Uncovering Java Integer Caching Mysteries!
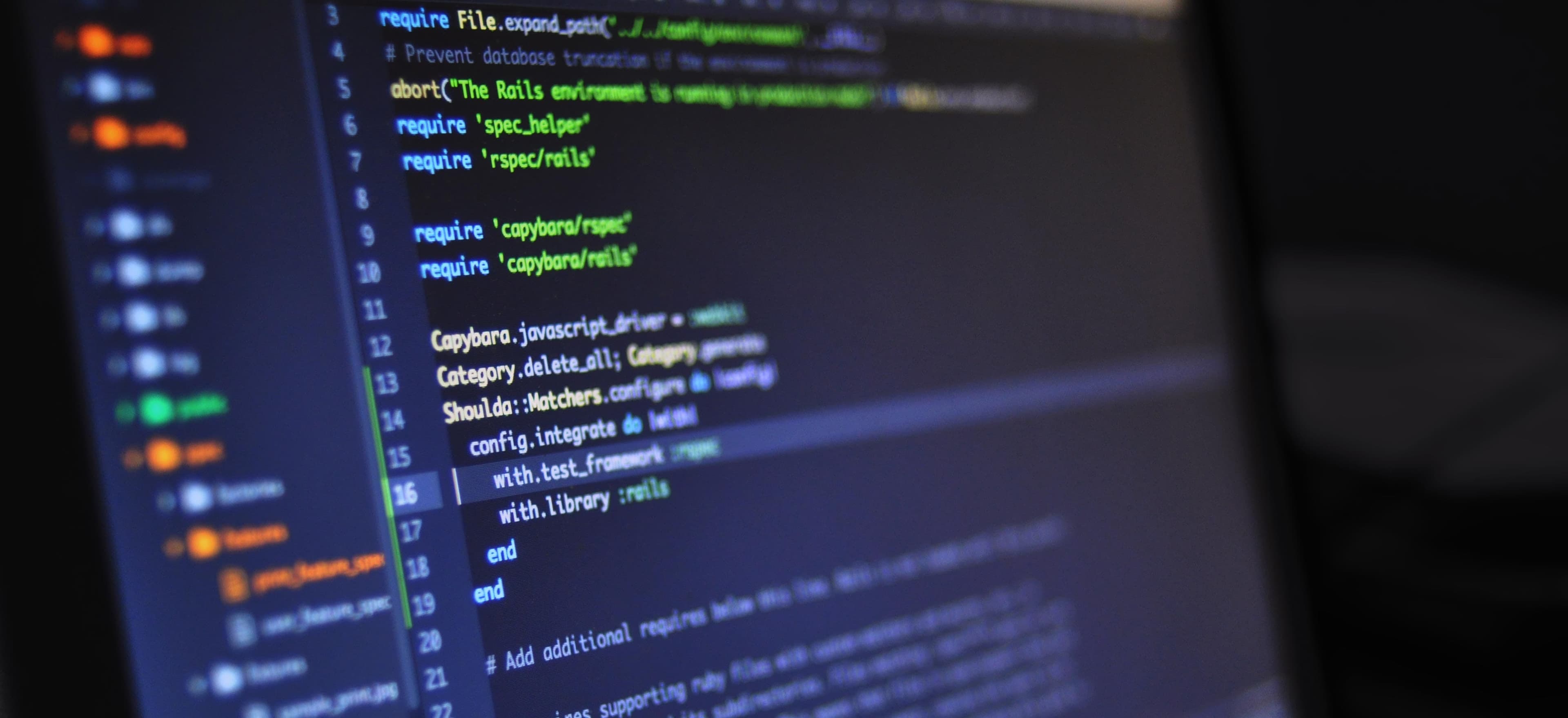
- Published on
Uncovering Java Integer Caching Mysteries!
Java is a powerful programming language, well-known for its portability, performance, and rich API. One intriguing aspect of Java's design is the way it handles integers, particularly through a mechanism known as integer caching. While this may seem like a niche topic, understanding integer caching can dramatically improve performance in your applications and prevent unnecessary object creation. In this blog post, we will dive deep into the mysteries of Java's integer caching, its implementation, and how you can leverage it in your code.
What is Integer Caching?
Integer caching refers to the mechanism by which the Java Virtual Machine (JVM) reuses instances of Integer objects within a certain range, specifically from -128 to 127. This range is frequently used in programming, so caching these objects helps conserve memory and enhance performance.
Why Integer Caching?
- Memory Efficiency: Creating new Integer objects can be resource-intensive. By reusing existing objects, the JVM minimizes memory usage.
- Performance Improvement: Caching reduces the overhead associated with object creation, leading to faster execution of code where integers are frequently used.
How Does Integer Caching Work?
The Integer
class in Java uses caching to maintain a pool of Integer objects. When you create an Integer object that falls within the range of -128 to 127, it doesn’t create a new object. Instead, it returns a reference to an already existing Integer object.
This is facilitated by the Integer.valueOf(int i)
method, which checks if i falls into the cached range. If so, it returns the cached object; otherwise, it creates a new instance.
Example 1: Integer Caching in Action
Let’s take a look at how integer caching works in practice:
public class IntegerCachingDemo {
public static void main(String[] args) {
Integer a = Integer.valueOf(100);
Integer b = Integer.valueOf(100);
System.out.println("a == b : " + (a == b));
}
}
Output:
a == b : true
In this case, both a
and b
reference the same Integer object because 100 falls within the cached range. Thus, the comparison returns true.
Example 2: Out of Cache Range
Now let's see what happens outside of the cached range:
public class IntegerCachingDemo {
public static void main(String[] args) {
Integer a = Integer.valueOf(128);
Integer b = Integer.valueOf(128);
System.out.println("a == b : " + (a == b));
}
}
Output:
a == b : false
For values such as 128, the JVM creates new integer objects. Hence, a
and b
are not the same object, leading to a false comparison.
Custom Caching and Integer Objects
While the built-in integer caching mechanism is useful, there are situations where you might need to create your own caching mechanisms, especially when dealing with larger integer ranges or custom objects.
Example 3: Custom Integer Caching
Below is an example of how you might implement a simple cache for integer objects.
import java.util.HashMap;
import java.util.Map;
public class CustomIntegerCache {
private static final int MAX_CACHE_SIZE = 500;
private static final Map<Integer, Integer> cache = new HashMap<>();
public static Integer valueOf(int i) {
if (cache.containsKey(i)) {
return cache.get(i);
} else {
if (cache.size() < MAX_CACHE_SIZE) {
cache.put(i, i);
}
return i; // In real applications, consider returning a new Integer instance or using another pooling technique
}
}
public static void main(String[] args) {
Integer a = CustomIntegerCache.valueOf(250);
Integer b = CustomIntegerCache.valueOf(250);
System.out.println("a == b : " + (a == b)); // Here it might not return true
}
}
Commentary
In this custom implementation, integers are stored in a HashMap. When a value is requested, the cache is checked. If the value exists, it returns the cached object. If not, the integer is either added to the cache or simply returned if the cache is full.
This is just a basic example to illustrate how caching can be tailored to your needs. In real-world applications, it would be wise to implement a more robust caching mechanism, potentially using third-party libraries such as Ehcache or Caffeine.
Common Pitfalls of Integer Caching
Understanding integer caching can help avoid performance pitfalls. Here are some common mistakes to watch for:
-
Not Realizing the Cache Bounds: Developers often assume integers work like other objects. Be cautious with values outside the cache range.
-
Using the Equality Operator: It's vital to understand the difference between
==
and.equals()
. The former checks for reference equality, while the latter checks for value equality.
Example 4: Equality Comparison Pitfall
public class EqualityComparison {
public static void main(String[] args) {
Integer a = Integer.valueOf(127);
Integer b = 127; // This autoboxes into an Integer object.
System.out.println("a == b : " + (a == b)); // true
System.out.println("a.equals(b) : " + a.equals(b)); // true
}
}
A Final Look
Integer caching in Java is a fundamental concept that, when understood, can lead to more efficient and performant applications. By leveraging the built-in integer caching mechanism, as well as creating your own caching strategies where necessary, you can handle integers more effectively in your Java programs.
If you want to delve deeper into Java performance optimization, consider checking out resources like Java Performance: The Definitive Guide or explore performance-related topics in community-driven platforms like Stack Overflow.
Understanding how integer caching operates is just one piece of the larger Java performance puzzle, and we encourage you to explore beyond integers as you design robust applications. Happy coding!