Unraveling Common Pitfalls in ASM Bytecode Manipulation
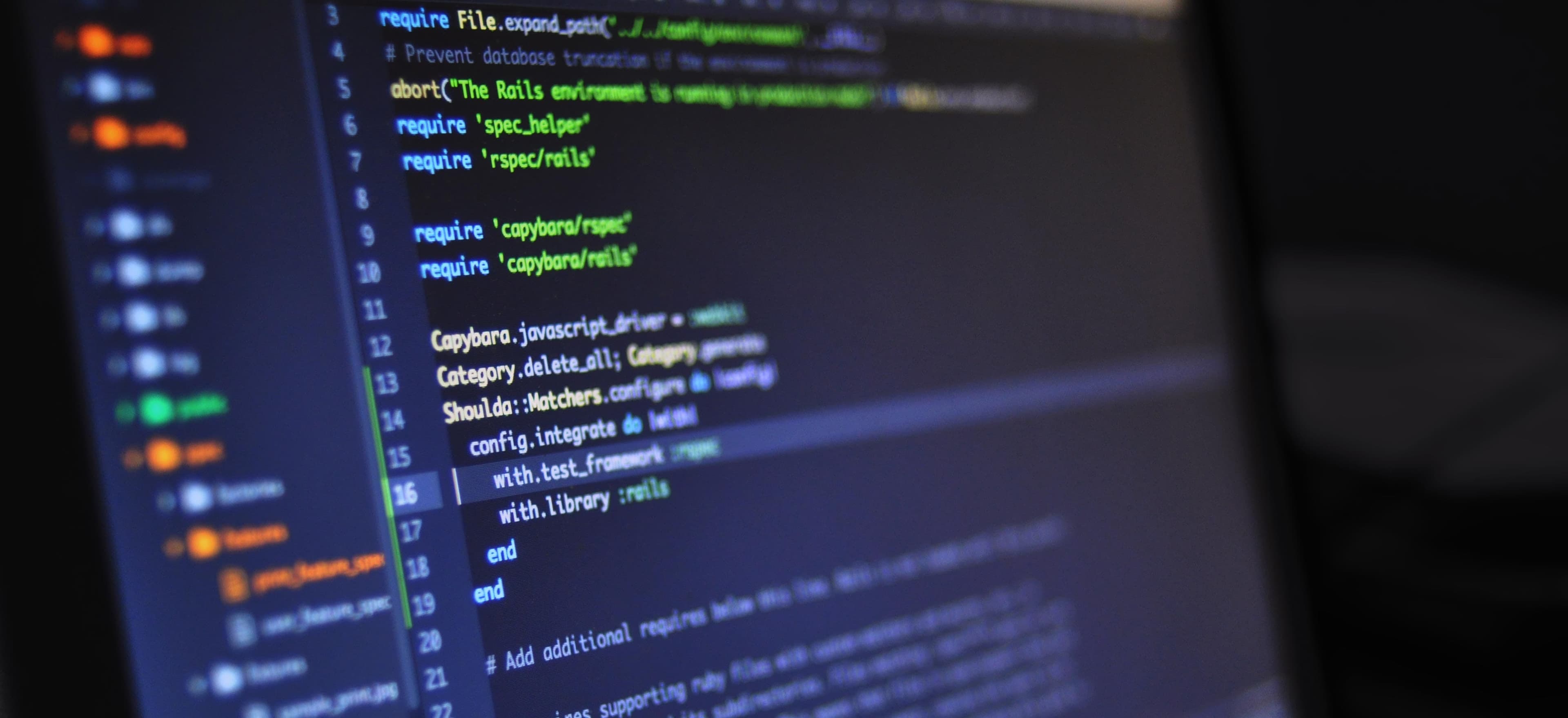
- Published on
Unraveling Common Pitfalls in ASM Bytecode Manipulation
Java developers often find themselves in the world of bytecode manipulation for various reasons: frameworks like AspectJ, libraries like Hibernate, or the need to instrument code dynamically. ASM, a low-level Java library, allows developers to manipulate bytecode efficiently. However, the journey into ASM bytecode manipulation is fraught with pitfalls.
Understanding ASM: The Basics
Before diving into pitfalls, let us establish what ASM is and why it is used. ASM is a Java library that provides functionalities to analyze and transform Java bytecode. This allows developers to perform tasks like code generation, optimization, and instrumentation dynamically.
Key Benefits of Using ASM:
- Lightweight: ASM manipulates bytecode directly and efficiently.
- Highly customizable: Enables tailored manipulation and transformation.
- High Performance: ASM-generated code tends to perform better due to its low-level nature.
For further reading on ASM, check the official ASM documentation.
Common Pitfalls in ASM Bytecode Manipulation
Manipulating bytecode with ASM can be powerful but also risky. Below, we’ll discuss several common pitfalls, their causes, and how to avoid them.
1. Incorrect ClassLoader Usage
Pitfall: One of the first complications that new ASM users encounter is misuse of ClassLoader. In Java, each class is loaded using a ClassLoader, which can be sensitive to the context in which the class is loaded.
Solution: Ensure that the correct ClassLoader is used when manipulating classes. When you create a transformer, explicitly specify the context.
import org.objectweb.asm.ClassVisitor;
import org.objectweb.asm.ClassWriter;
public class MyClassVisitor extends ClassVisitor {
public MyClassVisitor(ClassWriter writer) {
super(Opcodes.ASM9, writer);
}
@Override
public void visitEnd() {
// Finalize class visit, ensuring proper resource handling
super.visitEnd();
}
}
By utilizing ClassWriter
appropriately, you avoid potential issues relating to multiple versions of the same class, ensuring that your transformations apply correctly.
2. Ignoring Exception Handling
Pitfall: When manipulating bytecode, it is easy to overlook exceptions that may arise due to malformed bytecode. A failure to handle exceptions gracefully can lead to application crashes or undesirable behavior.
Solution: Always include robust exception handling in your bytecode manipulation logic. Catch exceptions that might be thrown and provide informative logging.
try {
// Bytecode transformation logic
} catch (Exception e) {
e.printStackTrace(); // Log the error
throw new RuntimeException("Failed to transform bytecode", e);
}
With proper handling, you can make your application more resilient and maintainable.
3. Modifying Final Fields
Pitfall: Final fields in Java are designed to be constants. Trying to modify them through bytecode manipulation can lead to unpredictable results or runtime exceptions.
Solution: Avoid altering final fields unless you are acutely aware of the consequences. Instead, consider alternative designs that allow for better flexibility, such as using accessors or wrapper classes.
public class MyClass {
private final int constantValue;
public MyClass(int value) {
this.constantValue = value;
}
}
If you need to change behavior, wrapping or composition can serve as an alternative without breaking the integrity of final fields.
4. Overlooking Stack Map Frames
Pitfall: Stack map frames are an integral part of bytecode verification. Neglecting to properly define stack map frames can make the modified class unloadable, leading to VerifyError
at runtime.
Solution: Ensure that the stack map frames are updated alongside bytecode changes. You can leverage methods like visitFrame()
to maintain stack integrity.
@Override
public void visitFrame(int type, int numLocal, Object[] local, int numStack, Object[] stack) {
// Define the frame according to the changes made
super.visitFrame(type, numLocal, local, numStack, stack);
}
By handling stack maps properly, you can avoid common verification errors in bytecode.
5. Performance Overhead from Unoptimized Code
Pitfall: Generated bytecode may exhibit performance overhead if not optimized. This often arises from unnecessary method calls or excessive boilerplate code.
Solution: When generating or manipulating bytecode, ensure that you adhere to best practices for performance. This includes minimizing unnecessary method calls and using inline expressions where appropriate.
public void myMethod() {
System.out.println("This is a method");
// Instead of calling a method, consider inlining heavy operations
}
By keeping your bytecode efficient, you can maintain optimal application performance.
6. Failing to Test Changes
Pitfall: One of the more severe pitfalls is neglecting to test bytecode manipulations. Since changes affect the underlying execution, a lack of testing can lead to runtime failures that are hard to trace.
Solution: Implement a robust testing strategy. Utilize automated tests to validate that bytecode changes perform as expected.
@Test
public void testBytecodeTransformation() {
// Execute transformation, then assert expected behavior
MyClass transformedClass = transformBytecode(MyClass.class);
assertEquals("Expected result", transformedClass.someMethod());
}
By prioritizing testing, you safeguard your application against unforeseen problems.
In Conclusion, Here is What Matters
Bytecode manipulation using ASM can empower developers to create optimized and functional applications. However, with great power comes the responsibility to avoid pitfalls. By taking heed of the discussed pitfalls and implementing the recommended solutions, you can navigate the intricate landscape of ASM bytecode manipulation more effectively.
For those looking to dive deeper into ASM, consider checking out resources like ASM: A Java Bytecode Manipulation Framework that provide insights and practical examples.
Further Reading
- Java Bytecode Basics
- The ASM Technical Documentation
Final Words
Embarking on your ASM journey? Remember to prioritize proper usage, error handling, optimization, and robust testing. With these guidelines as your compass, you can harness the full potential of ASM without falling into common traps. Happy coding!