Mastering Java ClassLoader Troubles: Solutions for App Servers
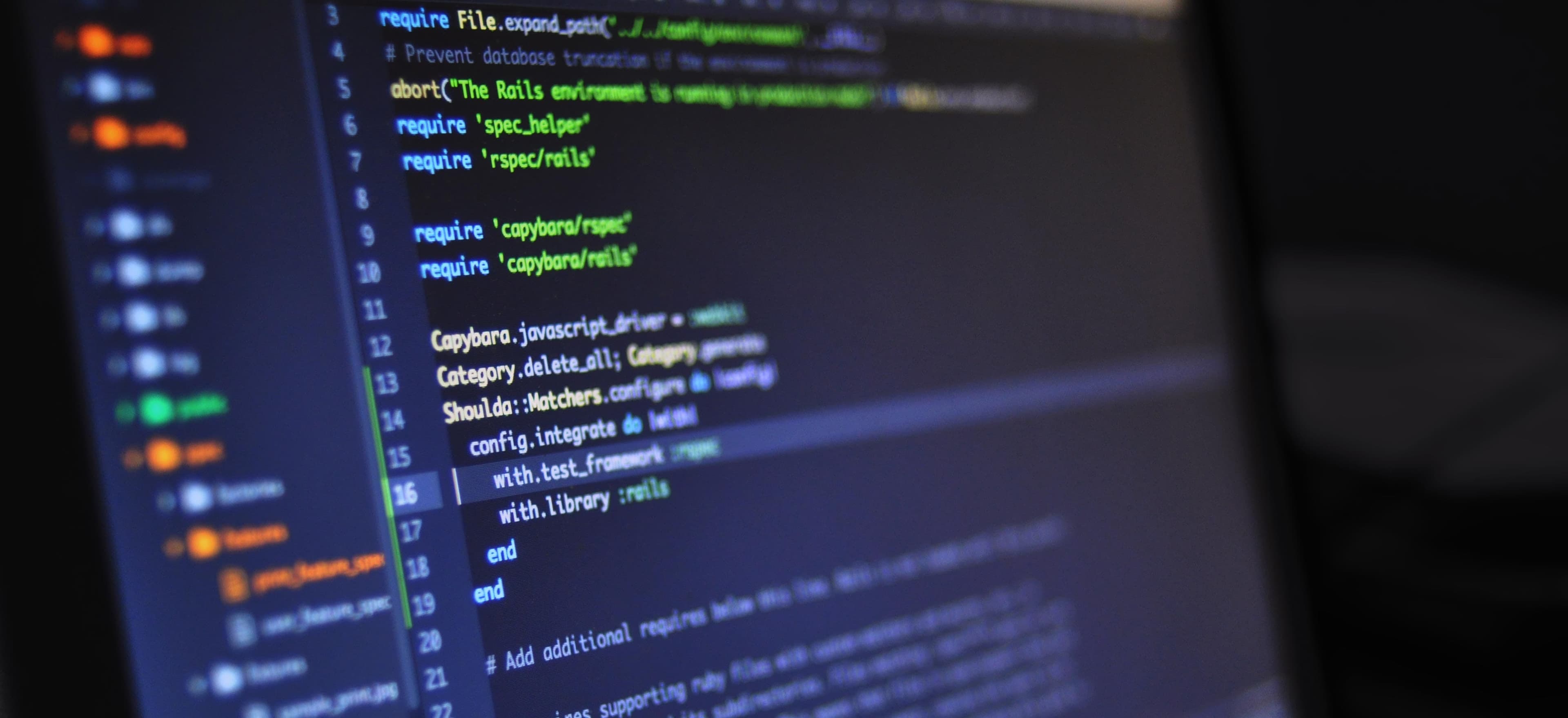
- Published on
Mastering Java ClassLoader Troubles: Solutions for App Servers
Java is a robust programming language widely used for building applications. One of its powerful features is the ClassLoader, which dynamically loads classes into the Java Virtual Machine (JVM) at runtime. However, managing ClassLoaders can be tricky, especially in complex applications like Java EE or web servers. In this post, we will explore common ClassLoader issues, their implications, and provide solutions to effectively manage them.
Understanding Java ClassLoader
A ClassLoader is an integral part of the Java Runtime Environment, responsible for loading classes during program execution. ClassLoaders follow a hierarchical structure to load classes. There are three primary types of ClassLoaders:
- Bootstrap ClassLoader: It loads core Java classes from the Java Runtime Environment (JRE).
- Extension ClassLoader: It loads classes from the extension directory (jre/lib/ext).
- Application ClassLoader: It loads classes from the application classpath.
When a class is needed, the JVM delegates the loading to its ClassLoader hierarchy until the appropriate ClassLoader is found.
Typical ClassLoader Issues
ClassLoader-related issues often arise due to:
- Classpath Conflicts: Multiple versions of the same class in different JAR files lead to conflicts.
- Duplication of Classes: Classes loaded by different ClassLoaders can cause
ClassCastException
. - Parent-Last Loading: Loading classes in a parent-last manner can cause unexpected behavior.
Let’s explore these issues and provide actionable solutions.
1. Classpath Conflicts
Classpath conflicts can occur when you have multiple versions of a library. For example, if your application server includes a version of commons-lang
and your application deploys another, this can lead to conflicts.
Solution: Isolate Dependencies
To avoid conflicts, use tools like Maven or Gradle for dependency management. Define dependencies explicitly to ensure only required versions are loaded.
<!-- Maven Example -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.9</version>
</dependency>
This helps in managing libraries effectively and avoids unwanted classloading issues. By letting the build tool handle dependencies, you reduce the chance of classpath conflicts considerably.
2. Duplication of Classes
When classes are loaded by different ClassLoaders, it can create issues, especially if specific classes need to communicate or extend each other.
Solution: Use a Common Parent ClassLoader
Establish a common classpath for your application to share classes.
// Custom ClassLoader Example
public class MyAppClassLoader extends ClassLoader {
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
// Replace with logic to load classes from a specific jar
byte[] b = loadClassData(name);
return defineClass(name, b, 0, b.length);
}
}
The above code demonstrates a basic structure of a custom ClassLoader. It allows you to control from where classes are loaded. Make sure to dynamically delegate to parent ClassLoaders as needed.
3. Parent-Last Loading
In complex systems, especially when integrating third-party libraries, employing a parent-last ClassLoader can have unintended consequences. The JVM searches for classes in a parent-first order.
Solution: Use a Custom ClassLoader
To make loading classes in a parent-last manner, create a custom ClassLoader.
public class ParentLastClassLoader extends URLClassLoader {
public ParentLastClassLoader(URL[] urls, ClassLoader parent) {
super(urls, null); // set parent to null for parent-last
}
@Override
protected Class<?> loadClass(String name, boolean resolve) throws ClassNotFoundException {
try {
// Attempt to load the class from the child ClassLoader
return findClass(name);
} catch (ClassNotFoundException e) {
// If the class is not found, delegate to parent ClassLoader
return super.loadClass(name, resolve);
}
}
}
In this example, the custom ClassLoader first attempts to load the class using its own resources and falls back to the parent if unsuccessful. This approach allows you to isolate updates or use alternative libraries without unintended side effects.
Best Practices for Managing ClassLoaders
-
Understand Your Environment: Familiarize yourself with how your application server manages ClassLoaders. Each server has its own loading mechanism, which may lead to different behaviors.
-
Optimize ClassPath and Dependencies: Use tools for dependency management to avoid conflicts proactively. Keep your classpath clean and minimal.
-
Leverage JARs Wisely: Instead of bundling numerous JAR dependencies, consider using a single, well-maintained JAR that includes the essential libraries you need.
-
Debug ClassLoader Issues: Utilize tools like Java ClassLoader Viewer to help analyze which classes are loaded from where.
-
Read Documentation: Pay close attention to third-party library documentation regarding ClassLoader behavior, especially in a server context.
A Final Look
ClassLoaders are an integral part of Java's flexibility and power, but they can also be a source of problems, especially in complex applications. By understanding how ClassLoaders work, isolating your dependencies, and leveraging custom ClassLoaders, you can overcome the typical pitfalls associated with ClassLoader management.
For further reading, you may want to check out the official Java Tutorials for deeper insights into ClassLoader implementation and troubleshooting.
With the right approach, you can master ClassLoader issues, providing a solid foundation for your Java applications' architecture and functionality. Happy coding!