Fixing Java OutOfMemoryError: Tips for Heap Space Management
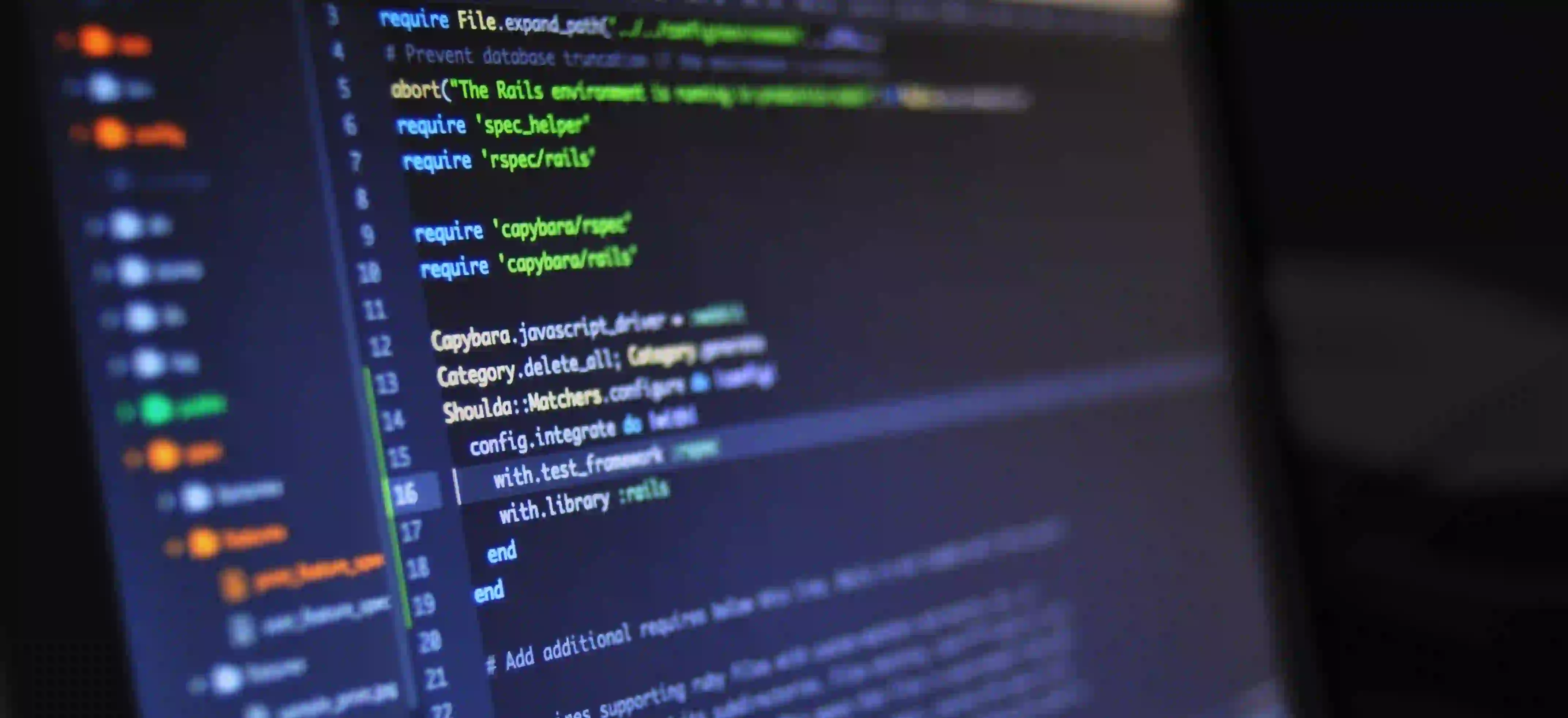
Fixing Java OutOfMemoryError: Tips for Heap Space Management
Java is one of the most popular programming languages in the world, renowned for its platform independence and robust memory management. However, even with its automatic garbage collection, developers still encounter the dreaded OutOfMemoryError
. This occurs when the Java Virtual Machine (JVM) canβt allocate enough memory for an object and there are no more objects eligible for garbage collection. In this blog post, we will dissect the causes of OutOfMemoryError
, understand JVM heap space management, and provide practical tips to prevent these errors from happening in Java applications.
Understanding OutOfMemoryError
Types of OutOfMemoryError
- Java Heap Space: This occurs when the JVM runs out of memory in the heap space allocated to the application.
- GC Overhead Limit Exceeded: When the garbage collector is taking too long to reclaim memory.
- Metaspace: This is related to the memory for class metadata, which can also run out in certain situations.
Why It's Important to Manage Heap Space
Managing your heap space effectively is crucial. Java developers often create applications under the assumption that the JVM will handle all memory management β but this isn't always the case. Tuning the heap size can lead to improved application performance and can significantly reduce the likelihood of running into OutOfMemoryError
.
Heap Space Management
The JVM heap is divided into several areas to manage memory efficiently:
- Young Generation: This is where new objects are allocated. It's smaller and also where most objects die.
- Old Generation: Objects that have survived garbage collection in the Young Generation eventually move here.
- Permanent Generation (or Metaspace in Java 8 and later): Stores metadata about classes and methods.
Understanding how these spaces work is essential for heap management.
Configuring Heap Size
You can specify the heap size when starting your Java application using the following JVM arguments:
java -Xms512m -Xmx4g YourApplication
-Xms
denotes the initial heap size.-Xmx
denotes the maximum heap size.
Why It Matters: Setting the initial heap size to an optimal value can reduce the likelihood of frequent garbage collections at the start. The maximum heap size needs to be set considering the resource availability of your environment.
Example of Repeatedly Triggered OutOfMemoryError
Let's consider a simple example where a developer forgets to release resources.
public class MemoryLeakExample {
private static List<Object> list = new ArrayList<>();
public static void main(String[] args) {
while (true) {
// Creating new Object in a loop
list.add(new Object());
}
}
}
Why It's Dangerous: This code continuously adds new objects to an ArrayList
, which will lead to a java.lang.OutOfMemoryError: Java heap space
since the ArrayList
will keep growing indefinitely.
Identifying Memory Leaks
Using Profiling Tools
Tools like VisualVM and JProfiler can help identify memory leaks. These tools allow you to:
- Monitor memory consumption.
- Take heap dumps when a memory-related issue occurs.
- Analyze the heap dump to see which objects are consuming memory.
Code Quality Analysis
Code analysis tools such as SonarQube help point out potential memory leaks and provide metrics on memory usage. Ensuring code is clean and follows best practices is another way to prevent memory leaks.
Best Practices for Avoiding OutOfMemoryError
1. Properly Release Resources
Always release resources like database connections, file handlers, etc. Utilize try-with-resources to ensure that resources are closed properly:
try (Connection connection = dataSource.getConnection()) {
// Database operations
} catch (SQLException e) {
e.printStackTrace();
}
Why It Helps: This ensures that connections are freed up immediately after usage, reducing the chance of running into memory issues.
2. Use Weak References
In cases where large caches are involved, consider using weak references. Java provides WeakHashMap
which will allow the garbage collector to reclaim objects that are only weakly reachable.
Map<String, WeakReference<MyObject>> cache = new WeakHashMap<>();
Why This Matters: This type of mapping lets the JVM reclaim memory for objects that are not currently in use.
3. Tune Garbage Collection
Understanding the behavior of different garbage collector algorithms in the JVM can lead to better performance. You can switch between different collectors:
java -XX:+UseG1GC -Xms512m -Xmx4g YourApplication
- G1GC: Designed for applications with large heaps, providing predictable response times.
4. Optimize Object Creation
Minimize object creation and avoid retaining references to unnecessary objects. Use object pool patterns where applicable.
public class ConnectionPool {
private static final int POOL_SIZE = 10;
private List<Connection> connections = new ArrayList<>(POOL_SIZE);
public Connection getConnection() {
// Logic to retrieve a connection from the pool.
}
}
Why It Matters: Object pooling reduces overhead associated with creation and destruction of objects, hence minimizing memory footprint.
Closing Remarks
Dealing with OutOfMemoryError
in Java is a common challenge that can be mitigated through thoughtful heap space management, proper coding practices, and efficient resource utilization. By understanding the JVM's memory model and implementing the strategies discussed in this article, you can avoid the pitfalls of memory leaks and ensure your application runs smoothly.
For further reading, you might want to check:
- Oracle's Documentation on Garbage Collection Tuning
- Java Memory Management in Depth
By following the tips and best practices outlined in this blog post, you can significantly enhance your Java application's performance and reliability while avoiding costly downtime due to memory issues.