Mastering Deadlock Prevention in Java Concurrent HashMap
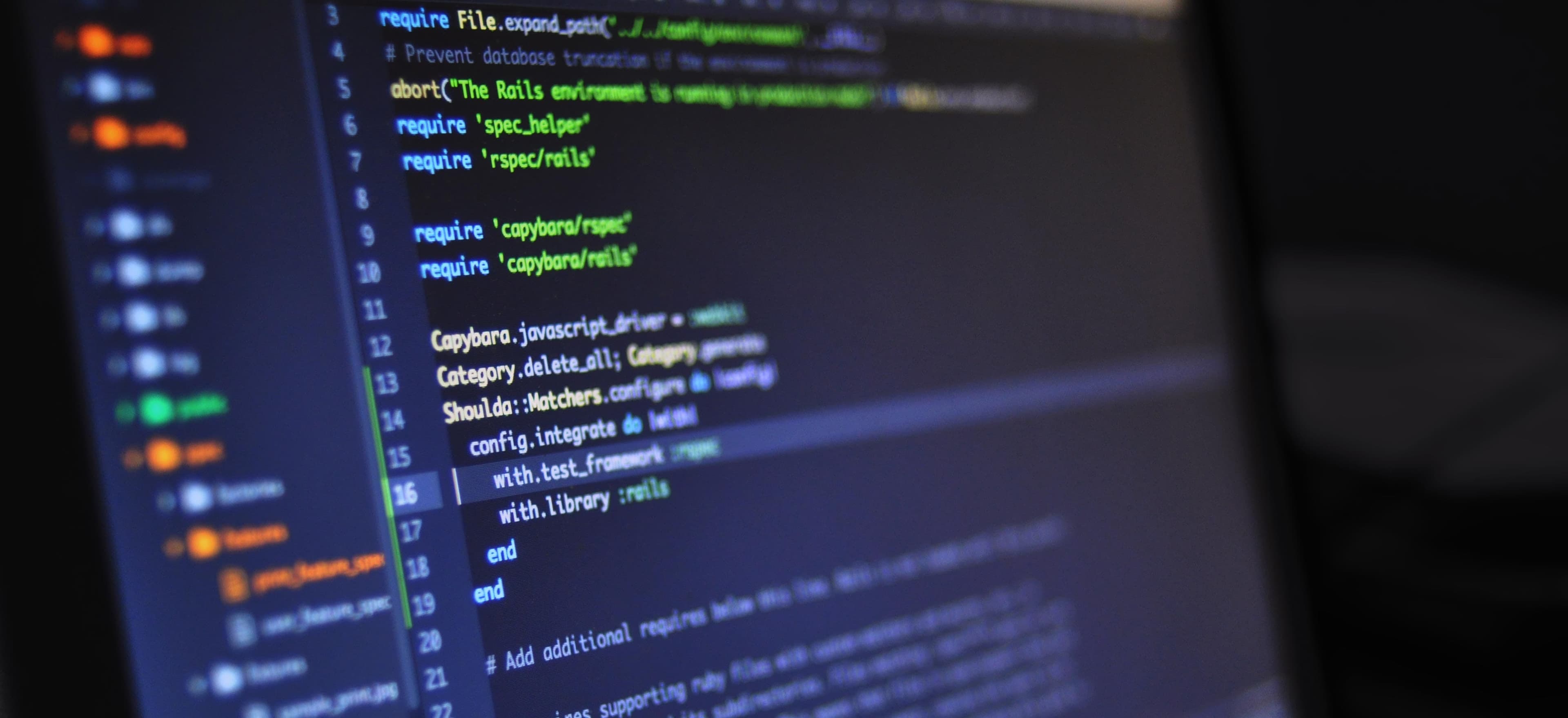
- Published on
Mastering Deadlock Prevention in Java Concurrent HashMap
In the realm of multithreaded programming, ensuring that your applications run smoothly while handling concurrent processes is paramount. One particular challenge faced by developers is deadlock— a state where two or more threads are waiting indefinitely for resources held by each other. In this blog post, we will explore deadlock prevention strategies, focusing on how to effectively manage concurrency in Java's ConcurrentHashMap
.
Understanding Deadlock
Before diving into solutions, let's define what deadlock is. Deadlock occurs when:
- Mutual Exclusion: There is at least one resource being held in a non-shareable mode.
- Hold and Wait: A thread is holding at least one resource while waiting to acquire additional resources.
- No Pre-emption: Resources cannot be forcibly taken from threads holding them.
- Circular Wait: There is a circular chain of threads where each thread is waiting for a resource held by the next thread in the chain.
To break it down simply—threads are stuck, unable to proceed because they wait for each other to release resources. Understanding these principles equips Java developers with the awareness to implement strategies that prevent deadlocks.
The ConcurrentHashMap: An Overview
Java provides various collection classes to manage data concurrently. Among these, the ConcurrentHashMap
stands out as a highly optimized data structure for concurrent access. The main attributes of ConcurrentHashMap
include:
- Thread-safety: It allows concurrent access without requiring extensive synchronization.
- Segment locking: It divides the map into segments and locks only the segment in use, allowing multiple threads to read and write simultaneously.
These features are integral to building efficient and responsive applications.
Here’s how you can declare a ConcurrentHashMap
:
import java.util.concurrent.ConcurrentHashMap;
public class Example {
public static void main(String[] args) {
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
// Adding elements
map.put("A", 1);
map.put("B", 2);
// Accessing elements
System.out.println(map.get("A")); // Output: 1
}
}
Techniques to Prevent Deadlocks
1. Lock Ordering
The simplest way to avoid deadlock is to impose a strict ordering on locks. If all threads acquire locks in a predetermined order, cyclic wait conditions cannot occur. Here’s how you can implement lock ordering:
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class LockOrderingExample {
private final Lock lock1 = new ReentrantLock();
private final Lock lock2 = new ReentrantLock();
public void methodA() {
lock1.lock();
try {
// Ensure that lock2 is acquired in a consistent order
lock2.lock();
try {
// Perform operations
} finally {
lock2.unlock();
}
} finally {
lock1.unlock();
}
}
public void methodB() {
lock1.lock();
try {
lock2.lock();
try {
// Perform operations
} finally {
lock2.unlock();
}
} finally {
lock1.unlock();
}
}
}
In this example, lock1
is always acquired before lock2
in both methods. This consistency prevents circular waits.
2. Timeout Mechanism
If threads cannot acquire locks, you can implement a timeout mechanism. This way, a thread gives up waiting for a lock after a specified duration, preventing potential deadlocks.
Here’s a sample implementation:
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class TimeoutExample {
private final Lock lock1 = new ReentrantLock();
private final Lock lock2 = new ReentrantLock();
public void methodA() {
try {
if (lock1.tryLock() && lock2.tryLock()) {
// Perform operations
}
} finally {
if (lock1.isHeldByCurrentThread()) {
lock1.unlock();
}
if (lock2.isHeldByCurrentThread()) {
lock2.unlock();
}
}
}
}
In this example, tryLock()
attempts to acquire the lock without blocking. If it cannot acquire both locks, the thread can perform other tasks or retry later.
3. Reduce Lock Contention
Reducing the scope and duration of locks can prevent deadlock situations. This minimizes the time for which locks are held:
public void process() {
// Lock only the critical section
synchronized (this) {
// Perform critical operation
}
// Perform non-critical operations outside of the synchronized block
}
This technique reduces contention on locks, as threads wait for shorter periods for resources.
4. Using Read-Write Locks
In scenarios where a resource is frequently read but seldom modified, using a ReadWriteLock
can minimize contention:
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class ReadWriteLockExample {
private final ReentrantReadWriteLock rwLock = new ReentrantReadWriteLock();
public void readOperation() {
rwLock.readLock().lock();
try {
// Perform read operations
} finally {
rwLock.readLock().unlock();
}
}
public void writeOperation() {
rwLock.writeLock().lock();
try {
// Perform write operations
} finally {
rwLock.writeLock().unlock();
}
}
}
In this example, multiple threads can read concurrently, while write operations are exclusive, reducing the chances of deadlock.
Testing for Deadlocks
While prevention strategies are vital, testing your applications for deadlock conditions is equally important. Tools such as Java Thread Dump Analysis or profilers can help diagnose including visualizing the state of threads and locks.
You can perform a thread dump using the following command during runtime:
jstack <pid>
This provides a snapshot of all currently running threads, helping you identify blocked and waiting threads. You can find more on analyzing thread dumps here.
Key Takeaways
By proactively addressing deadlocks through strategies like lock ordering, timeout mechanisms, reducing contention, and utilizing read-write locks, you can significantly enhance the robustness and responsiveness of your Java applications. ConcurrentHashMap
is a powerful tool for multithreaded scenarios, but remember to apply these techniques.
For additional reading on concurrent collections, consider exploring the Java Documentation for in-depth examples and best practices. With the right strategies in place, you can master deadlock prevention in your Java applications, ensuring that they remain efficient and reliable.
Happy coding!