Boosting Java Reflection Performance: Essential Tips
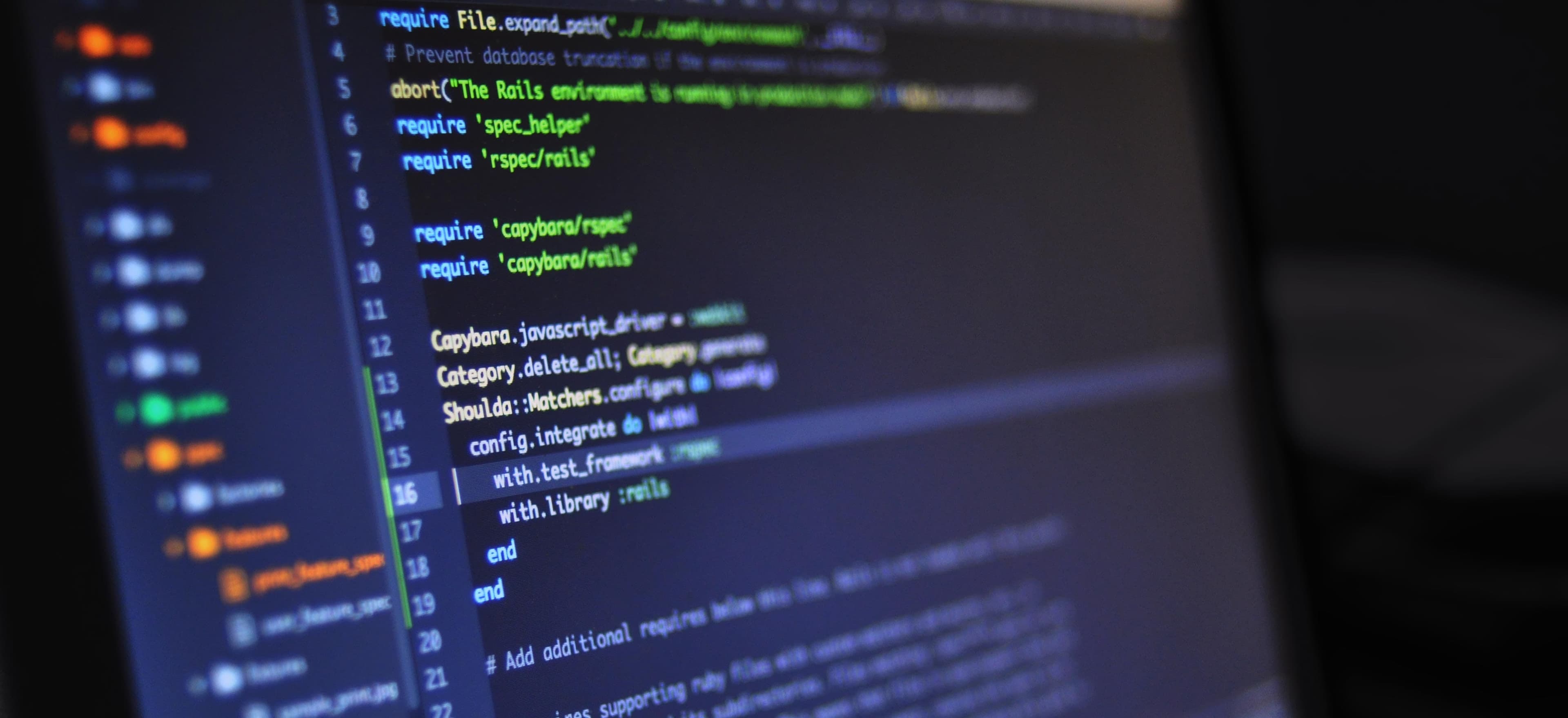
- Published on
Boosting Java Reflection Performance: Essential Tips
Java Reflection is a powerful feature that allows developers to inspect and manipulate classes, methods, fields, and even the entire object structure at runtime. While reflection provides flexibility and dynamism, it can also introduce performance bottlenecks if not handled correctly. In this blog post, we’ll explore essential tips for improving Java Reflection performance, ensuring your applications run smoother and faster.
Understanding Java Reflection
Reflection is part of the Java Programming Language, providing an interface to analyze and operate upon classes, interfaces, fields, and methods at runtime. It’s typically used for:
- Accessing Class Metadata: To understand what a class consists of.
- Dynamically Invoking Methods: To call methods at runtime that are known only at runtime.
- Modification: Changing fields/accessing private data when needed.
While reflection is incredibly useful, it is inherently slower than direct method calls or field access due to the overhead it introduces. This is primarily due to:
- Method Lookup Time: Reflection requires a lookup to determine which method or field you intend to manipulate.
- Security Checks: The Java Runtime Environment (JRE) performs additional security checks to ensure safe access and correct permissions.
- Type Checking Overhead: With reflection, additional checks are needed to ascertain types at runtime.
When to Use Reflection
Before diving into optimization strategies, it's essential to determine when you should use reflection. Some common scenarios include:
- Framework Development: Dependency Injection frameworks often rely on reflection.
- Serialization Libraries: Reflection helps serialize and deserialize objects.
- Testing Libraries: Mocking frameworks utilize reflection to access private methods and fields.
However, it's essential to use reflection judiciously, as excessive use can lead to maintenance challenges and performance degradation.
Essential Tips for Boosting Reflection Performance
To maximize performance while using reflection in Java, consider the following strategies:
1. Cache Reflective Calls
When you make reflective calls for methods or constructors, cache these lookups. Doing so prevents unnecessary repetitive lookups which can significantly degrade performance.
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Map;
public class ReflectionUtil {
private static final Map<String, Method> methodCache = new HashMap<>();
public static Object invokeCachedMethod(Object obj, String methodName, Object... args) throws Exception {
Class<?> cls = obj.getClass();
Method method = methodCache.get(methodName);
if (method == null) {
// If method not in cache, look it up
method = cls.getMethod(methodName, getParameterTypes(args));
methodCache.put(methodName, method);
}
return method.invoke(obj, args);
}
private static Class<?>[] getParameterTypes(Object... args) {
return args == null ? new Class<?>[0] : Arrays.stream(args).map(Object::getClass).toArray(Class<?>[]::new);
}
}
Why Cache? Caching reduces the number of calls to reflection methods, minimizing overhead and improving speed, especially if the same methods are invoked repeatedly.
2. Use Access Control Setters
When accessing fields or methods, the Java Reflection API offers a way to override access checks by calling setAccessible(true)
. Be cautious, as this can have security implications.
import java.lang.reflect.Field;
public class ReflectionExample {
public static void setFieldValue(Object obj, String fieldName, Object value) throws Exception {
Field field = obj.getClass().getDeclaredField(fieldName);
field.setAccessible(true); // Bypass private access control
field.set(obj, value);
}
}
Why Use Access Control Setters? This allows direct access to private fields, reducing the overhead introduced by multiple access checks, hence improving performance.
3. Minimize Reflection Usage
Understanding when not to use reflection is just as critical as optimizing it. If code can achieve the same goal without reflection, opt for that approach. For instance, using interfaces and polymorphism can often replace the need for reflection.
public interface Shape {
double area();
}
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
Consider using design patterns like Strategy or Factory to avoid reflection.
4. Use Method Handles Instead of Reflection
Java 7 introduced the MethodHandle API, which provides a more performance-oriented alternative to reflection. Method handles are arguably faster because they bypass much of the reflection overhead.
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodType;
public class MethodHandleExample {
public static void main(String[] args) throws Throwable {
MethodHandles.Lookup lookup = MethodHandles.lookup();
MethodHandle methodHandle = lookup.findVirtual(Circle.class, "area", MethodType.methodType(double.class));
Circle circle = new Circle(2.0);
double area = (double) methodHandle.invoke(circle);
System.out.println("Area of Circle: " + area);
}
}
Why Use Method Handles? They are typically faster than reflective method invocations due to reduced overhead. They can also be more flexible with dynamic calls.
5. Limit Access to Local Contexts
Limit the usage of reflection to a specified local context where possible. For operations that should not be universal, minimizing the scope limits the performance impact.
A Final Look
Java Reflection can be a double-edged sword—it offers flexibility and powerful capabilities but can lead to performance degradation and complexity if not managed wisely. By implementing the aforementioned strategies—caching method calls, using access control setter wisely, minimizing reflection overall, leveraging method handles, and restricting access—developers can harness the benefits of reflection without succumbing to its pitfalls.
Learn More
To gain deeper insights into Java Reflection and its performance implications, check out these resources:
By keeping these tips in mind, you can work effectively with Java Reflection while maintaining high performance and clean code architecture. Happy coding!