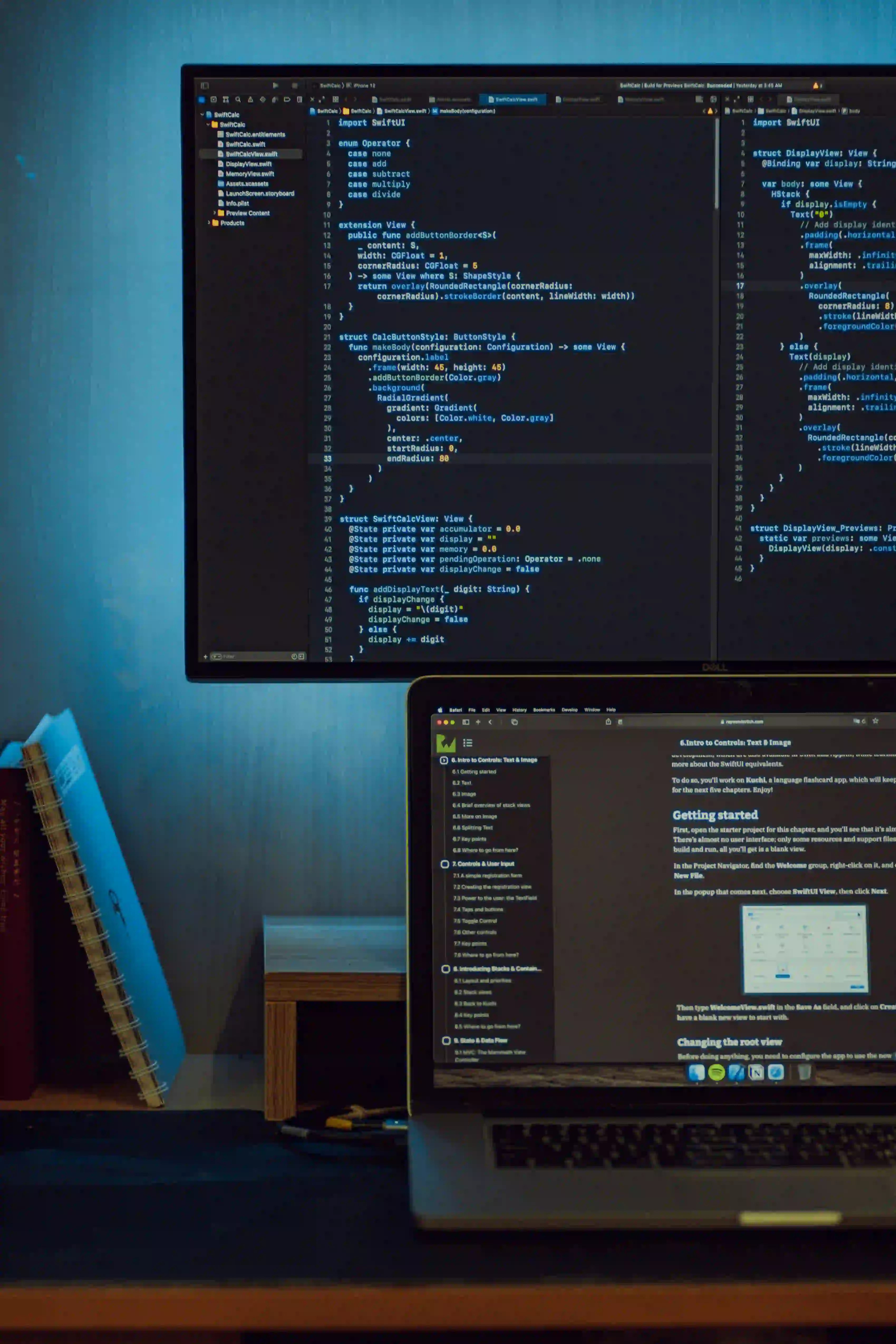
Preventing Java ClassLoader Memory Leaks in Your Applications
In the world of Java development, one of the often-overlooked challenges is memory management, specifically regarding ClassLoaders. Memory leaks caused by ClassLoader issues can lead to significant performance problems and application failures. This blog post aims to shed light on the mechanisms behind ClassLoaders, how they can leak memory, and strategies for preventing these leaks.
Understanding ClassLoaders
Before diving into memory leaks, let's understand what ClassLoaders are. In Java, a ClassLoader is a part of the Java Runtime Environment (JRE) responsible for loading classes into memory. When a Java application starts, several ClassLoaders are employed:
- Bootstrap ClassLoader: Loads core Java classes from the JDK.
- Extension ClassLoader: Loads classes from the Java Extensions directory.
- Application ClassLoader: Loads classes from the application classpath.
Why ClassLoader Leaks Occur
Memory leaks involving ClassLoaders typically arise in environments with dynamic class loading and unloading, such as web applications running on application servers (e.g., Tomcat, JBoss). Common causes include:
- Static References: Classes loaded by a ClassLoader are not released from memory if there are static references to them.
- Thread Locals: Using thread-local variables can prevent cleanup when Classes remain linked to executing threads.
- Third-party Libraries: Libraries may inadvertently maintain references to classes loaded by their own ClassLoaders.
Signs of ClassLoader Memory Leaks
Detecting ClassLoader leaks can be tricky. However, some classic symptoms indicate that your application might be experiencing a leak:
- Increased memory usage over time without release.
- OutOfMemoryError exceptions related to too many class definitions.
- Extended garbage collection pauses or frequent full GCs.
Monitoring Tools
To address these symptoms proactively, consider utilizing monitoring and analysis tools. Some popular choices include:
- VisualVM: A monitoring tool that provides detailed information about heap memory settings and ClassLoader statistics.
- JProfiler: A commercial product offering in-depth analysis, including memory leak detection.
- Eclipse Memory Analyzer (MAT): A powerful tool for analyzing memory dumps.
Preventing Memory Leaks
The key to preventing memory leaks caused by ClassLoader issues includes following best practices when loading classes and managing their lifecycle.
1. Avoid Static References
Static references outlive the ClassLoader that loaded them. Consider the following example:
public class Singleton {
private static Singleton instance = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return instance;
}
}
By holding a static instance, this Singleton class prevents its ClassLoader from being garbage-collected, leading to potential memory leaks. Instead, you should use the following approach:
public class Reusable {
private Reusable() {}
public static Reusable createInstance() {
return new Reusable();
}
}
In this design, ClassLoaders can be cleaned up after use.
2. Dereference Class-level Cache
If you're caching instances at the class level, always ensure to nullify references once they are no longer needed. For example:
public class CachingService {
private static Map<String, MyObject> cache = new HashMap<>();
public MyObject getCachedObject(String key) {
return cache.get(key);
}
public void clearCache() {
cache.clear();
}
}
In this code, always call clearCache
at the appropriate lifecycle phase (e.g., on application shutdown). This way, the entries will be released, allowing for the Collection and its ClassLoader to be garbage-collected.
3. Use Weak References
For caching scenarios, using WeakReference
can ensure that the cached object does not prevent the ClassLoader from being collected. Here’s a snippet:
import java.lang.ref.WeakReference;
import java.util.Map;
import java.util.HashMap;
public class WeakCache {
private Map<String, WeakReference<MyObject>> cache = new HashMap<>();
public void put(String key, MyObject value) {
cache.put(key, new WeakReference<>(value));
}
public MyObject get(String key) {
WeakReference<MyObject> ref = cache.get(key);
return ref == null ? null : ref.get();
}
}
In this implementation, if no strong references exist for MyObject
, it can be garbage-collected, preventing memory leaks.
4. Clean Up Thread Locals
Thread-local variables maintain a reference to their values for the duration of their thread's lifecycle. If you are using ThreadLocal
, make sure to clean up on thread pool shutdown:
public class ThreadLocalExample {
private static ThreadLocal<MyObject> threadLocal = new ThreadLocal<>();
public static void set(MyObject obj) {
threadLocal.set(obj);
}
public static void clear() {
threadLocal.remove();
}
}
Always call the clear()
method when a thread finishes its work.
5. Class Loader Context Management
When using custom ClassLoaders, ensure they are properly managed. If you create a ClassLoader:
public class CustomClassLoader extends ClassLoader {
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
// Class loading logic
}
}
Always ensure to release references to any classes you load. Employing a unique ClassLoader per deployment can help isolate references.
6. Use ClassLoader Hierarchy
Understanding and correctly leveraging the ClassLoader hierarchy can prevent leaks. Classes should ideally be loaded by the parent ClassLoader whenever possible. This can be accomplished like this:
public class MyClassLoader extends ClassLoader {
@Override
protected Class<?> loadClass(String name, boolean resolve) throws ClassNotFoundException {
Class<?> clazz = findLoadedClass(name);
if (clazz == null) {
clazz = getParent().loadClass(name);
}
if (resolve) {
resolveClass(clazz);
}
return clazz;
}
}
By prioritizing parent ClassLoaders, you reduce the risk of leaking child ClassLoader instances.
Wrapping Up
Memory leaks due to ClassLoader mishandling can severely hinder Java applications. By applying best practices, such as avoiding static references, managing thread locals, and utilizing weak references, you can significantly mitigate the risk of ClassLoader memory leaks in your applications.
For more in-depth strategies on Java performance tuning and memory management, you might explore the following resources:
- Java Memory Management
- Effective Java (by Joshua Bloch)
Keep learning, and avoid those leaks! Happy coding!